HollowCodable is a codable customization using property wrappers library for Swift.
- Make Complex Codable Serializate a breeze with declarative annotations!
struct YourModel: HollowCodable {
@Immutable
var id: Int
var title: String?
var url: URL?
@Immutable @BoolCoding
var bar: Bool?
@TrueBoolCoding
var hasDefBool: Bool
@SecondsSince1970DateCoding
var timestamp: Date?
@DateCoding<Hollow.DateFormat.yyyy_mm_dd_hh_mm_ss, Hollow.Since1970.seconds>
var time: Date?
@ISO8601DateCoding
var iso8601: Date?
@HexColorCoding
var color: HollowColor?
@EnumCoding<TextEnumType>
var type: TextEnumType?
enum TextEnumType: String {
case text1 = "text1"
case text2 = "text2"
}
@DecimalNumberCoding
var amount: NSDecimalNumber?
@RGBColorCoding
var background_color: HollowColor?
@AnyBacked<String>
var anyString: String?
@IgnoredKey
var ignorKey: String? = "1234"
var dict: DictAA?
struct DictAA: HollowCodable {
@AnyBacked<Double> var amount: Double?
}
var list: [FruitAA]?
struct FruitAA: HollowCodable {
var fruit: String?
var dream: String?
}
static var codingKeys: [ReplaceKeys] {
return [
ReplaceKeys(location: CodingKeys.color, keys: "hex_color", "hex_color2"),
ReplaceKeys(location: CodingKeys.url, keys: "github"),
]
}
}
/// You can used like:
let datas = ApiResponse<[YourModel]>.deserialize(from: json)?.data
- Like this json:
{
"code": 200,
"message": "test case.",
"data": [{
"id": 2,
"title": "Harbeth Framework",
"github": "https://github.com/yangKJ/Harbeth",
"amount": "23.6",
"hex_color": "#FA6D5B",
"type": "text1",
"timestamp" : 590277534,
"bar": 1,
"hasDefBool": "",
"time": "2024-05-29 23:49:55",
"iso8601": null,
"anyString": 5,
"background_color": {
"red": 255,
"green": 128,
"blue": 128
},
"dict": {
"amount": "52.9",
},
"list": [{
"fruit": "Apple",
"dream": "Day"
}, {
"fruit": "Banana",
"dream": "Night"
}]
}, {
"id": 7,
"title": "Network Framework",
"github": "https://github.com/yangKJ/RxNetworks",
"amount": 120.3,
"hex_color2": "#1AC756",
"type": null,
"timestamp" : 590288534,
"bar": null,
"hasDefBool": null,
"time": "2024-05-29 20:23:46",
"iso8601": "2023-05-23T09:43:38Z",
"anyString": null,
"background_color": null,
"dict": null,
"list": null
}]
}
- @Immutable: Becomes an immutable property.
- @IgnoredKey: Optional Property to not included it when Encoding or Decoding.
- @AnyBackedCoding: Support multiple types when Encoding or Decoding.
- @DefaultBackedCoding: When Decoding fails, the default value will be set.
- @BoolCoding: Bool Encoding or Decoding and can set default value.
- @Base64DataCoding: For a Data property that should be serialized to a Base64 encoded String.
- @DateFormatterCoding: Date property that should be serialized using the customized DataFormat.
- @ISO8601DateCoding: Date property that should be serialized using the ISO8601DateFormatter.
- @Since1970DateCoding: Date property that should be serialized to Since1970.
- @DecimalNumberCoding: Deserialization to a NSDecimalNumber property.
- @EnumCoding: Serialization to enumeration with RawRepresentable.
- @HexColorCoding: UIColor/NSColor property that should be serialized to hex string.
- @RGBAColorCoding: RGBA color Encoding or Decoding.
- @PointCoding: CGPoint Encoding or Decoding.
- @RectCoding: CGRect Encoding or Decoding.
And support customization, you only need to implement the Transformer protocol.
It also supports the attribute wrapper that can set the default value, and you need to implement the HasDefaultValuable protocol.
Booming is a base network library for Swift. Developed for Swift 5, it aims to make use of the latest language features. The framework's ultimate goal is to enable easy networking that makes it easy to write well-maintainable code.
This module is serialize and deserialize the data, Replace HandyJSON.
π· Example of use in conjunction with the network part:
func request(_ count: Int) -> Observable<[CodableModel]> {
CodableAPI.cache(count)
.request(callbackQueue: DispatchQueue(label: "request.codable"))
.deserialized(ApiResponse<[CodableModel]>.self)
.compactMap({ $0.data })
.observe(on: MainScheduler.instance)
.catchAndReturn([])
}
public extension Observable where Element: Any {
@discardableResult func deserialized<T: HollowCodable>(_ type: T.Type) -> Observable<T> {
return self.map { element -> T in
return try T.deserialize(element: element)
}
}
}
CocoaPods is a dependency manager. For usage and installation instructions, visit their website. To integrate using CocoaPods, specify it in your Podfile:
If you wang using Codable:
pod 'HollowCodable'
The general process is almost like this, the Demo is also written in great detail, you can check it out for yourself.π·
Tip: If you find it helpful, please help me with a star. If you have any questions or needs, you can also issue.
Thanks.π
- π· E-mail address: yangkj310@gmail.com π·
- πΈ GitHub address: yangKJ πΈ
Buy me a coffee or support me on GitHub.
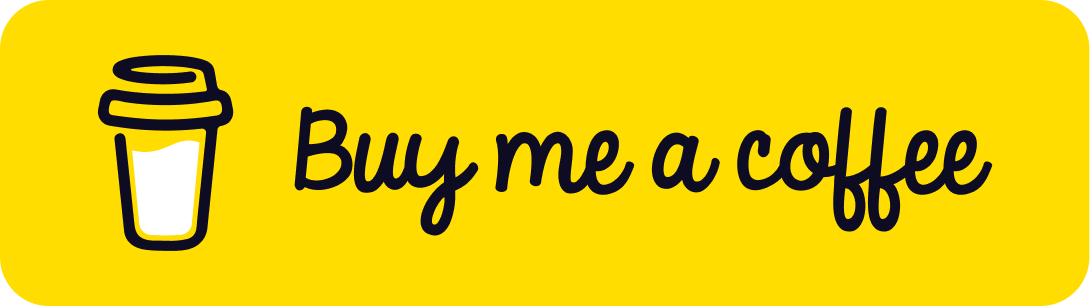
Booming is available under the MIT license. See the LICENSE file for more info.