Rapi
We made retrofit more understandable !! RAPI
Explore the docs »
View Demo
·
Report Bug
·
Request Feature
Table of Contents
About The Project
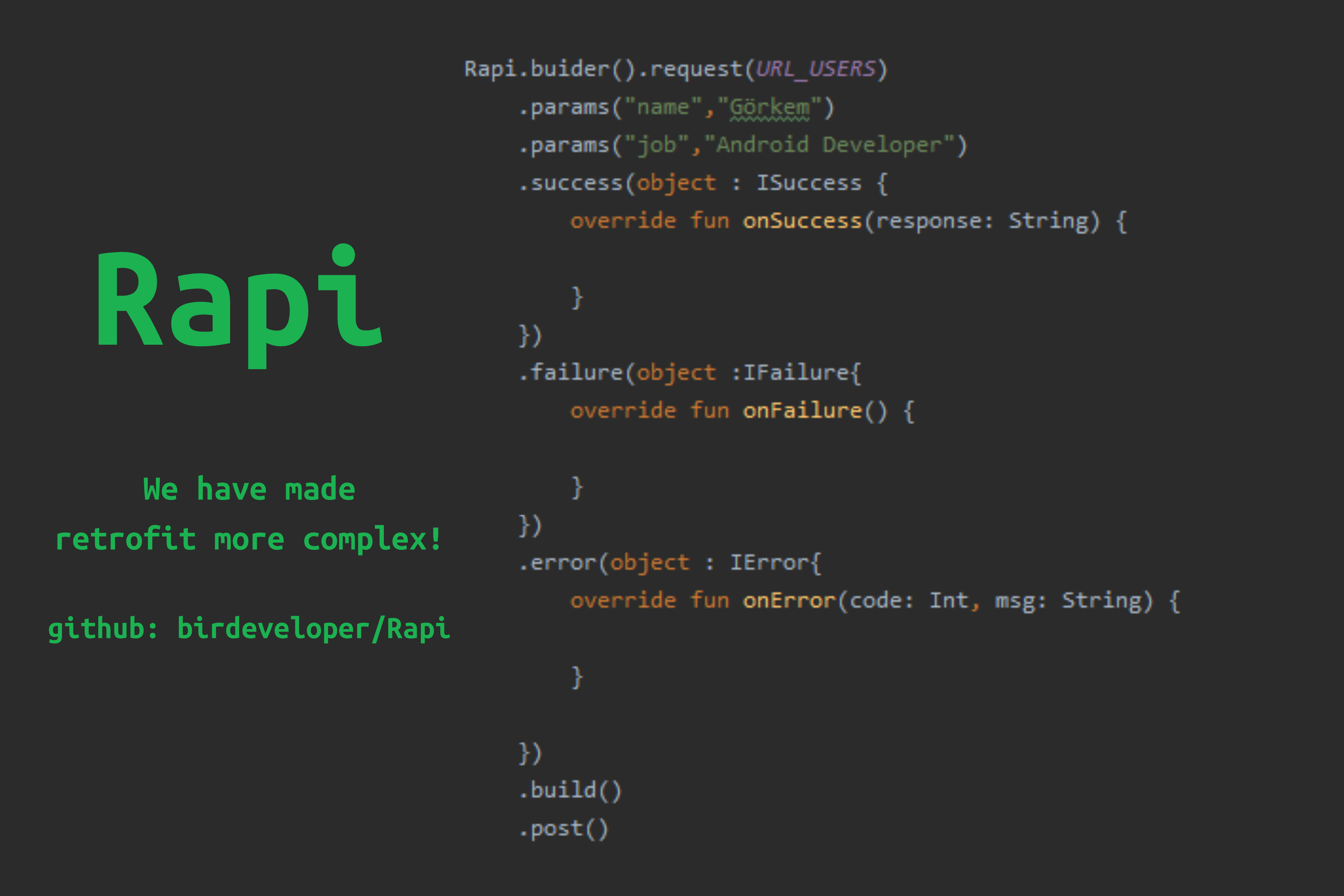
Rapi offers you a lot of innovations by shortening the steps that retrofit should take in a very short and concise way. It organizes the network requests in the background and easily adapts to the MVVM structure.
Built With
Getting Started
To get a local copy up and running follow these simple steps.
Prerequisites
This is an example of how to list things you need to use the software and how to install them. (already installed in the library)
- retrofit
implementation 'com.squareup.retrofit2:retrofit:2.6.3'
Installation
To get a Git project into your build: Step 1. Add the JitPack repository to your build file. Add it in your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Step 2. Add the dependency
dependencies {
implementation 'com.github.birdeveloper:Rapi:1.0.0'
}
Usage
Setup
val headers: WeakHashMap<String, String> = WeakHashMap()
headers["Authorization"] = "Bearer vF9dft4qmTFDfd27asWEgf"
Rapi.init(this,Constants.BASE_URL, headers, 120)
// context, base url, headers or null, timeout
GET Request
Rapi.buider()
.request("https://reqres.in/api/users/")
.params("page",2)
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.get()
POST Request
Rapi.buider()
.request("https://reqres.in/api/users/")
.params("name","Görkem")
.params("job","Android Developer")
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.post()
POST RAW Request
val jsonStr = "{\"name\":\"Görkem\",\"job\":\"Android Developer\"}"
Rapi.buider()
.request(URL_USERS)
.raw(jsonStr)
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.post()
PUT Request
Rapi.buider()
.request(Constants.URL_USER_ID)
.params("name","Resul")
.params("job","Software Developer")
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.put()
DELETE Request
Rapi.buider()
.request(Constants.URL_USER_ID)
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.delete()
DOWNLOAD Request
/*
IMPORTANT!!! Do not forget to write and read permission before discarding this request !!
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
There are codes related to the permissions in the sample file.
*/
Rapi.buider()
.request(Constants.URL_DOWNLOAD)
.dir(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS).absolutePath)
.extension("doc")
.name("example.doc")
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.download()
UPLOAD Request
/*
IMPORTANT!!! Do not forget to write and read permission before discarding this request !!
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
There are codes related to the permissions in the sample file.
*/
Rapi.buider()
.request(Constants.URL_UPLOAD)
.file(File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS).absolutePath+"/example.jpeg"))
.onRequest(object : IRequest{
override fun onRequestStart() {
Log.d("onRequestStart", "run")
}
override fun onRequestEnd() {
Log.d("onRequestEnd", "finish")
}
})
.success(object : ISuccess {
override fun onSuccess(response: String) {
Log.d("onSuccess", response)
}
})
.failure(object :IFailure{
override fun onFailure() {
Log.d("onFailure", "yes")
}
})
.error(object : IError{
override fun onError(code: Int, msg: String) {
Log.d("onError", "code: $code ----- msg: $msg")
}
})
.build()
.upload()
Roadmap
See the open issues for a list of proposed features (and known issues).
Contributing
Contributions are what make the open source community such an amazing place to be learn, inspire, and create. Any contributions you make are greatly appreciated.
- Fork the Project
- Create your Feature Branch (
git checkout -b birdeveloper/Rapi
) - Commit your Changes (
git commit -m 'Add some Rapi'
) - Push to the Branch (
git push origin birdeveloper/Rapi
) - Open a Pull Request
License
Distributed under the MIT License. See LICENSE
for more information.
Contact
Görkem KARA - @gorkemkara - email
Project Link: https://github.com/birdeveloper/rapi