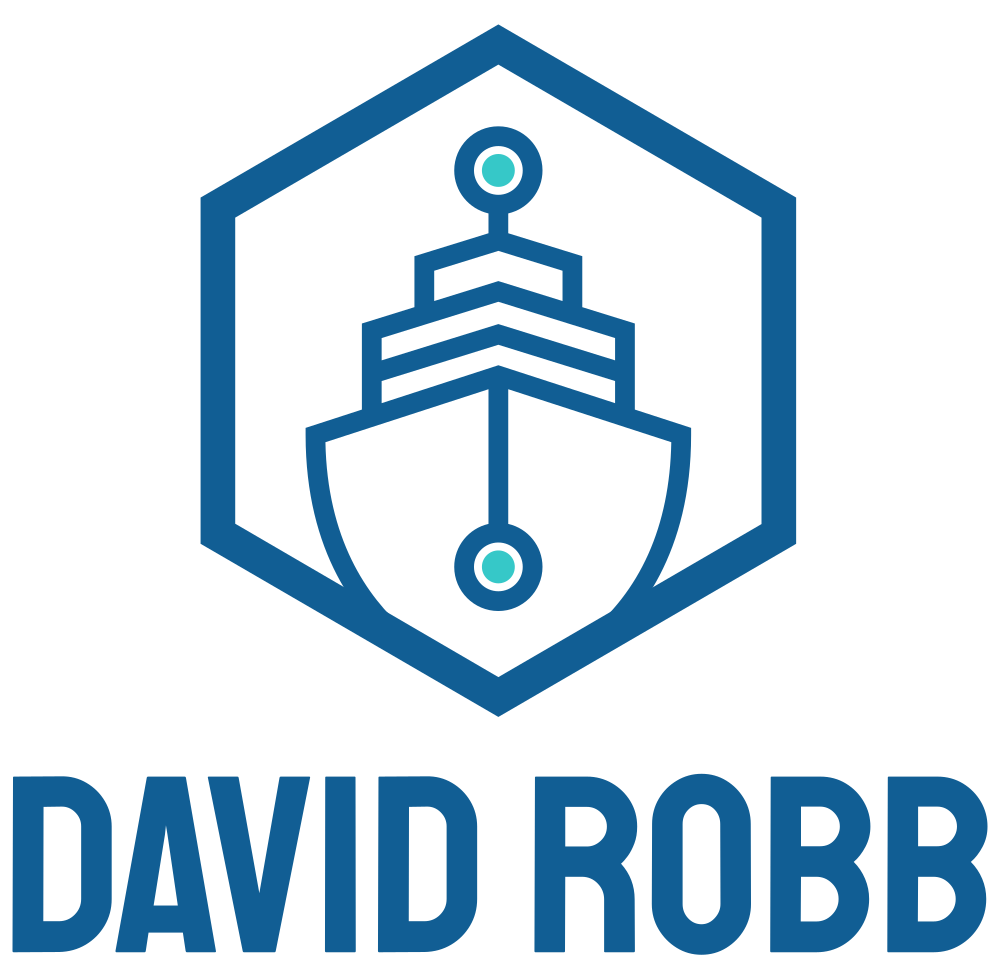
This is a basic template to integrate tailwindcss with Django instead of using the tailwind cdn, which is not recommended for a production deployment.
Explore the docs »
View Demo
·
Report Bug
·
Request Feature
Table of Contents
This is a self contained template for Django 4.2.2, and Tailwindcss 3.3.2. It also includes additional packages I use with every Django project (flake8, black, ruff, and django-extensions).
You will need to have node.js installed on your system to build this project. Go to npmjs to download and install the latest node.js version. This project was created with node v19.6.0 You will also need Python installed on your system. Go to python to download and install the latest python version. This project was created with python v3.11.1.
There are two ways to get started with this project:
- Build by following the instructions
- Build by cloning this repo
You can build this project yourself by doing the following steps:
-
Make sure you have Python and Node.js installed on your system.
-
Create a top-level folder to hold this project.
-
Change into the folder you created and start with creating a python virtual environment.
- python3 -m venv venv, you can use any name for the virtual environment, I always use venv.
-
Activate the virtual environment using:
source venv/bin/activate or venv\Scripts\Activate
-
Run the following command to install Django and the supporting modules:
pip install Django django-extensions flake8 flake8-django black ruff
-
Create a new Django project:
django-admin startproject <your_project_name>
-
Now create a new Django application:
python manage.py startapp core
-
Go into your project's settings.py and make the following changes to INSTALLED_APPS:
... # Applications 'core.apps.CoreConfig', # Modules 'django_extensions',
-
Still in settings.py, go down to TEMPLATES and add the path to the templates folder:
'DIRS': [BASE_DIR / 'templates'],
-
Go down to the static files section of settings.py and add the following lines below the STATIC_URL entry:
STATICFILES_DIRS = [BASE_DIR / 'static'] STATIC_ROOT = BASE_DIR.parent / 'cdn' / 'static'
-
In the django project folder create a static folder, and a templates folder.
-
under the static folder I typically create these folders:
- assets - holds static images and the favicon
- css - holds the css file for the project
- js - holds the javascript file for the project
- src - holds the main.css file for tailwindcss
-
Copy your favicon.ico file the the assets folder (can be a png, or svg - your choice)
-
Create a file named custom.min.css in the css folder (or whatever name you prefer)
-
Create a file named main.js in the js folder (or whatever name you prefer)
-
In the src folder create a file named main.css, or use any name you want. Put the following content in this file:
@tailwind base; @tailwind components; @tailwind utilities;
-
Go back up to the parent folder of the project and create the folder cdn, and inside that a static folder.
-
Back in the django project open the urls.py file, and add the following to configure static files:
from django.conf import settings ... # Add below urlpatterns if settings.DEBUG: from django.conf.urls.static import static urlpatterns += static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
-
Under templates create a base.html file, add the basic html boilerplate to the file and don't forget to put {% load static %} at the top of the file.
-
Add links for favicon, css, and javascript using the static tag to denote the location of the files
-
In the core application open views.py and create the following function:
def index(request): context = {} return render(request, 'core/index.html', context)
-
In the templates folder create a new directory named core, and inside the core folder create index.html. Add the following code to this file.
{% extends 'base.html' %} {% block content %} <div class="flex flex-col justify-center items-center h-screen bg-rose-700"> <h1 class="text-6xl font-bold text-white">Django + Tailwindcss Template</h1> <p class="text-xl">Your text here...</p> </div> {% endblock content %}
{% endblock content %} 23. In the core folder create a new file named urls.py, and add the following code:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
- In the project urls.py file add the following code:
from django.urls import include, ...
urlpatterns = [
...
path('', include('core.urls')),
]
- At this point you can either run the server (python manage.py runserver) or you can run the initial migrate (python manage.py migrate).
- In the root folder of the project run the following command to install tailwindcss:
npm install tailwindcss postcss postcss-cli autoprefixer cssnano
- This will create a new folder named node_modules, and two files package.json, and package-lock.json
- At the command line run the following command:
npx tailwindcss init
-
This will create a new file named tailwind.config.js
-
Inside tailwind.config.js, add the following code to the components section:
"./dj_tw_template/**/*.{html,js}"
-
Manually create a postcss.config.js file, and add the following code:
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
cssnano: {},
},
}
-
Go to packages.json and add a scripts section so you can run postcss and compile the tailwindcss output css file:
"scripts": { "dev": "postcss ./dj_tw_template/static/src/main.css -o ./dj_tw_template/static/css/custom.min.css --watch", "build": "postcss ./dj_tw_template/static/src/main.css -o ./dj_tw_template/static/css/custom.min.css" },
-
To execute the scripts, use either
npm run dev
ornpm run build
-
You can now customize the tailwind code in either base.html or in index.html. For pre-coded examples for a navbar, footer, table or card, go to flowbite.com for some good inspiration.
If you don't want to do all the work yourself, you are free to clone this repository and start customizing this code for your own project.
git clone https://github.com/drobb/django-tailwind-template.git
In the dj_tw-template folder don't forget to create and activate a virtual environment. Once that is done run pip install -r requirements.txt
In the root of the cloned repository run the command npm install
, this will download all the necessary packages to run the project.
You should be able to run both the django project, and the tailwind dev or build script (in separate terminal windows) and play with the project.
Happy coding!
This is intended as starter code for tailwindcss, and not necessarily for Django (although it could be used as Django starter code). I have left this basic enough that you can change any of the names and still end up with a functioning tailwindcss+Django project.
For more examples, please refer to the Documentation
See the open issues for a full list of proposed features (and known issues).
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement". Don't forget to give the project a star! Thanks again!
- Fork the Project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
Distributed under the MIT License. See LICENSE.txt
for more information.
Your Name - @DavidRobb2 - drobb2011@gmail.com
Project Link: https://github.com/drobb2020/django-tailwind-template