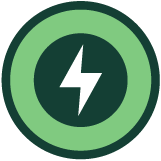
The information in this repository is the result of the FastAPI course given by Platzi.
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. Some of its main features are:
- Fast
- Less errors
- Easy
- Robust
- Standards-based
FastAPI was created by Sebastián Ramírez, and it was developed based on libraries such as Starlette and Pydantic.
- Python fundamentals
- Oriented object programming
- API REST concepts
Suggested resources:
To access the documentation, it is just necessary add /docs
to the URL. For example, if the server is running in http://localhost:8000
, the documentation will be in http://localhost:8000/docs
. It will show a page with all the http methods and the parameters that can be used in the server.
To run an application in this repository, just clone the repository and run the following command:
./docker/start.sh <filename>:<appname>
This script will create a docker container with the necessary dependencies to run the application using the uvicorn
server. The application will be available in http://localhost:5000
.
Note: If you don't pass the app argument, it will use the default name
main:app
.
To run an application in this repository without Docker, just clone the repository and run the following command (make sure to have the dependencies installed):
uvicorn <filename>:<appname>
Note
Flag Description --reload
Automatically reload the server when a change is made. --port
Specify the port to run the server. --host
Specify the host to run the server. Warning: In WSL2, it is necessary to specify the host as
0.0.0.0
to be able to access the server from the browser. This is because the app is running in a virtual machine and to access from another machine, it is necessary to specify the host.
The HTTP methods are used to specify the type of action that will be performed on the resource. The most common are:
Method | Description |
---|---|
GET |
Retrieve a resource. |
POST |
Create a resource. |
PUT |
Update a resource. |
DELETE |
Delete a resource. |
To create a resource, it is necessary to send the data in the body of the request. The data must be in JSON format. For example, to create a user, the following request can be made:
@<appname>.<httpmethod>('<path>')
def <functionname>():
...
return <response>
The parameters can be of different types, such as:
Type | Description |
---|---|
Path |
The parameter is part of the path. |
Query |
The parameter is a query parameter. |
This type of parameter is used to specify the resource that will be retrieved and it will call the server with the url http://localhost:8000/<path>/<parameter1>/<parameter2>
.
@<appname>.<httpmethod>('<path>/{<parameter1>}/{<parameter2>}')
def <functionname>(<parameter1>: <type>, <parameter2>: <type>):
...
return <response>
This type of parameter is used to specify the resource that will be retrieved and it will call the server with the url http://localhost:8000/<path>/?<parameter1>=<value1>&<parameter2>=<value2>
.
@<appname>.<httpmethod>('<path>')
def <functionname>(<parameter1>: <type>, <parameter2>: <type>):
...
return <response>
Warning: The path in the decorator must end with a
/
.
Note:
- The only difference between the
Path
andQuery
parameters is that thePath
parameter must be specified in the decorator and in the function, and theQuery
parameter must just be specified in the function.- If the parameter is optional, it is necessary to specify a default value in the function.
- To send or receive the data in a dictionary, it is necessary import the Body class from
fastapi
and specify it as a default value in each parameter.
Example:
@app.post('/movies') def create_movie(id: int = Body(), title: str = Body(), year: int = Body()): moviedb[id] = {'title': title, 'year': year} return moviedb[id]In this example the request must be sent with the following body:
{ "id": 1, "title": "The Matrix", "year": 1999 }
The schemas are used to specify the data that will be sent or received in the request. It is possible thanks to the Pydantic
library. The schemas are defined as classes that inherit from BaseModel
and the attributes are defined as class attributes. For example, to define a schema for a user, the following code can be used:
from pydantic import BaseModel
class User(BaseModel):
<attribute1>: <type>
<attribute2>: <type>
...
The validation is done automatically by the Pydantic
library. For example, if the attribute name
is defined as a string, the following request will return an error:
{
"name": 123
}
With the Field
class, it is possible to specify the type of data that will be received and the validation that will be done. For example:
<attribute1>: str = Field(..., min_length=3, max_length=50)
<attribute2>: int = Field(..., gt=70, lt=100)
With the Path
and Query
classes, it is possible to specify the type of data that will be received and the validation that will be done. For example:
@<appname>.<httpmethod>('<path>/{<parameter1>}/{<parameter2>}')
def <functionname>(<parameter1>: Path(..., gt=0), <parameter2>: Query(..., gt=0)):
...
return <response>
The responses can be used to specify the data that will be sent in the response. These responses are imported from fastapi
and they are defined as classes that inherit from ResponseModel
. For example, to define a response for a user, the following code can be used:
from fastapi.responses import HTMLResponse, JSONResponse, ...
@<appname>.<httpmethod>('<path>', ..., response_model=<response_class>)
def <functionname>():
...
return <response>
The status codes are used to specify the status of the response. The information about the status codes can be found in the MDN Web Docs. In fastapi
, the status codes are passed as parameters in the decorator. For example:
@<appname>.<httpmethod>('<path>', ..., status_code=<number>)
def <functionname>():
...
return <response>
Note: if fastapi responses are used, the status code can be specified in the response class like this:
return JSONResponse(status_code=<number>, content=<response>) ``
Also, it is possible to get the status code from the status
module of fastapi
.
The token authentication is used to authenticate the user. It is possible to use jwt
or oauth2
in order to authenticate the user.