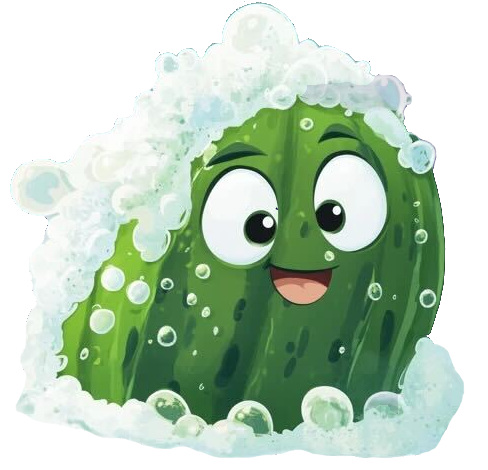
Always clean your Gherkin!
A modern and extensible linter for Gherkin syntax, supporting custom rules, automatic fixing et al. Powered by TypeScript and ESM.
- Installation
- Usage
- Configuration
- Disabling Rules
- Automatic Fixing
- Custom Rules
- Reporting
- Contributing
- Testing
Gherklin can be installed either via NPM or Yarn
npm install gherklin
yarn add gherklin
Gherklin includes a bin file, which can be ran with the following
npx tsx ./node_modules/.bin/gherklin
Gherklin also has an API that can be invoked. A simple example of which is:
import { Runner } from 'gherklin'
;(async () => {
const runner = new Runner()
const init = await runner.init()
if (!init.success) {
throw init.schemaErrors
}
const result = await runner.run()
if (!result.success) {
process.exit(1)
}
process.exit(0)
})()
Gherklin will look for a gherklin.config.ts
file in the same directory as your package.json
file.
This file should default export an object, which contains the parameters for Gherklin.
export default {
featureDirectory: './features',
customRulesDirectory: './custom-rules',
rules: {
'allowed-tags': 'off',
},
}
Parameter | Type | Description |
---|---|---|
featureDirectory (required) |
string |
The folder where your Gherkin features are |
customRulesDirectory |
string | The directory where your custom rules are |
rules |
object |
Configuration per rule |
rules
contains the configuration for each rule, whether built in or custom.
Check rules for a list of built in rules.
Gherklin rules can be disabled on a per line or per file basis.
Adding gherklin-disable
at the top of a file in a comment will disable Gherklin rules for that
file.
# gherklin-disable
Feature: All disabled
Scenario: Nothing here will be linted
Adding gherklin-disable-next-line
will disable Gherklin for the next line.
Feature: The below tag is invalid
# gherklin-disable-next-line
@invalid-tag
Scenario: The above tag is invalid
While gherklin-disable
works for every rule, gherklin-disable-next-line
only works for rules
where it makes sense.
For example, using gherklin-disable-next-line
does not make sense for the no-empty-file
rule.
You can also disable specific rules for the file, using the # gherklin-disable rule-name.
# gherklin-disable allowed-tags, no-unnamed-scenario
Feature: The below tag is invalid
@invalid-tag
Scenario:
Gherklin supports automatic fixing for a small set of rules, usually those to do with whitespace.
To allow Gherklin to automatically fix rules that can be fixed, pass the fix
option to your gherklin.config.ts
file:
export default {
...
fix: true,
}
Gherklin fixes files before the rules are ran against the file. This is so you can be sure that the fix actually worked.
In order to support custom rule fixes, your rule class needs to have a fix
method.
This method takes the Document as a parameter.
This method must call document.regenerate()
to save the document.
An example can be found with the no-trailing-spaces rule.
Custom rules can be loaded by setting the customRulesDirectory
parameter in your config file.
There are a few things needed to consider for rules:
- Each file in the directory contains one rule.
- Each file exports a default class that implements Rule
Schema for rules uses Zod. There are a few different schemas specified in the schemas file, but if you want to create something different, you can use Zod directly. When the rule is loaded, this schema is used to verify the configuration value for the rule.
Gherklin comes with a generator script that you can use. Running the following will generate the skeleton for a new Rule class:
npx tsx ./node_modules/.bin/gherklin-rule
By default, Gherklin outputs to STDOUT. You can change this by specifying the reporter in your
gherklin.config.ts
file or inline configuration. If no reporter is specified, the STDOUT Reporter will be used.
Currently, the available reporters are STDOUT, HTML and JSON.
key | description |
---|---|
type | The type of reporter (html, json, stdout) |
outFile | The file the report is written to |
If you are adding new rules, they must follow the same format as existing rules ( see Custom Rules). Each new rule must have acceptance tests (see testing) and be added to the Rules README.
Tests use the Mocha Test Framework and live in the tests directory.
Tests can be ran with npm test
.