This project is just a self-learning experience by implementing in C++14 an algorithm similar to this Udacity project.
Demo on straight road videoclip:
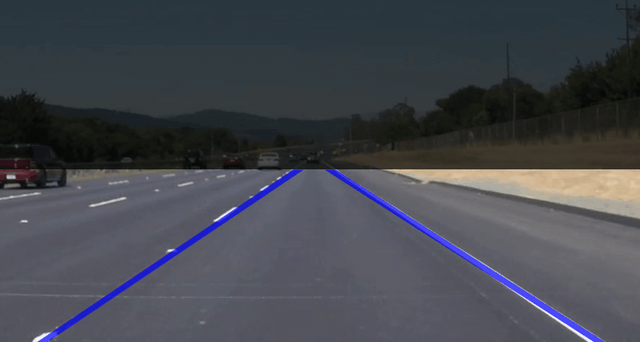
Demo on cornering road videoclip:
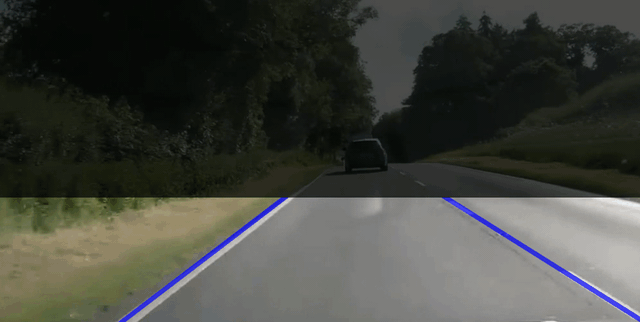
- gcc/clang, cmake
- Install OpenCV
chmod +x ./ci/install_opencv.sh && ./ci/install_opencv.sh
- Install Boost
sudo apt-get install libboost-all-dev
- Clone this repo
git clone https://github.com/huuanhhuynguyen/basic_lane_finding.git
- Make a build directory:
mkdir build && cd build
- Compile:
cmake .. && make
- Run it:
./basic_lane_finding_cpp
- Clone google test
git clone https://github.com/google/googletest tests/googletest/ tests/googletest/
- In the build/ directory, build the test
cmake .. -DBUILD_TEST=ON && make
- Run all tests
./tests/gtest_run
The implementation can be seen in src/main.cpp
with the following steps:
- Read in image as RGB
- Apply Gaussian blur
- Apply a region of interest (ROI)
- Apply Hough line detection
- Transform Hough line to Cartesian line (with slope and bias)
- Filter lines based on slopes
- Separate into left and right lines based on slopes
- On left lines, get the median line
- On right lines, get the median line.
- Apply moving average on each left and right line
- Visualize