Stratis is a python-based framework for developing and testing strategies, inspired by the simplicity of tradingview's Pinescript. Currently, stratis is in the early stages of development, and is not yet ready for production use. However, it is encouraged to try it out and provide feedback via GitHub issues.
Stratis is a part of Shenandoah Research's Open source Trading Software Initiative and developed by Polyad Decision Sciences software engineers!
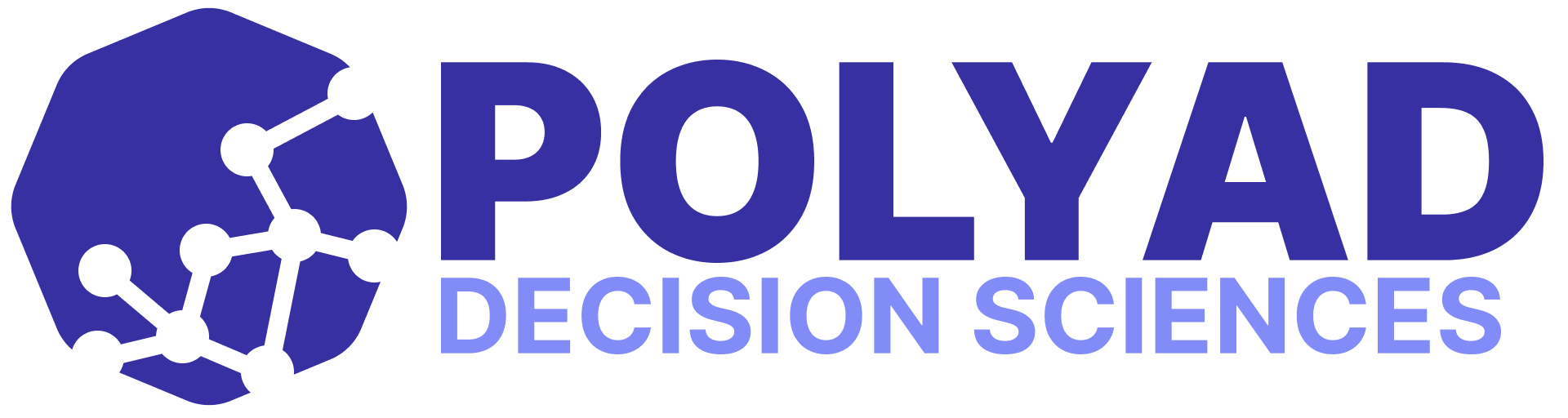
The following code demonstrates how to create a strategy that prints the timestamp and close price of the
OHLC data every hour. The on_step
decorator is used to run the function on every step of the OHLC data. You can find
more info about how to create strategies here.
Using the Strategy
class, along with the on_step
decorator, you can create strategies that are as simple or as complex as you want, with
the full power of python at your disposal.
class OHLCDemo(Strategy):
@on_step # on_step decorated, runs every "step" of the OHLC data
def print_ohlc(self):
# shorthands for the OHLC data
timestamp = self.data.timestamp
close = self.data.close
# if the timestamp is a multiple of 3600000 (1 hour)
if timestamp % 3600000 == 0:
# create a datetime object from the timestamp
dt = datetime.datetime.fromtimestamp(timestamp / 1000)
if dt.hour == 10:
print(f'{dt}: {close}')
data = CSVAdapter('data/AAPL.csv')
strategy = OHLCDemo().run(data)
It is heavily recommended to use Docker to run stratis. This is because stratis requires a number of dependencies that can be difficult to install without Docker.
To install Docker, follow the instructions here.
Once Docker is installed, you can run stratis by running the following commands:
# Clone the repository
git clone https://github.com/robswc/stratis
# Change directory to the repository
cd stratis
# Run the docker-compose file
docker-compose up -d # -d runs the containers in the background
The Stratis UI interface should now be accessible via:
And the Stratis backend (core) should be accessible via:
For more advanced usage, you can run app with python directly, as it is a FastAPI app under the hood. Please note, this may or may not work for Windows and MacOS, as I have only tested it on Linux.
I would recommend using a virtual environment for this. You will also have to install the requirements. The following commands will start the backend of stratis.
cd app # change directory to the app folder
python python -m uvicorn main:app --reload # reloads the app on file changes (useful for development)
The frontend of stratis is a NextJS app. The repository for the frontend can be found here.