- can it receive user input
- plotly can (basically) replicate Tableau
-
If you want to install plotly just run
!pip install plotly
in your jupyter notebook ORpip install plotly
in your terminal
-
Load in data
-
Make plotly visualizations
-
Show how plotly can be used in dash apps
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv("data/covid19.csv", encoding='ISO-8859-1')
df['dateRep'] = pd.to_datetime(df["dateRep"])
df.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 2020-03-27 | 27 | 3 | 2020 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
1 | 2020-03-26 | 26 | 3 | 2020 | 33 | 0 | Afghanistan | AF | AFG | 37172386.0 |
2 | 2020-03-25 | 25 | 3 | 2020 | 2 | 0 | Afghanistan | AF | AFG | 37172386.0 |
3 | 2020-03-24 | 24 | 3 | 2020 | 6 | 1 | Afghanistan | AF | AFG | 37172386.0 |
4 | 2020-03-23 | 23 | 3 | 2020 | 10 | 0 | Afghanistan | AF | AFG | 37172386.0 |
df.sort_values(by="dateRep", inplace=True)
df.reset_index(inplace=True, drop=True)
df.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Singapore | SG | SGP | 5638676.0 |
1 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Greece | EL | GRC | 10727668.0 |
2 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Lithuania | LT | LTU | 2789533.0 |
3 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Canada | CA | CAN | 37058856.0 |
4 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Brazil | BR | BRA | 209469333.0 |
df.tail()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
7120 | 2020-12-03 | 12 | 3 | 2020 | 15 | 1 | Indonesia | ID | IDN | 267663435.0 |
7121 | 2020-12-03 | 12 | 3 | 2020 | 1 | 0 | Cambodia | KH | KHM | 16249798.0 |
7122 | 2020-12-03 | 12 | 3 | 2020 | 0 | 0 | Algeria | DZ | DZA | 42228429.0 |
7123 | 2020-12-03 | 12 | 3 | 2020 | 15 | 0 | Iceland | IS | ISL | 353574.0 |
7124 | 2020-12-03 | 12 | 3 | 2020 | 15 | 0 | Russia | RU | RUS | 144478050.0 |
# in pandas with matplotlib
df_Afg = df.loc[df["countriesAndTerritories"]=="Afghanistan", ["dateRep", "cases"]]
df_Afg.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | cases | |
---|---|---|
20 | 2019-12-31 | 0 |
131 | 2020-01-01 | 0 |
190 | 2020-01-02 | 0 |
261 | 2020-01-03 | 0 |
271 | 2020-01-13 | 0 |
df_Afg.set_index("dateRep").plot()
<matplotlib.axes._subplots.AxesSubplot at 0x12e11a518>
import plotly.graph_objects as go
fig = go.Figure()
df_Afg = df.loc[df["geoId"]=="AF"]
trace = go.Scatter(x=df_Afg["dateRep"],
y=df_Afg["cases"],
mode="lines+markers",
name="AF")
fig.add_trace(trace)
<div id="c46404bc-4830-451f-a1c3-3c9aa4c7e0aa" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("c46404bc-4830-451f-a1c3-3c9aa4c7e0aa")) {
Plotly.newPlot(
'c46404bc-4830-451f-a1c3-3c9aa4c7e0aa',
[{"mode": "lines+markers", "name": "AF", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00"], "y": [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 3, 6, 5, 1, 0, 0, 2, 0, 10, 6, 2, 33, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 3, 0, 0]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('c46404bc-4830-451f-a1c3-3c9aa4c7e0aa'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
usa_border_buddies = ["United_States_of_America", "Mexico", "Canada"]
fig = go.Figure()
df_USA = df.loc[df["countriesAndTerritories"]=="United_States_of_America"]
trace1 = go.Scatter(x=df_USA["dateRep"], y=df_USA["cases"], mode="lines+markers", name="US")
fig.add_trace(trace1)
df_MEXICO = df.loc[df["countriesAndTerritories"]=="Mexico"]
trace2 = go.Scatter(x=df_MEXICO["dateRep"], y=df_MEXICO["cases"], mode="lines+markers", name="Mexico")
fig.add_trace(trace2)
df_CANADA = df.loc[df["countriesAndTerritories"]=="Canada"]
trace3 = go.Scatter(x=df_CANADA["dateRep"], y=df_CANADA["cases"], mode="lines+markers", name="Canada")
fig.add_trace(trace3)
fig.show()
<div id="8971ca36-712f-4e12-8176-b65d5dd72091" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("8971ca36-712f-4e12-8176-b65d5dd72091")) {
Plotly.newPlot(
'8971ca36-712f-4e12-8176-b65d5dd72091',
[{"mode": "lines+markers", "name": "US", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 3, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 3, 0, 0, 0, 1, 0, 1, 20, 1, 1, 0, 0, 0, 0, 0, 0, 1, 19, 0, 0, 18, 0, 6, 1, 6, 0, 3, 14, 351, 511, 777, 823, 887, 1766, 2988, 4835, 5374, 7123, 8459, 11236, 8789, 13963, 16797, 0, 0, 22, 0, 0, 34, 0, 1, 74, 0, 0, 105, 0, 0, 95, 0, 0, 121, 0, 0, 200, 0, 1, 271, 0, 0, 287]}, {"mode": "lines+markers", "name": "Mexico", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 5, 10, 15, 12, 29, 11, 25, 46, 39, 48, 65, 51, 38, 70, 110, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 4]}, {"mode": "lines+markers", "name": "Canada", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 4, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 4, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 1, 2, 2, 0, 0, 3, 35, 38, 68, 60, 120, 145, 121, 156, 125, 260, 199, 216, 313, 1426, 633, 0, 0, 3, 0, 1, 3, 0, 0, 12, 0, 2, 6, 0, 0, 6, 0, 0, 5, 0, 0, 15, 0, 0, 16, 0, 0, 10]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('8971ca36-712f-4e12-8176-b65d5dd72091'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
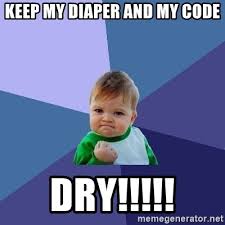
usa_border_buddies = ["United_States_of_America", "Mexico", "Canada"]
fig = go.Figure()
for country in usa_border_buddies:
df_country = df.loc[df["countriesAndTerritories"]==country]
geoId = df_country["geoId"].values[0]
trace = go.Scatter(x=df_country["dateRep"], y=df_country["cases"], mode="lines+markers", name=geoId)
fig.add_trace(trace)
fig.show()
<div id="9048f970-a386-494a-9e5f-558b8fa53bfa" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("9048f970-a386-494a-9e5f-558b8fa53bfa")) {
Plotly.newPlot(
'9048f970-a386-494a-9e5f-558b8fa53bfa',
[{"mode": "lines+markers", "name": "US", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 3, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 3, 0, 0, 0, 1, 0, 1, 20, 1, 1, 0, 0, 0, 0, 0, 0, 1, 19, 0, 0, 18, 0, 6, 1, 6, 0, 3, 14, 351, 511, 777, 823, 887, 1766, 2988, 4835, 5374, 7123, 8459, 11236, 8789, 13963, 16797, 0, 0, 22, 0, 0, 34, 0, 1, 74, 0, 0, 105, 0, 0, 95, 0, 0, 121, 0, 0, 200, 0, 1, 271, 0, 0, 287]}, {"mode": "lines+markers", "name": "MX", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 5, 10, 15, 12, 29, 11, 25, 46, 39, 48, 65, 51, 38, 70, 110, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 4]}, {"mode": "lines+markers", "name": "CA", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 4, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 4, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 1, 2, 2, 0, 0, 3, 35, 38, 68, 60, 120, 145, 121, 156, 125, 260, 199, 216, 313, 1426, 633, 0, 0, 3, 0, 1, 3, 0, 0, 12, 0, 2, 6, 0, 0, 6, 0, 0, 5, 0, 0, 15, 0, 0, 16, 0, 0, 10]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('9048f970-a386-494a-9e5f-558b8fa53bfa'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
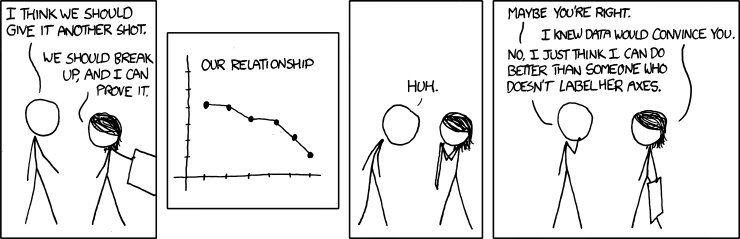
fig = go.Figure()
for country in usa_border_buddies:
df_country = df.loc[df["countriesAndTerritories"]==country]
geoId = df_country["geoId"].values[0]
trace = go.Scatter(x=df_country["dateRep"], y=df_country["cases"], mode="lines+markers", name=geoId)
fig.add_trace(trace)
# add labels
fig.update_layout(
title="Covid Cases for CA/MX/USA",
xaxis_title="Timeline",
yaxis_title="Number of Covid Cases"
)
fig.show()
<div id="acc77986-5b8d-46ec-9225-fbe18cd62542" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("acc77986-5b8d-46ec-9225-fbe18cd62542")) {
Plotly.newPlot(
'acc77986-5b8d-46ec-9225-fbe18cd62542',
[{"mode": "lines+markers", "name": "US", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 3, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 3, 0, 0, 0, 1, 0, 1, 20, 1, 1, 0, 0, 0, 0, 0, 0, 1, 19, 0, 0, 18, 0, 6, 1, 6, 0, 3, 14, 351, 511, 777, 823, 887, 1766, 2988, 4835, 5374, 7123, 8459, 11236, 8789, 13963, 16797, 0, 0, 22, 0, 0, 34, 0, 1, 74, 0, 0, 105, 0, 0, 95, 0, 0, 121, 0, 0, 200, 0, 1, 271, 0, 0, 287]}, {"mode": "lines+markers", "name": "MX", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 5, 10, 15, 12, 29, 11, 25, 46, 39, 48, 65, 51, 38, 70, 110, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 4]}, {"mode": "lines+markers", "name": "CA", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 4, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 4, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 1, 2, 2, 0, 0, 3, 35, 38, 68, 60, 120, 145, 121, 156, 125, 260, 199, 216, 313, 1426, 633, 0, 0, 3, 0, 1, 3, 0, 0, 12, 0, 2, 6, 0, 0, 6, 0, 0, 5, 0, 0, 15, 0, 0, 16, 0, 0, 10]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}, "title": {"text": "Covid Cases for CA/MX/USA"}, "xaxis": {"title": {"text": "Timeline"}}, "yaxis": {"title": {"text": "Number of Covid Cases"}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('acc77986-5b8d-46ec-9225-fbe18cd62542'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
fig = go.Figure()
for country in usa_border_buddies:
df_country = df.loc[df["countriesAndTerritories"]==country]
geoId = df_country["geoId"].values[0]
trace = go.Scatter(x=df_country["dateRep"], y=df_country["cases"], mode="lines+markers", name=geoId)
fig.add_trace(trace)
fig.update_layout(
title={
"text": "Covid Cases for CA/MX/USA",
"x":0.5,
"xanchor": "center"
},
xaxis_title="Timeline",
yaxis_title="Number of Covid Cases"
)
fig.show()
<div id="7915ee78-228b-4d22-957e-15b64d006909" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("7915ee78-228b-4d22-957e-15b64d006909")) {
Plotly.newPlot(
'7915ee78-228b-4d22-957e-15b64d006909',
[{"mode": "lines+markers", "name": "US", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 3, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 3, 0, 0, 0, 1, 0, 1, 20, 1, 1, 0, 0, 0, 0, 0, 0, 1, 19, 0, 0, 18, 0, 6, 1, 6, 0, 3, 14, 351, 511, 777, 823, 887, 1766, 2988, 4835, 5374, 7123, 8459, 11236, 8789, 13963, 16797, 0, 0, 22, 0, 0, 34, 0, 1, 74, 0, 0, 105, 0, 0, 95, 0, 0, 121, 0, 0, 200, 0, 1, 271, 0, 0, 287]}, {"mode": "lines+markers", "name": "MX", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 5, 10, 15, 12, 29, 11, 25, 46, 39, 48, 65, 51, 38, 70, 110, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 4]}, {"mode": "lines+markers", "name": "CA", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-03T00:00:00", "2020-03-13T00:00:00", "2020-03-14T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-04-03T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-05-03T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-06-03T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-07-03T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-09-03T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-10-03T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00", "2020-12-03T00:00:00"], "y": [0, 0, 1, 4, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 4, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 1, 2, 2, 0, 0, 3, 35, 38, 68, 60, 120, 145, 121, 156, 125, 260, 199, 216, 313, 1426, 633, 0, 0, 3, 0, 1, 3, 0, 0, 12, 0, 2, 6, 0, 0, 6, 0, 0, 5, 0, 0, 15, 0, 0, 16, 0, 0, 10]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}, "title": {"text": "Covid Cases for CA/MX/USA", "x": 0.5, "xanchor": "center"}, "xaxis": {"title": {"text": "Timeline"}}, "yaxis": {"title": {"text": "Number of Covid Cases"}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('7915ee78-228b-4d22-957e-15b64d006909'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
df.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Singapore | SG | SGP | 5638676.0 |
1 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Greece | EL | GRC | 10727668.0 |
2 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Lithuania | LT | LTU | 2789533.0 |
3 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Canada | CA | CAN | 37058856.0 |
4 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Brazil | BR | BRA | 209469333.0 |
df_total_cases = df[["cases", "countryterritoryCode", "day", "month", "year"]]
df_total_cases = df_total_cases.groupby(by="countryterritoryCode").agg(sum).reset_index()
df_total_cases.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
countryterritoryCode | cases | day | month | year | |
---|---|---|---|---|---|
0 | ABW | 28 | 135 | 18 | 12120 |
1 | AFG | 75 | 1257 | 152 | 157559 |
2 | AGO | 3 | 147 | 18 | 12120 |
3 | ALB | 174 | 342 | 57 | 38380 |
4 | AND | 224 | 275 | 42 | 28280 |
import plotly.express as px
df_total_cases = df[["cases", "countryterritoryCode", "day", "month", "year"]]
df_total_cases = df_total_cases.groupby(by="countryterritoryCode").agg(sum).reset_index()
df_total_cases.head()
fig = px.scatter_geo(df_total_cases,
animation_frame="day",
locations="countryterritoryCode",
locationmode="ISO-3",
size="cases")
fig.show()
<div id="b535edd7-deeb-40cd-8d58-29c0ba441d8a" class="plotly-graph-div" style="height:600px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("b535edd7-deeb-40cd-8d58-29c0ba441d8a")) {
Plotly.newPlot(
'b535edd7-deeb-40cd-8d58-29c0ba441d8a',
[{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=135<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ABW"], "marker": {"color": "#636efa", "size": [28], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}],
{"geo": {"center": {}, "domain": {"x": [0.0, 0.98], "y": [0.0, 1.0]}}, "height": 600, "legend": {"itemsizing": "constant", "tracegroupgap": 0}, "margin": {"t": 60}, "sliders": [{"active": 0, "currentvalue": {"prefix": "day="}, "len": 0.9, "pad": {"b": 10, "t": 60}, "steps": [{"args": [["135"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "135", "method": "animate"}, {"args": [["1257"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1257", "method": "animate"}, {"args": [["147"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "147", "method": "animate"}, {"args": [["342"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "342", "method": "animate"}, {"args": [["275"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "275", "method": "animate"}, {"args": [["1290"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1290", "method": "animate"}, {"args": [["345"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "345", "method": "animate"}, {"args": [["1277"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1277", "method": "animate"}, {"args": [["183"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "183", "method": "animate"}, {"args": [["1340"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1340", "method": "animate"}, {"args": [["1287"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1287", "method": "animate"}, {"args": [["242"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "242", "method": "animate"}, {"args": [["296"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "296", "method": "animate"}, {"args": [["266"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "266", "method": "animate"}, {"args": [["329"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "329", "method": "animate"}, {"args": [["1337"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1337", "method": "animate"}, {"args": [["221"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "221", "method": "animate"}, {"args": [["289"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "289", "method": "animate"}, {"args": [["1268"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1268", "method": "animate"}, {"args": [["102"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "102", "method": "animate"}, {"args": [["188"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "188", "method": "animate"}, {"args": [["1323"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1323", "method": "animate"}, {"args": [["225"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "225", "method": "animate"}, {"args": [["322"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "322", "method": "animate"}, {"args": [["276"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "276", "method": "animate"}, {"args": [["258"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "258", "method": "animate"}, {"args": [["364"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "364", "method": "animate"}, {"args": [["285"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "285", "method": "animate"}, {"args": [["298"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "298", "method": "animate"}, {"args": [["316"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "316", "method": "animate"}, {"args": [["168"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "168", "method": "animate"}, {"args": [["349"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "349", "method": "animate"}, {"args": [["110"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "110", "method": "animate"}, {"args": [["309"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "309", "method": "animate"}, {"args": [["207"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "207", "method": "animate"}, {"args": [["125"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "125", "method": "animate"}, {"args": [["1269"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1269", "method": "animate"}, {"args": [["1301"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1301", "method": "animate"}, {"args": [["1296"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1296", "method": "animate"}, {"args": [["1320"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1320", "method": "animate"}, {"args": [["1305"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1305", "method": "animate"}, {"args": [["287"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "287", "method": "animate"}, {"args": [["1299"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1299", "method": "animate"}, {"args": [["286"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "286", "method": "animate"}, {"args": [["1326"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1326", "method": "animate"}, {"args": [["271"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "271", "method": "animate"}, {"args": [["27"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "27", "method": "animate"}, {"args": [["273"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "273", "method": "animate"}, {"args": [["1333"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1333", "method": "animate"}, {"args": [["284"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "284", "method": "animate"}, {"args": [["1316"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1316", "method": "animate"}, {"args": [["362"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "362", "method": "animate"}, {"args": [["1291"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1291", "method": "animate"}, {"args": [["1331"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1331", "method": "animate"}, {"args": [["1327"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1327", "method": "animate"}, {"args": [["297"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "297", "method": "animate"}, {"args": [["1271"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1271", "method": "animate"}, {"args": [["53"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "53", "method": "animate"}, {"args": [["1324"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1324", "method": "animate"}, {"args": [["78"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "78", "method": "animate"}, {"args": [["1309"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1309", "method": "animate"}, {"args": [["317"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "317", "method": "animate"}, {"args": [["1275"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1275", "method": "animate"}, {"args": [["1289"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1289", "method": "animate"}, {"args": [["353"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "353", "method": "animate"}, {"args": [["321"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "321", "method": "animate"}, {"args": [["1238"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1238", "method": "animate"}, {"args": [["331"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "331", "method": "animate"}, {"args": [["327"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "327", "method": "animate"}, {"args": [["1286"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1286", "method": "animate"}, {"args": [["330"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "330", "method": "animate"}, {"args": [["119"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "119", "method": "animate"}, {"args": [["250"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "250", "method": "animate"}, {"args": [["1248"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1248", "method": "animate"}, {"args": [["1222"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1222", "method": "animate"}, {"args": [["1241"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1241", "method": "animate"}, {"args": [["333"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "333", "method": "animate"}, {"args": [["1322"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1322", "method": "animate"}, {"args": [["361"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "361", "method": "animate"}, {"args": [["375"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "375", "method": "animate"}, {"args": [["304"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "304", "method": "animate"}, {"args": [["341"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "341", "method": "animate"}, {"args": [["1313"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1313", "method": "animate"}, {"args": [["1332"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1332", "method": "animate"}, {"args": [["359"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "359", "method": "animate"}, {"args": [["314"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "314", "method": "animate"}, {"args": [["347"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "347", "method": "animate"}, {"args": [["358"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "358", "method": "animate"}, {"args": [["56"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "56", "method": "animate"}, {"args": [["280"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "280", "method": "animate"}, {"args": [["300"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "300", "method": "animate"}, {"args": [["336"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "336", "method": "animate"}, {"args": [["283"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "283", "method": "animate"}, {"args": [["1325"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "1325", "method": "animate"}, {"args": [["265"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "265", "method": "animate"}, {"args": [["91"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "91", "method": "animate"}], "x": 0.1, "xanchor": "left", "y": 0, "yanchor": "top"}], "template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}, "updatemenus": [{"buttons": [{"args": [null, {"frame": {"duration": 500, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 500, "easing": "linear"}}], "label": "▶", "method": "animate"}, {"args": [[null], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "◼", "method": "animate"}], "direction": "left", "pad": {"r": 10, "t": 70}, "showactive": false, "type": "buttons", "x": 0.1, "xanchor": "right", "y": 0, "yanchor": "top"}]},
{"responsive": true}
).then(function(){
Plotly.addFrames('b535edd7-deeb-40cd-8d58-29c0ba441d8a', [{"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=135<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ABW"], "marker": {"color": "#636efa", "size": [28], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "135"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1257<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["AFG"], "marker": {"color": "#636efa", "size": [75], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1257"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=147<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["AGO", "ERI", "TLS", "UGA"], "marker": {"color": "#636efa", "size": [3, 6, 1, 14], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "147"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=342<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ALB"], "marker": {"color": "#636efa", "size": [174], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "342"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=275<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["AND", "UKR"], "marker": {"color": "#636efa", "size": [224, 156], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "275"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1290<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ARE"], "marker": {"color": "#636efa", "size": [333], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1290"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=345<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ARG", "ZAF"], "marker": {"color": "#636efa", "size": [589, 927], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "345"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1277<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ARM", "LKA"], "marker": {"color": "#636efa", "size": [329, 106], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1277"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=183<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ATG", "SUR"], "marker": {"color": "#636efa", "size": [7, 8], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "183"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1340<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["AUS", "AUT", "BEL", "CAN", "CHE", "CHN", "CZE", "DEU", "DNK", "ESP", "FRA", "GBR", "IRN", "ISL", "ITA", "JPN", "KOR", "NLD", "NOR", "SGP", "SWE", "USA"], "marker": {"color": "#636efa", "size": [3166, 7029, 6235, 4018, 10714, 82079, 2062, 42288, 1877, 56188, 29155, 11658, 29406, 802, 80539, 1364, 9332, 7431, 3156, 594, 2806, 85991], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1340"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1287<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["AZE", "OMN", "THA"], "marker": {"color": "#636efa", "size": [122, 109, 1136], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1287"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=242<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BEN", "LBR", "SOM", "TZA"], "marker": {"color": "#636efa", "size": [6, 3, 2, 13], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "242"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=296<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BFA", "BOL"], "marker": {"color": "#636efa", "size": [146, 61], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "296"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=266<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BGD", "CUB"], "marker": {"color": "#636efa", "size": [48, 67], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "266"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=329<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BGR"], "marker": {"color": "#636efa", "size": [264], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "329"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1337<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BHR", "MYS", "SMR"], "marker": {"color": "#636efa", "size": [458, 2031, 218], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1337"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=221<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BHS"], "marker": {"color": "#636efa", "size": [9], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "221"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=289<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BIH", "CMR"], "marker": {"color": "#636efa", "size": [181, 88], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "289"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1268<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BLR", "MKD"], "marker": {"color": "#636efa", "size": [86, 201], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1268"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=102<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BLZ", "VIR"], "marker": {"color": "#636efa", "size": [2, 17], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "102"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=188<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BMU", "CYM", "FJI", "FRO", "GGY", "GIB", "GRL", "HTI", "JEY", "MUS", "TCD"], "marker": {"color": "#636efa", "size": [15, 6, 5, 140, 34, 35, 6, 8, 32, 81, 5], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "188"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1323<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BRA", "IRQ", "ISR"], "marker": {"color": "#636efa", "size": [2915, 382, 2666], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1323"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=225<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BRB", "GMB", "MNE"], "marker": {"color": "#636efa", "size": [24, 3, 67], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "225"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=322<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BRN"], "marker": {"color": "#636efa", "size": [114], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "322"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=276<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["BTN"], "marker": {"color": "#636efa", "size": [3], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "276"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=258<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CAF", "COG", "UZB", "XKX"], "marker": {"color": "#636efa", "size": [5, 4, 83, 79], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "258"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=364<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CHL"], "marker": {"color": "#636efa", "size": [1306], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "364"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=285<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CIV"], "marker": {"color": "#636efa", "size": [96], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "285"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=298<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["COD"], "marker": {"color": "#636efa", "size": [54], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "298"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=316<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["COL"], "marker": {"color": "#636efa", "size": [491], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "316"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=168<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CPV", "IMN", "MDG", "MSR", "NCL", "NER", "PNG", "ZWE"], "marker": {"color": "#636efa", "size": [5, 26, 23, 5, 15, 10, 1, 3], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "168"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=349<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CRI", "PER"], "marker": {"color": "#636efa", "size": [231, 580], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "349"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=110<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CUW"], "marker": {"color": "#636efa", "size": [7], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "110"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=309<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["CYP"], "marker": {"color": "#636efa", "size": [146], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "309"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=207<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["DJI", "GUM", "KGZ", "NIC", "PYF", "SLV", "ZMB"], "marker": {"color": "#636efa", "size": [18, 49, 44, 2, 30, 13, 14], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "207"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=125<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["DMA", "GRD", "MOZ", "SYR"], "marker": {"color": "#636efa", "size": [11, 7, 7, 5], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "125"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1269<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["DOM"], "marker": {"color": "#636efa", "size": [488], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1269"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1301<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["DZA", "RUS"], "marker": {"color": "#636efa", "size": [305, 840], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1301"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1296<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ECU"], "marker": {"color": "#636efa", "size": [1403], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1296"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1320<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["EGY"], "marker": {"color": "#636efa", "size": [456], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1320"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1305<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["EST"], "marker": {"color": "#636efa", "size": [538], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1305"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=287<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ETH", "KEN", "SDN"], "marker": {"color": "#636efa", "size": [12, 31, 3], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "287"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1299<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["FIN"], "marker": {"color": "#636efa", "size": [958], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1299"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=286<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GAB", "GHA"], "marker": {"color": "#636efa", "size": [7, 132], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "286"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1326<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GEO"], "marker": {"color": "#636efa", "size": [79], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1326"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=271<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GIN", "GUY"], "marker": {"color": "#636efa", "size": [5, 5], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "271"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=27<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GNB", "VGB"], "marker": {"color": "#636efa", "size": [2, 2], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "27"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=273<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GNQ", "GTM", "KAZ", "LCA", "MRT", "NAM", "RWA", "SWZ", "SYC", "URY", "VEN"], "marker": {"color": "#636efa", "size": [12, 25, 120, 3, 3, 8, 50, 6, 7, 238, 107], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "273"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1333<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["GRC"], "marker": {"color": "#636efa", "size": [892], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1333"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=284<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["HND", "JOR"], "marker": {"color": "#636efa", "size": [67, 172], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "284"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1316<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["HRV"], "marker": {"color": "#636efa", "size": [495], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1316"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=362<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["HUN"], "marker": {"color": "#636efa", "size": [300], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "362"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1291<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["IDN"], "marker": {"color": "#636efa", "size": [893], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1291"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1331<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["IND"], "marker": {"color": "#636efa", "size": [724], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1331"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1327<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["IRL"], "marker": {"color": "#636efa", "size": [1819], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1327"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=297<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["JAM"], "marker": {"color": "#636efa", "size": [26], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "297"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1271<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["KHM"], "marker": {"color": "#636efa", "size": [98], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1271"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=53<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["KNA", "MLI"], "marker": {"color": "#636efa", "size": [2, 4], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "53"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1324<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["KWT"], "marker": {"color": "#636efa", "size": [208], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1324"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=78<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LAO", "LBY", "TCA"], "marker": {"color": "#636efa", "size": [6, 1, 2], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "78"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1309<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LBN", "PAK"], "marker": {"color": "#636efa", "size": [368, 1197], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1309"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=317<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LIE"], "marker": {"color": "#636efa", "size": [56], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "317"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1275<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LTU"], "marker": {"color": "#636efa", "size": [299], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1275"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1289<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX"], "marker": {"color": "#636efa", "size": [1453], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1289"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=353<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["LVA"], "marker": {"color": "#636efa", "size": [244], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "353"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=321<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MAR"], "marker": {"color": "#636efa", "size": [275], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "321"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1238<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MCO"], "marker": {"color": "#636efa", "size": [33], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1238"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=331<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MDA"], "marker": {"color": "#636efa", "size": [177], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "331"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=327<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MDV"], "marker": {"color": "#636efa", "size": [13], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "327"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1286<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MEX"], "marker": {"color": "#636efa", "size": [585], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1286"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=330<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MLT", "SRB"], "marker": {"color": "#636efa", "size": [134, 384], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "330"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=119<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MMR"], "marker": {"color": "#636efa", "size": [5], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "119"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=250<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["MNG"], "marker": {"color": "#636efa", "size": [11], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "250"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1248<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["NGA"], "marker": {"color": "#636efa", "size": [65], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1248"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1222<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["NPL"], "marker": {"color": "#636efa", "size": [3], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1222"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1241<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["NZL"], "marker": {"color": "#636efa", "size": [338], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1241"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=333<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["PAN"], "marker": {"color": "#636efa", "size": [674], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "333"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1322<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["PHL", "VNM"], "marker": {"color": "#636efa", "size": [707, 153], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1322"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=361<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["POL"], "marker": {"color": "#636efa", "size": [1221], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "361"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=375<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["PRT"], "marker": {"color": "#636efa", "size": [3544], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "375"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=304<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["PRY"], "marker": {"color": "#636efa", "size": [52], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "304"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=341<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["PSE"], "marker": {"color": "#636efa", "size": [84], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "341"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1313<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["QAT"], "marker": {"color": "#636efa", "size": [549], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1313"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1332<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["ROU"], "marker": {"color": "#636efa", "size": [1029], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1332"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=359<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["SAU"], "marker": {"color": "#636efa", "size": [1012], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "359"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=314<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["SEN"], "marker": {"color": "#636efa", "size": [105], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "314"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=347<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["SVK"], "marker": {"color": "#636efa", "size": [226], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "347"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=358<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["SVN"], "marker": {"color": "#636efa", "size": [577], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "358"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=56<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["SXM"], "marker": {"color": "#636efa", "size": [2], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "56"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=280<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["TGO"], "marker": {"color": "#636efa", "size": [24], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "280"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=300<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["TTO"], "marker": {"color": "#636efa", "size": [65], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "300"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=336<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["TUN"], "marker": {"color": "#636efa", "size": [173], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "336"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=283<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["TUR"], "marker": {"color": "#636efa", "size": [3629], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "283"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=1325<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["TWN"], "marker": {"color": "#636efa", "size": [252], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "1325"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=265<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["VAT"], "marker": {"color": "#636efa", "size": [5], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "265"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "day=91<br>cases=%{marker.size}<br>countryterritoryCode=%{location}", "legendgroup": "", "locationmode": "ISO-3", "locations": ["VCT"], "marker": {"color": "#636efa", "size": [1], "sizemode": "area", "sizeref": 214.9775}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "91"}]);
}).then(function(){
var gd = document.getElementById('b535edd7-deeb-40cd-8d58-29c0ba441d8a'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
import plotly as py
df_total_cases = df[["cases", "countryterritoryCode"]]
df_total_cases = df_total_cases.groupby(by="countryterritoryCode").agg(sum).reset_index()
df_total_cases.head()
data = dict(
type = 'choropleth',
locations = df_total_cases["countryterritoryCode"],
locationmode ='ISO-3',
z=df_total_cases["cases"])
map = go.Figure(data=[data])
map.show()
<div id="a032dd92-de56-42cb-981c-98d8843e5d98" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("a032dd92-de56-42cb-981c-98d8843e5d98")) {
Plotly.newPlot(
'a032dd92-de56-42cb-981c-98d8843e5d98',
[{"locationmode": "ISO-3", "locations": ["ABW", "AFG", "AGO", "ALB", "AND", "ARE", "ARG", "ARM", "ATG", "AUS", "AUT", "AZE", "BEL", "BEN", "BFA", "BGD", "BGR", "BHR", "BHS", "BIH", "BLR", "BLZ", "BMU", "BOL", "BRA", "BRB", "BRN", "BTN", "CAF", "CAN", "CHE", "CHL", "CHN", "CIV", "CMR", "COD", "COG", "COL", "CPV", "CRI", "CUB", "CUW", "CYM", "CYP", "CZE", "DEU", "DJI", "DMA", "DNK", "DOM", "DZA", "ECU", "EGY", "ERI", "ESP", "EST", "ETH", "FIN", "FJI", "FRA", "FRO", "GAB", "GBR", "GEO", "GGY", "GHA", "GIB", "GIN", "GMB", "GNB", "GNQ", "GRC", "GRD", "GRL", "GTM", "GUM", "GUY", "HND", "HRV", "HTI", "HUN", "IDN", "IMN", "IND", "IRL", "IRN", "IRQ", "ISL", "ISR", "ITA", "JAM", "JEY", "JOR", "JPN", "KAZ", "KEN", "KGZ", "KHM", "KNA", "KOR", "KWT", "LAO", "LBN", "LBR", "LBY", "LCA", "LIE", "LKA", "LTU", "LUX", "LVA", "MAR", "MCO", "MDA", "MDG", "MDV", "MEX", "MKD", "MLI", "MLT", "MMR", "MNE", "MNG", "MOZ", "MRT", "MSR", "MUS", "MYS", "NAM", "NCL", "NER", "NGA", "NIC", "NLD", "NOR", "NPL", "NZL", "OMN", "PAK", "PAN", "PER", "PHL", "PNG", "POL", "PRT", "PRY", "PSE", "PYF", "QAT", "ROU", "RUS", "RWA", "SAU", "SDN", "SEN", "SGP", "SLV", "SMR", "SOM", "SRB", "SUR", "SVK", "SVN", "SWE", "SWZ", "SXM", "SYC", "SYR", "TCA", "TCD", "TGO", "THA", "TLS", "TTO", "TUN", "TUR", "TWN", "TZA", "UGA", "UKR", "URY", "USA", "UZB", "VAT", "VCT", "VEN", "VGB", "VIR", "VNM", "XKX", "ZAF", "ZMB", "ZWE"], "type": "choropleth", "z": [28, 75, 3, 174, 224, 333, 589, 329, 7, 3166, 7029, 122, 6235, 6, 146, 48, 264, 458, 9, 181, 86, 2, 15, 61, 2915, 24, 114, 3, 5, 4018, 10714, 1306, 82079, 96, 88, 54, 4, 491, 5, 231, 67, 7, 6, 146, 2062, 42288, 18, 11, 1877, 488, 305, 1403, 456, 6, 56188, 538, 12, 958, 5, 29155, 140, 7, 11658, 79, 34, 132, 35, 5, 3, 2, 12, 892, 7, 6, 25, 49, 5, 67, 495, 8, 300, 893, 26, 724, 1819, 29406, 382, 802, 2666, 80539, 26, 32, 172, 1364, 120, 31, 44, 98, 2, 9332, 208, 6, 368, 3, 1, 3, 56, 106, 299, 1453, 244, 275, 33, 177, 23, 13, 585, 201, 4, 134, 5, 67, 11, 7, 3, 5, 81, 2031, 8, 15, 10, 65, 2, 7431, 3156, 3, 338, 109, 1197, 674, 580, 707, 1, 1221, 3544, 52, 84, 30, 549, 1029, 840, 50, 1012, 3, 105, 594, 13, 218, 2, 384, 8, 226, 577, 2806, 6, 2, 7, 5, 2, 5, 24, 1136, 1, 65, 173, 3629, 252, 13, 14, 156, 238, 85991, 83, 5, 1, 107, 2, 17, 153, 79, 927, 14, 3]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('a032dd92-de56-42cb-981c-98d8843e5d98'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
import plotly.express as px
fig = px.histogram(df, x="cases")
fig.show()
<div id="c5ef8d6a-87d7-4180-bf29-2dafed277ea7" class="plotly-graph-div" style="height:600px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("c5ef8d6a-87d7-4180-bf29-2dafed277ea7")) {
Plotly.newPlot(
'c5ef8d6a-87d7-4180-bf29-2dafed277ea7',
[{"alignmentgroup": "True", "bingroup": "x", "hoverlabel": {"namelength": 0}, "hovertemplate": "cases=%{x}<br>count=%{y}", "legendgroup": "", "marker": {"color": "#636efa"}, "name": "", "offsetgroup": "", "orientation": "v", "showlegend": false, "type": "histogram", "x": [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 27, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 5, 0, 0, 0, 0, 0, 2095, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 5, 2, 0, 0, 0, 0, 3, 1, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 54, 9, 0, 1, 0, 0, 3, 5, 0, 595, 0, 574, 0, 0, 2, 2, 0, 3, 0, 0, 1, 32, 1, 3, 205, 0, 9, 0, 4, 4, 3, 1, 0, 0, 1, 240, 1, 0, 0, 0, 0, 43, 0, 1, 0, 1, 0, 2, 0, 6, 1, 0, 6, 1, 0, 0, 5, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 17, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 136, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 19, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 151, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 140, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 97, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 259, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 2, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 441, 0, 0, 1, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 665, 0, 3, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 787, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 6, 0, 1753, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 1, 0, 0, 0, 0, 1466, 0, 3, 2, 0, 0, 0, 0, 0, 0, 3, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 1740, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 1, 0, 3, 1, 1980, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2590, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 6, 3, 3, 2, 385, 4, 1, 1, 1, 20, 1, 2, 0, 2, 17, 6, 6, 0, 1, 0, 18, 0, 5, 0, 7, 0, 3, 0, 0, 205, 561, 13, 1, 0, 0, 0, 0, 2, 2, 686, 1, 0, 0, 0, 6, 0, 4, 6, 0, 4, 30, 0, 4, 0, 0, 0, 0, 1, 4, 2, 0, 0, 0, 0, 3, 15, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 15141, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 1, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 8, 0, 0, 0, 47, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 4156, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2538, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 9, 0, 0, 0, 8, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 5, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 14, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 134, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2007, 0, 0, 1, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 7, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 2, 0, 1, 0, 0, 0, 2052, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1890, 0, 0, 0, 99, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 7, 0, 0, 0, 0, 0, 0, 0, 15, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 88, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0, 1750, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 18, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 34, 0, 0, 394, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 79, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 75, 0, 0, 0, 0, 0, 13, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 891, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 9, 0, 0, 0, 0, 0, 0, 2, 12, 1, 826, 0, 0, 19, 0, 0, 0, 0, 0, 0, 0, 0, 14, 0, 0, 0, 190, 0, 4, 1, 0, 0, 2, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 13, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 647, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 10, 0, 0, 0, 3, 0, 0, 0, 27, 0, 0, 0, 0, 0, 62, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 256, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 53, 0, 0, 4, 0, 161, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 12, 0, 0, 0, 0, 218, 57, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 15, 0, 0, 3, 0, 0, 0, 0, 0, 1, 2, 0, 0, 0, 0, 0, 0, 0, 18, 0, 0, 2, 1, 0, 0, 0, 0, 0, 0, 2, 0, 130, 1, 0, 2, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 18, 515, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 97, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 410, 0, 1, 0, 0, 0, 0, 0, 0, 0, 20, 0, 0, 2, 0, 0, 0, 0, 1, 0, 3, 1, 0, 0, 0, 2, 2, 0, 0, 0, 6, 254, 0, 0, 0, 0, 0, 1, 0, 0, 93, 0, 0, 4, 1, 0, 0, 0, 0, 0, 0, 0, 1, 4, 0, 21, 0, 0, 2, 0, 0, 34, 0, 0, 1, 2, 78, 1, 1, 0, 0, 1, 2, 10, 0, 22, 0, 1, 0, 0, 0, 4, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 439, 0, 0, 6, 0, 1, 0, 5, 0, 14, 3, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 15, 0, 44, 0, 1, 0, 0, 449, 0, 0, 0, 0, 0, 0, 1, 329, 0, 1, 1, 0, 7, 0, 0, 0, 0, 0, 2, 0, 1, 427, 1, 2, 13, 106, 1, 0, 2, 5, 250, 3, 0, 0, 1, 1, 6, 0, 0, 0, 21, 0, 0, 26, 3, 0, 0, 0, 0, 0, 0, 2, 0, 1, 1, 3, 0, 1, 17, 3, 0, 0, 0, 0, 1, 1, 24, 0, 0, 0, 0, 1, 0, 0, 0, 6, 0, 0, 2, 1, 0, 1, 0, 4, 0, 2, 1, 0, 0, 1, 2, 9, 0, 1, 0, 2, 2, 0, 2, 1, 0, 2, 1, 2, 428, 909, 0, 0, 5, 0, 0, 4, 0, 0, 0, 1, 0, 1, 0, 0, 10, 0, 0, 238, 5, 0, 2, 0, 0, 0, 0, 2, 2, 0, 0, 20, 2, 19, 5, 143, 0, 0, 0, 0, 17, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2812, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 6, 1, 28, 5, 1, 146, 2, 1, 2, 0, 1, 48, 4, 6, 2, 3, 4, 1, 1, 127, 1, 1, 31, 600, 1, 4, 10, 523, 2, 3, 1, 14, 2, 1, 1, 6, 1, 1, 3, 96, 35, 1, 158, 19, 2, 4, 1075, 19, 20, 12, 4, 5, 2, 11, 1, 3, 595, 9, 5, 5, 6, 212, 4, 34, 8, 0, 13, 2, 0, 110, 134, 1, 5, 9, 6, 115, 3, 1, 14, 32, 1, 9, 3, 56, 4, 1, 111, 1, 1, 11, 1, 6, 9, 2, 0, 22, 11, 39, 160, 2651, 85, 1, 17, 2, 132, 22, 1, 351, 1, 1, 864, 14, 2, 1, 12, 1, 30, 2, 25, 0, 18, 1, 2, 10, 27, 16, 5, 802, 2, 19, 10, 3, 7, 62, 39, 8, 21, 190, 48, 1, 0, 11, 3, 12, 7, 12, 160, 1, 5, 1, 3, 3, 20, 1, 267, 143, 2, 4, 117, 12, 3, 41, 10, 20, 1, 1, 511, 2, 2547, 21, 2, 34, 1, 785, 0, 34, 1, 128, 0, 8, 1, 57, 9, 3, 1, 16, 17, 1289, 5, 4, 9, 1, 7, 8, 3, 6, 107, 1227, 7, 45, 15, 35, 19, 0, 2, 9, 10, 1, 52, 13, 13, 6, 6, 6, 693, 155, 38, 19, 1, 13, 58, 24, 25, 12, 5, 52, 0, 21, 0, 777, 0, 6, 0, 5, 2, 130, 0, 0, 90, 17, 14, 4, 40, 151, 0, 18, 0, 23, 6, 0, 7, 0, 62, 1, 7, 19, 3, 5, 6, 76, 0, 14, 36, 3, 0, 7, 36, 2, 0, 0, 11, 3, 11, 0, 1, 0, 1, 4, 0, 38, 7, 6, 6, 6, 7, 11, 433, 1, 5, 2, 2, 47, 5, 0, 155, 2, 0, 15, 10, 0, 57, 43, 0, 838, 0, 1, 9, 0, 1, 12, 1, 0, 0, 27, 23, 2, 733, 14, 6, 64, 286, 1, 1, 2, 238, 10, 18, 41, 5, 1522, 22, 3, 38, 1365, 3, 149, 68, 27, 1, 0, 1, 0, 24, 0, 55, 0, 39, 27, 12, 2, 3, 11, 56, 34, 251, 3, 1, 2, 9, 28, 21, 0, 1209, 40, 25, 205, 1, 170, 32, 1, 1, 8, 0, 0, 12, 40, 0, 2, 3, 4, 10, 38, 0, 6, 0, 3, 6, 3, 4, 2, 8, 84, 197, 5, 3, 841, 0, 6230, 14, 0, 48, 103, 6, 8, 3, 1, 0, 29, 0, 0, 0, 6, 1, 2, 823, 3, 1, 64, 11, 9, 14, 5, 17, 0, 1, 0, 0, 16, 76, 2, 11, 0, 3, 79, 0, 924, 10, 60, 10, 5, 21, 15, 108, 8, 176, 49, 190, 6, 11, 39, 9, 10, 74, 57, 4, 5, 2, 4, 0, 5, 4, 0, 1043, 0, 2000, 26, 0, 0, 72, 32, 4, 0, 3, 17, 46, 1, 5, 0, 1, 1, 0, 0, 11, 9, 17, 2, 156, 29, 12, 4, 0, 57, 0, 7, 18, 152, 0, 2, 0, 21, 1174, 5, 6, 15, 0, 0, 16, 77, 21, 1, 89, 38, 887, 0, 4, 3, 52, 3, 1, 22, 120, 0, 0, 86, 0, 5, 1, 2, 2, 0, 1, 10, 0, 5, 9, 0, 0, 0, 11, 21, 2, 17, 26, 4, 0, 12, 1, 21, 15, 1053, 1, 0, 6, 156, 2, 29, 12, 10, 92, 110, 125, 32, 34, 0, 63, 2, 21, 4, 10, 45, 10, 278, 81, 1, 0, 1, 2, 0, 8, 2, 0, 0, 6, 9, 8, 34, 4, 0, 1210, 2, 0, 54, 12, 30, 0, 34, 4000, 199, 84, 1438, 1, 1, 9, 17, 1, 11, 11, 9, 0, 9, 23, 3, 0, 8, 0, 3526, 35, 17, 53, 1, 69, 7, 79, 12, 0, 3, 2, 158, 40, 1, 3, 4, 7, 30, 0, 9, 17, 167, 4, 0, 0, 20, 0, 0, 0, 23, 93, 316, 21, 11, 15, 12, 45, 38, 0, 12, 21, 4, 0, 0, 48, 0, 1987, 2, 1, 33, 9, 0, 0, 0, 1178, 5, 407, 1144, 0, 13, 2, 2, 0, 31, 0, 0, 0, 25, 90, 0, 0, 1, 14, 0, 16, 8, 2, 15, 13, 10, 3, 1, 0, 1, 61, 0, 92, 9, 0, 8, 117, 0, 8, 1, 13, 45, 1097, 51, 0, 19, 139, 145, 0, 0, 3, 0, 292, 0, 120, 57, 26, 450, 59, 0, 1, 0, 0, 6, 46, 19, 0, 22, 0, 0, 2, 3, 1766, 0, 33, 2, 0, 0, 47, 1, 1, 23, 0, 22, 2, 18, 28, 0, 6, 37, 115, 2538, 152, 0, 30, 3, 1, 33, 134, 0, 2, 5, 111, 38, 194, 10, 0, 10, 49, 1042, 15, 2, 6, 10, 1, 0, 43, 5, 0, 31, 12, 12, 8, 0, 0, 19, 137, 13, 50, 57, 37, 2988, 0, 4, 44, 8, 1404, 1, 91, 0, 360, 0, 47, 0, 3, 6, 0, 117, 121, 1, 10, 0, 0, 4, 11, 10, 15, 74, 0, 3, 17, 0, 11, 2, 5, 0, 10, 93, 37, 11, 115, 2, 346, 2, 5, 70, 2, 10, 88, 5, 18, 1, 0, 1, 0, 19, 2, 18, 3, 10, 0, 4, 75, 0, 0, 1, 680, 15, 39, 1192, 4, 28, 3, 29, 15, 2, 25, 0, 31, 0, 314, 22, 11, 3, 0, 2, 1, 0, 13, 6, 12, 243, 31, 6, 33, 7, 1, 23, 4207, 23, 3, 0, 0, 5, 15, 0, 0, 31, 72, 46, 0, 15, 0, 14, 0, 52, 3, 1, 17, 9, 110, 1, 8, 0, 135, 31, 11, 0, 0, 0, 1, 22, 1861, 0, 9, 9, 156, 0, 1, 11, 0, 33, 2, 2, 0, 0, 5, 12, 309, 5322, 0, 0, 80, 35, 12, 34, 0, 0, 2, 2, 13, 27, 7, 172, 9, 0, 1, 176, 168, 0, 77, 10, 1, 409, 1, 1, 2, 0, 13, 104, 1, 11, 878, 7, 0, 0, 0, 1046, 6, 0, 122, 13, 4, 1, 1, 0, 191, 99, 11, 87, 23, 3, 244, 26, 3, 18, 8, 0, 0, 193, 26, 3431, 5, 129, 55, 0, 36, 16, 0, 2, 0, 15, 0, 3, 32, 16, 550, 28, 32, 89, 4, 647, 10, 5, 2, 5940, 0, 0, 7, 0, 143, 0, 68, 67, 17, 4835, 2, 0, 144, 13, 31, 6, 10, 4, 0, 12, 0, 6, 5, 0, 46, 2, 28, 0, 3, 9, 14, 190, 17, 36, 23, 0, 0, 1, 4, 0, 25, 5374, 22, 11, 6, 0, 200, 0, 22, 235, 0, 0, 0, 0, 70, 16, 952, 1, 17, 13, 2833, 31, 1, 55, 3, 9, 29, 0, 1, 706, 0, 8, 5, 1, 10, 63, 2, 0, 40, 10, 154, 39, 1, 27, 311, 11, 0, 54, 9, 15, 9, 534, 147, 1, 14, 2, 1, 0, 1, 1, 79, 7, 9, 5986, 4, 21, 0, 1, 0, 12, 0, 30, 35, 0, 5, 0, 462, 0, 0, 0, 0, 126, 0, 30, 1, 4, 0, 139, 0, 0, 16, 0, 13, 3, 4, 5, 50, 22, 165, 14, 5, 453, 0, 11, 14, 0, 3, 26, 0, 8, 46, 0, 0, 57, 16, 2, 227, 12, 283, 1617, 2, 0, 7, 13, 4, 2, 1, 0, 1, 25, 4049, 0, 125, 2, 210, 18, 7, 0, 0, 0, 2, 23, 31, 82, 130, 104, 13, 0, 1237, 3, 40, 22, 9, 0, 16, 0, 5, 2, 3, 79, 92, 1, 3, 106, 184, 0, 15, 83, 0, 3276, 9, 39, 150, 13, 21, 1, 0, 1847, 0, 91, 0, 0, 0, 8, 71, 64, 3, 103, 224, 3, 17, 0, 5, 375, 59, 12, 1035, 29, 4, 17, 0, 4, 0, 2, 0, 0, 41, 4, 10, 15, 0, 0, 89, 1, 4, 6, 1, 4, 233, 42, 0, 0, 0, 0, 0, 0, 2, 260, 0, 966, 102, 0, 10, 0, 5, 224, 4946, 21, 0, 18, 558, 15, 48, 36, 0, 1, 1237, 0, 14, 1, 9, 52, 151, 40, 7, 9, 0, 6, 260, 78, 111, 118, 1, 0, 1, 637, 123, 4, 6557, 6, 171, 47, 11, 71, 19, 0, 8, 13, 2, 0, 141, 55, 28, 1, 2, 277, 24, 186, 13, 9, 2, 67, 6, 14, 0, 2, 0, 1, 0, 4, 1, 25, 48, 2, 23, 1, 0, 0, 4, 24, 0, 0, 45, 53, 6, 2, 67, 0, 98, 0, 153, 4, 35, 7123, 7, 0, 0, 0, 35, 12, 36, 0, 80, 13, 0, 0, 0, 9, 206, 257, 2, 6, 3, 0, 573, 1, 0, 0, 0, 0, 43, 0, 23, 0, 1559, 4, 24, 150, 3311, 0, 0, 28, 0, 0, 12, 320, 12, 0, 0, 0, 1, 2, 894, 15, 122, 199, 1, 69, 138, 5, 5560, 13, 94, 36, 0, 3, 11, 38, 0, 0, 64, 0, 36, 0, 0, 0, 1, 34, 10, 3, 3, 188, 0, 35, 0, 586, 13, 0, 23, 0, 3, 119, 3, 14, 289, 0, 8, 90, 65, 160, 7, 15, 132, 16, 1, 45, 5, 0, 1, 128, 39, 3646, 68, 6, 1, 0, 0, 25, 0, 30, 95, 19, 611, 14, 7, 7, 29, 17, 0, 3, 41, 7, 8, 33, 607, 5, 0, 2, 1, 665, 66, 3, 12, 7, 29, 0, 1, 1, 22, 0, 12, 18, 0, 19, 17, 8, 9, 64, 170, 25, 0, 0, 0, 8459, 418, 10, 0, 98, 4, 0, 0, 31, 0, 1028, 119, 1, 13, 0, 123, 20, 121, 0, 0, 0, 5, 15, 95, 105, 10, 0, 23, 11, 0, 8, 1, 48, 71, 128, 34, 40, 115, 33, 65, 17, 7, 7, 5, 29, 0, 0, 345, 0, 20, 16, 5, 21, 1, 0, 11, 0, 3, 10, 1044, 5, 3, 219, 0, 1, 1, 0, 11, 3, 0, 460, 0, 3, 0, 1, 8, 371, 0, 22, 30, 51, 35, 32, 11, 4, 4517, 0, 342, 0, 77, 1, 0, 106, 54, 2, 0, 3, 1411, 293, 2, 19, 30, 15, 1, 24, 0, 0, 20, 6, 2, 2, 0, 5, 76, 2, 0, 53, 3838, 26, 99, 3, 7, 33, 0, 17, 0, 3, 212, 36, 13, 1, 0, 4438, 0, 4, 41, 28, 0, 74, 71, 2, 11236, 114, 114, 8, 19, 0, 11, 0, 10, 17, 6, 0, 6, 71, 143, 1, 1, 3, 0, 967, 0, 0, 0, 0, 855, 2, 4, 2, 0, 0, 0, 8, 0, 51, 110, 3, 87, 43, 14, 0, 0, 6, 3, 0, 216, 4789, 71, 103, 12, 0, 36, 3, 65, 0, 20, 0, 5, 1, 239, 3, 0, 45, 26, 39, 1, 32, 5, 0, 82, 0, 0, 192, 1, 14, 545, 16, 39, 5, 1762, 313, 0, 2, 0, 18, 2, 107, 19, 176, 8, 0, 1, 774, 0, 131, 0, 106, 12, 0, 13, 0, 4, 60, 195, 24, 0, 6, 90, 19, 0, 49, 256, 0, 70, 2, 23, 17, 158, 31, 99, 1, 57, 48, 2342, 526, 205, 1, 10, 0, 2446, 27, 4, 15, 39, 0, 0, 100, 3, 15, 0, 0, 51, 0, 50, 11, 67, 343, 3, 1, 0, 13, 0, 1, 2, 0, 38, 21, 1, 1, 65, 101, 6, 25, 0, 0, 4, 796, 4, 48, 0, 37, 5, 26, 600, 0, 8789, 2, 92, 76, 17, 0, 0, 0, 1427, 0, 1, 0, 19, 9, 4, 16, 17, 2, 71, 0, 0, 186, 2, 20, 1, 36, 1, 811, 0, 1, 0, 6584, 21, 86, 310, 107, 0, 6, 0, 6, 0, 27, 0, 33, 11, 4, 1, 0, 8, 0, 0, 0, 302, 2, 5, 4, 204, 224, 0, 155, 2, 42, 72, 6, 2, 152, 98, 5249, 7, 1, 30, 0, 104, 0, 2, 10, 0, 0, 47, 2, 38, 50, 25, 488, 9, 7, 13, 26, 11, 36, 8, 1, 16, 145, 7, 15, 78, 1, 0, 84, 7, 66, 2, 4, 0, 15, 3, 142, 104, 7937, 0, 152, 35, 121, 0, 5, 89, 0, 9, 0, 24, 0, 92, 0, 0, 29, 129, 75, 85, 0, 29, 1, 48, 2931, 76, 6, 0, 26, 21, 0, 65, 15, 9, 852, 70, 0, 0, 5, 5210, 0, 13, 3, 4, 6, 14, 80, 59, 561, 0, 5, 133, 0, 2, 1, 0, 0, 4, 2, 0, 13963, 0, 24, 0, 88, 29, 4, 0, 10, 25, 24, 28, 2, 0, 0, 1, 0, 4, 1, 8, 55, 0, 144, 10, 4, 1452, 0, 1, 606, 33, 2, 4, 6, 5, 0, 94, 238, 163, 133, 104, 1426, 0, 5, 350, 10, 925, 2206, 15, 0, 0, 29, 22, 35, 5, 0, 0, 220, 1, 0, 260, 0, 0, 0, 0, 12, 87, 1, 9, 1, 4954, 0, 172, 33, 150, 3, 0, 0, 3, 1, 0, 0, 0, 633, 2, 111, 9, 115, 5, 0, 0, 1, 7, 235, 234, 23, 11, 30, 0, 19, 232, 54, 668, 19, 0, 3, 0, 7, 9, 24, 439, 2, 134, 2, 182, 11, 0, 8, 49, 192, 0, 0, 549, 14, 6, 81, 6, 170, 4, 0, 71, 153, 9, 5780, 0, 10, 408, 26, 1, 1, 64, 78, 0, 9, 9, 0, 0, 123, 0, 10, 22, 3922, 14, 112, 96, 6, 5, 12, 1, 1, 0, 71, 255, 14, 3, 0, 0, 9, 5, 1, 0, 0, 0, 91, 3, 297, 18, 1298, 0, 21, 55, 22, 0, 25, 1, 16, 1, 120, 2, 1000, 2389, 33, 32, 5, 1, 110, 24, 22, 7, 2, 1, 4, 36, 17, 482, 4, 0, 1, 0, 32, 0, 6, 4, 2, 2, 2129, 8, 50, 2, 4, 14, 1, 35, 743, 1141, 23, 4, 16797, 0, 13, 633, 19, 9, 6153, 5, 6, 0, 9, 0, 1196, 1, 0, 14, 43, 2, 1019, 96, 39, 0, 28, 39, 4, 29, 1, 103, 41, 0, 4, 36, 2, 15, 1, 4, 0, 1, 140, 111, 2, 0, 235, 1, 28, 21, 4, 8578, 0, 75, 2, 1, 3, 1, 12, 0, 6, 5, 10, 296, 86, 65, 218, 10, 87, 0, 22, 30, 240, 91, 2, 77, 164, 0, 1, 39, 4, 16, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 3237, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 22, 2, 1, 3, 1, 2, 5, 1, 1, 5, 835, 7, 1, 3, 2, 9, 3, 0, 8, 1, 39, 10, 1, 119, 37, 1, 1, 516, 1, 5, 1, 1, 11, 14, 6, 2, 34, 2, 1, 6, 8, 10, 667, 7, 1, 0, 0, 0, 0, 0, 0, 0, 15, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 1, 0, 6, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 6, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 10, 0, 5, 0, 0, 0, 2, 0, 0, 3872, 0, 0, 1, 0, 0, 1, 0, 73, 2, 0, 10, 2, 1, 49, 3, 4, 587, 11, 1, 7, 3, 20, 586, 23, 22, 1, 10, 5, 2, 2, 2, 117, 49, 4, 10, 438, 1, 3, 2, 1, 5, 66, 3, 8, 3, 14, 5, 34, 11, 5, 34, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 1, 0, 0, 5, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 10, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3727, 0, 0, 0, 0, 0, 0, 0, 2, 12, 1, 3, 5, 26, 138, 0, 61, 2, 769, 5, 4, 7, 2, 170, 22, 1, 7, 518, 7, 7, 74, 1, 2, 5, 30, 1, 1, 2, 30, 1, 1, 591, 1, 5, 9, 3, 27, 30, 1, 3, 5, 3, 138, 0, 4, 1, 7, 44, 2, 10, 4, 32, 6, 12, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 41, 3160, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 5, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 0, 0, 2, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 5, 5, 76, 1, 48, 12, 1, 4, 8, 1, 190, 6, 6, 7, 1, 2, 101, 4, 7, 1, 3, 2, 778, 2, 6, 1, 113, 284, 2, 1, 33, 105, 1, 1, 2, 4, 1234, 1, 13, 13, 3, 3, 33, 6, 27, 46, 1, 483, 59, 3, 2, 9, 1, 4, 1, 3, 1, 122, 1, 1, 59, 1, 5, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 7, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 0, 0, 5, 0, 0, 0, 0, 3418, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 43, 3, 2, 1, 4, 6, 11, 16, 24, 10, 2, 2, 163, 3, 1, 46, 3, 0, 1, 7, 1, 3, 1, 1, 1, 1247, 16, 56, 1, 2, 1076, 3, 8, 21, 25, 47, 1, 60, 8, 0, 8, 6, 3, 60, 367, 3, 1, 6, 7, 0, 34, 95, 103, 1, 10, 3, 55, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 2607, 0, 7, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 5, 7, 6, 3, 4, 2, 248, 31, 14, 9, 1, 7, 2, 4, 159, 2, 4, 2, 1, 45, 55, 1492, 3, 6, 2, 12, 12, 22, 67, 11, 3, 2, 68, 2, 34, 743, 1, 5, 6, 42, 3, 11, 1, 2, 6, 1, 121, 2, 3, 410, 33, 4, 9, 16, 1, 10, 77, 5, 7, 2, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 2974, 0, 0, 1, 0, 0, 0, 0, 0, 6, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, -9, 3, 75, 30, 9, 26, 10, 595, 3, 2, 10, 6, 14, 1, 45, 1, 2, 3, 15, 56, 9, 1, 10, 10, 4, 200, 2, 4, 3, 23, 42, 12, 2, 18, 1, 1, 1, 2, 131, 1, 2, 23, 39, 48, 11, 4, 1, 1, 286, 8, 0, 20, 6, 4, 1, 10, 1, 1797, 29, 20, 237, 615, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 4, 65, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2490, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 4, 2, 2, 881, 3, 13, 2, 8, 4, 16, 12, 78, 2, 12, 9, 0, 0, 29, 977, 157, 7, 23, 2, 0, 14, 2, 2, 3, 15, 4, 3, 6, 9, 435, 4, 6, 31, 242, 28, 1, 4, 9, 2, 0, 4, 3, 4, 5, 1, 116, 51, 3, 372, 13, 85, 52, 2, 4, 2, 6, 3, 6, 5, 5, 151, 6, 0, 61, 54, 2, 1, 271, 4, 15, 8, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 39, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 2028, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 7, 13, 20, 1, 9, 26, 18, 3, 238, 25, 3, 497, 3, 4, 4, 16, 31, 20, 271, 19, 12, 252, 6, 9, 1, 114, 10, 18, 9, 1, 0, 2, 8, 11, 47, 12, 3, 2313, 2, 1, 121, 2, 51, 52, 2, 3, 3, 3, 83, 64, 20, 14, 2, 152, 3, 958, 0, 3, 1, 1, 9, 2, 4, 6, 2, 4, 1, 501, 0, 2, 6, 24, 6, 1, 23, 287, 212, 4, 0, 10, 2, 136, 15, 1, 0, 15, 15], "xaxis": "x", "yaxis": "y"}],
{"barmode": "relative", "height": 600, "legend": {"tracegroupgap": 0}, "margin": {"t": 60}, "template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}, "xaxis": {"anchor": "y", "domain": [0.0, 0.98], "title": {"text": "cases"}}, "yaxis": {"anchor": "x", "domain": [0.0, 1.0], "title": {"text": "count"}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('c5ef8d6a-87d7-4180-bf29-2dafed277ea7'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
df_Afg.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
20 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
131 | 2020-01-01 | 1 | 1 | 2020 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
190 | 2020-01-02 | 1 | 2 | 2020 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
261 | 2020-01-03 | 1 | 3 | 2020 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
271 | 2020-01-13 | 13 | 1 | 2020 | 0 | 0 | Afghanistan | AF | AFG | 37172386.0 |
df_cumulative_sum_Afg = pd.DataFrame()
df_cumulative_sum_Afg["cases"] = df_Afg["cases"].cumsum()
df_cumulative_sum_Afg["dateRep"] = df_Afg["dateRep"]
df_cumulative_sum_Afg.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
cases | dateRep | |
---|---|---|
20 | 0 | 2019-12-31 |
131 | 0 | 2020-01-01 |
190 | 0 | 2020-01-02 |
261 | 0 | 2020-01-03 |
271 | 0 | 2020-01-13 |
fig = go.Figure()
trace1 = go.Scatter(x=df_cumulative_sum_Afg["dateRep"],
y=df_cumulative_sum_Afg["cases"],
mode="lines+markers", name="Afg")
fig.add_trace(trace1)
<div id="49d8794e-e35f-47de-be9b-48d18c33ad06" class="plotly-graph-div" style="height:525px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("49d8794e-e35f-47de-be9b-48d18c33ad06")) {
Plotly.newPlot(
'49d8794e-e35f-47de-be9b-48d18c33ad06',
[{"mode": "lines+markers", "name": "Afg", "type": "scatter", "x": ["2019-12-31T00:00:00", "2020-01-01T00:00:00", "2020-01-02T00:00:00", "2020-01-03T00:00:00", "2020-01-13T00:00:00", "2020-01-14T00:00:00", "2020-01-15T00:00:00", "2020-01-16T00:00:00", "2020-01-17T00:00:00", "2020-01-18T00:00:00", "2020-01-19T00:00:00", "2020-01-20T00:00:00", "2020-01-21T00:00:00", "2020-01-22T00:00:00", "2020-01-23T00:00:00", "2020-01-24T00:00:00", "2020-01-25T00:00:00", "2020-01-26T00:00:00", "2020-01-27T00:00:00", "2020-01-28T00:00:00", "2020-01-29T00:00:00", "2020-01-30T00:00:00", "2020-01-31T00:00:00", "2020-02-01T00:00:00", "2020-02-02T00:00:00", "2020-02-03T00:00:00", "2020-02-13T00:00:00", "2020-02-14T00:00:00", "2020-02-15T00:00:00", "2020-02-16T00:00:00", "2020-02-17T00:00:00", "2020-02-18T00:00:00", "2020-02-19T00:00:00", "2020-02-20T00:00:00", "2020-02-21T00:00:00", "2020-02-22T00:00:00", "2020-02-23T00:00:00", "2020-02-24T00:00:00", "2020-02-25T00:00:00", "2020-02-26T00:00:00", "2020-02-27T00:00:00", "2020-02-28T00:00:00", "2020-02-29T00:00:00", "2020-03-01T00:00:00", "2020-03-02T00:00:00", "2020-03-15T00:00:00", "2020-03-16T00:00:00", "2020-03-17T00:00:00", "2020-03-18T00:00:00", "2020-03-19T00:00:00", "2020-03-20T00:00:00", "2020-03-21T00:00:00", "2020-03-22T00:00:00", "2020-03-23T00:00:00", "2020-03-24T00:00:00", "2020-03-25T00:00:00", "2020-03-26T00:00:00", "2020-03-27T00:00:00", "2020-04-01T00:00:00", "2020-04-02T00:00:00", "2020-05-01T00:00:00", "2020-05-02T00:00:00", "2020-06-01T00:00:00", "2020-06-02T00:00:00", "2020-07-01T00:00:00", "2020-07-02T00:00:00", "2020-08-01T00:00:00", "2020-08-02T00:00:00", "2020-08-03T00:00:00", "2020-09-01T00:00:00", "2020-09-02T00:00:00", "2020-10-01T00:00:00", "2020-10-02T00:00:00", "2020-11-01T00:00:00", "2020-11-02T00:00:00", "2020-11-03T00:00:00", "2020-12-01T00:00:00", "2020-12-02T00:00:00"], "y": [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 4, 10, 15, 16, 16, 16, 18, 18, 28, 34, 36, 69, 69, 69, 69, 69, 69, 69, 69, 69, 69, 69, 69, 72, 72, 72, 72, 72, 72, 72, 75, 75, 75]}],
{"template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}},
{"responsive": true}
).then(function(){
var gd = document.getElementById('49d8794e-e35f-47de-be9b-48d18c33ad06'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>
df.head(2)
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Singapore | SG | SGP | 5638676.0 |
1 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Greece | EL | GRC | 10727668.0 |
df["cases_sum"] = df["cases"].cumsum()
df.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | cases_sum | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Singapore | SG | SGP | 5638676.0 | 0 |
1 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Greece | EL | GRC | 10727668.0 | 0 |
2 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Lithuania | LT | LTU | 2789533.0 | 0 |
3 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Canada | CA | CAN | 37058856.0 | 0 |
4 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Brazil | BR | BRA | 209469333.0 | 0 |
df_total_cases = df[["dateRep", "cases", "countryterritoryCode", "day", "month", "year", "cases_sum"]]
df_total_cases = df_total_cases.groupby(by="countryterritoryCode").agg(sum).reset_index()
df_total_cases.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
countryterritoryCode | cases | day | month | year | cases_sum | |
---|---|---|---|---|---|---|
0 | ABW | 28 | 135 | 18 | 12120 | 1717979 |
1 | AFG | 75 | 1257 | 152 | 157559 | 13812224 |
2 | AGO | 3 | 147 | 18 | 12120 | 1937816 |
3 | ALB | 174 | 342 | 57 | 38380 | 5076230 |
4 | AND | 224 | 275 | 42 | 28280 | 2936660 |
import matplotlib
dates = matplotlib.dates.date2num(df["dateRep"])
df["datesRepStr"] = df.dateRep.astype(str)
df.head()
.dataframe tbody tr th {
vertical-align: top;
}
.dataframe thead th {
text-align: right;
}
dateRep | day | month | year | cases | deaths | countriesAndTerritories | geoId | countryterritoryCode | popData2018 | cases_sum | datesRepInt | datesRepStr | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Singapore | SG | SGP | 5638676.0 | 0 | 737424.0 | 2019-12-31 |
1 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Greece | EL | GRC | 10727668.0 | 0 | 737424.0 | 2019-12-31 |
2 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Lithuania | LT | LTU | 2789533.0 | 0 | 737424.0 | 2019-12-31 |
3 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Canada | CA | CAN | 37058856.0 | 0 | 737424.0 | 2019-12-31 |
4 | 2019-12-31 | 31 | 12 | 2019 | 0 | 0 | Brazil | BR | BRA | 209469333.0 | 0 | 737424.0 | 2019-12-31 |
fig = px.scatter_geo(df,
animation_frame="datesRepStr",
animation_group="countryterritoryCode",
locations="countryterritoryCode",
locationmode="ISO-3",
size="cases_sum")
fig.update_layout(
title={
"text": "Number of Covid Cases over Time",
"x": 0.5,
"xanchor":'center'}
)
fig.show()
<div id="d61a1113-b0b4-4576-91d6-5d6bef2b5a30" class="plotly-graph-div" style="height:600px; width:100%;"></div>
<script type="text/javascript">
require(["plotly"], function(Plotly) {
window.PLOTLYENV=window.PLOTLYENV || {};
if (document.getElementById("d61a1113-b0b4-4576-91d6-5d6bef2b5a30")) {
Plotly.newPlot(
'd61a1113-b0b4-4576-91d6-5d6bef2b5a30',
[{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2019-12-31<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SGP", "GRC", "LTU", "CAN", "BRA", "IDN", "MYS", "RUS", "OMN", "BLR", "NOR", "FRA", "AZE", "JPN", "LUX", "AUS", "CHE", null, "EGY", "ISR", "AFG", "KWT", "FIN", "HRV", "ESP", "PHL", "LKA", "GEO", "DNK", "KHM", "DZA", "ITA", "LBN", "BHR", "THA", "NPL", "IRN", "ECU", "DEU", "DOM", "SWE", "USA", "ARM", "NGA", "IRQ", "NZL", "GBR", "CZE", "MEX", "PAK", "ISL", "SMR", "MKD", "QAT", "IND", "MCO", "EST", "NLD", "IRL", "CHN", "ARE", "KOR", "ROU", "VNM", "AUT", "BEL", "TWN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SGP", "GRC", "LTU", "CAN", "BRA", "IDN", "MYS", "RUS", "OMN", "BLR", "NOR", "FRA", "AZE", "JPN", "LUX", "AUS", "CHE", null, "EGY", "ISR", "AFG", "KWT", "FIN", "HRV", "ESP", "PHL", "LKA", "GEO", "DNK", "KHM", "DZA", "ITA", "LBN", "BHR", "THA", "NPL", "IRN", "ECU", "DEU", "DOM", "SWE", "USA", "ARM", "NGA", "IRQ", "NZL", "GBR", "CZE", "MEX", "PAK", "ISL", "SMR", "MKD", "QAT", "IND", "MCO", "EST", "NLD", "IRL", "CHN", "ARE", "KOR", "ROU", "VNM", "AUT", "BEL", "TWN"], "marker": {"color": "#636efa", "size": [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 27, 27, 27, 27, 27, 27, 27, 27], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}],
{"geo": {"center": {}, "domain": {"x": [0.0, 0.98], "y": [0.0, 1.0]}}, "height": 600, "legend": {"itemsizing": "constant", "tracegroupgap": 0}, "margin": {"t": 60}, "sliders": [{"active": 0, "currentvalue": {"prefix": "datesRepStr="}, "len": 0.9, "pad": {"b": 10, "t": 60}, "steps": [{"args": [["2019-12-31"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2019-12-31", "method": "animate"}, {"args": [["2020-01-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-01", "method": "animate"}, {"args": [["2020-01-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-02", "method": "animate"}, {"args": [["2020-01-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-03", "method": "animate"}, {"args": [["2020-01-13"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-13", "method": "animate"}, {"args": [["2020-01-14"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-14", "method": "animate"}, {"args": [["2020-01-15"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-15", "method": "animate"}, {"args": [["2020-01-16"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-16", "method": "animate"}, {"args": [["2020-01-17"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-17", "method": "animate"}, {"args": [["2020-01-18"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-18", "method": "animate"}, {"args": [["2020-01-19"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-19", "method": "animate"}, {"args": [["2020-01-20"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-20", "method": "animate"}, {"args": [["2020-01-21"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-21", "method": "animate"}, {"args": [["2020-01-22"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-22", "method": "animate"}, {"args": [["2020-01-23"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-23", "method": "animate"}, {"args": [["2020-01-24"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-24", "method": "animate"}, {"args": [["2020-01-25"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-25", "method": "animate"}, {"args": [["2020-01-26"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-26", "method": "animate"}, {"args": [["2020-01-27"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-27", "method": "animate"}, {"args": [["2020-01-28"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-28", "method": "animate"}, {"args": [["2020-01-29"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-29", "method": "animate"}, {"args": [["2020-01-30"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-30", "method": "animate"}, {"args": [["2020-01-31"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-01-31", "method": "animate"}, {"args": [["2020-02-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-01", "method": "animate"}, {"args": [["2020-02-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-02", "method": "animate"}, {"args": [["2020-02-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-03", "method": "animate"}, {"args": [["2020-02-13"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-13", "method": "animate"}, {"args": [["2020-02-14"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-14", "method": "animate"}, {"args": [["2020-02-15"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-15", "method": "animate"}, {"args": [["2020-02-16"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-16", "method": "animate"}, {"args": [["2020-02-17"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-17", "method": "animate"}, {"args": [["2020-02-18"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-18", "method": "animate"}, {"args": [["2020-02-19"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-19", "method": "animate"}, {"args": [["2020-02-20"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-20", "method": "animate"}, {"args": [["2020-02-21"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-21", "method": "animate"}, {"args": [["2020-02-22"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-22", "method": "animate"}, {"args": [["2020-02-23"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-23", "method": "animate"}, {"args": [["2020-02-24"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-24", "method": "animate"}, {"args": [["2020-02-25"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-25", "method": "animate"}, {"args": [["2020-02-26"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-26", "method": "animate"}, {"args": [["2020-02-27"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-27", "method": "animate"}, {"args": [["2020-02-28"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-28", "method": "animate"}, {"args": [["2020-02-29"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-02-29", "method": "animate"}, {"args": [["2020-03-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-01", "method": "animate"}, {"args": [["2020-03-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-02", "method": "animate"}, {"args": [["2020-03-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-03", "method": "animate"}, {"args": [["2020-03-13"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-13", "method": "animate"}, {"args": [["2020-03-14"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-14", "method": "animate"}, {"args": [["2020-03-15"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-15", "method": "animate"}, {"args": [["2020-03-16"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-16", "method": "animate"}, {"args": [["2020-03-17"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-17", "method": "animate"}, {"args": [["2020-03-18"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-18", "method": "animate"}, {"args": [["2020-03-19"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-19", "method": "animate"}, {"args": [["2020-03-20"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-20", "method": "animate"}, {"args": [["2020-03-21"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-21", "method": "animate"}, {"args": [["2020-03-22"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-22", "method": "animate"}, {"args": [["2020-03-23"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-23", "method": "animate"}, {"args": [["2020-03-24"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-24", "method": "animate"}, {"args": [["2020-03-25"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-25", "method": "animate"}, {"args": [["2020-03-26"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-26", "method": "animate"}, {"args": [["2020-03-27"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-03-27", "method": "animate"}, {"args": [["2020-04-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-04-01", "method": "animate"}, {"args": [["2020-04-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-04-02", "method": "animate"}, {"args": [["2020-04-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-04-03", "method": "animate"}, {"args": [["2020-05-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-05-01", "method": "animate"}, {"args": [["2020-05-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-05-02", "method": "animate"}, {"args": [["2020-05-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-05-03", "method": "animate"}, {"args": [["2020-06-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-06-01", "method": "animate"}, {"args": [["2020-06-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-06-02", "method": "animate"}, {"args": [["2020-06-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-06-03", "method": "animate"}, {"args": [["2020-07-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-07-01", "method": "animate"}, {"args": [["2020-07-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-07-02", "method": "animate"}, {"args": [["2020-07-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-07-03", "method": "animate"}, {"args": [["2020-08-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-08-01", "method": "animate"}, {"args": [["2020-08-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-08-02", "method": "animate"}, {"args": [["2020-08-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-08-03", "method": "animate"}, {"args": [["2020-09-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-09-01", "method": "animate"}, {"args": [["2020-09-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-09-02", "method": "animate"}, {"args": [["2020-09-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-09-03", "method": "animate"}, {"args": [["2020-10-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-10-01", "method": "animate"}, {"args": [["2020-10-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-10-02", "method": "animate"}, {"args": [["2020-10-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-10-03", "method": "animate"}, {"args": [["2020-11-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-11-01", "method": "animate"}, {"args": [["2020-11-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-11-02", "method": "animate"}, {"args": [["2020-11-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-11-03", "method": "animate"}, {"args": [["2020-12-01"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-12-01", "method": "animate"}, {"args": [["2020-12-02"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-12-02", "method": "animate"}, {"args": [["2020-12-03"], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "2020-12-03", "method": "animate"}], "x": 0.1, "xanchor": "left", "y": 0, "yanchor": "top"}], "template": {"data": {"bar": [{"error_x": {"color": "#2a3f5f"}, "error_y": {"color": "#2a3f5f"}, "marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "bar"}], "barpolar": [{"marker": {"line": {"color": "#E5ECF6", "width": 0.5}}, "type": "barpolar"}], "carpet": [{"aaxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "baxis": {"endlinecolor": "#2a3f5f", "gridcolor": "white", "linecolor": "white", "minorgridcolor": "white", "startlinecolor": "#2a3f5f"}, "type": "carpet"}], "choropleth": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "choropleth"}], "contour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "contour"}], "contourcarpet": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "contourcarpet"}], "heatmap": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmap"}], "heatmapgl": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "heatmapgl"}], "histogram": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "histogram"}], "histogram2d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2d"}], "histogram2dcontour": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "histogram2dcontour"}], "mesh3d": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "type": "mesh3d"}], "parcoords": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "parcoords"}], "scatter": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter"}], "scatter3d": [{"line": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatter3d"}], "scattercarpet": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattercarpet"}], "scattergeo": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergeo"}], "scattergl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattergl"}], "scattermapbox": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scattermapbox"}], "scatterpolar": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolar"}], "scatterpolargl": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterpolargl"}], "scatterternary": [{"marker": {"colorbar": {"outlinewidth": 0, "ticks": ""}}, "type": "scatterternary"}], "surface": [{"colorbar": {"outlinewidth": 0, "ticks": ""}, "colorscale": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "type": "surface"}], "table": [{"cells": {"fill": {"color": "#EBF0F8"}, "line": {"color": "white"}}, "header": {"fill": {"color": "#C8D4E3"}, "line": {"color": "white"}}, "type": "table"}]}, "layout": {"annotationdefaults": {"arrowcolor": "#2a3f5f", "arrowhead": 0, "arrowwidth": 1}, "colorscale": {"diverging": [[0, "#8e0152"], [0.1, "#c51b7d"], [0.2, "#de77ae"], [0.3, "#f1b6da"], [0.4, "#fde0ef"], [0.5, "#f7f7f7"], [0.6, "#e6f5d0"], [0.7, "#b8e186"], [0.8, "#7fbc41"], [0.9, "#4d9221"], [1, "#276419"]], "sequential": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]], "sequentialminus": [[0.0, "#0d0887"], [0.1111111111111111, "#46039f"], [0.2222222222222222, "#7201a8"], [0.3333333333333333, "#9c179e"], [0.4444444444444444, "#bd3786"], [0.5555555555555556, "#d8576b"], [0.6666666666666666, "#ed7953"], [0.7777777777777778, "#fb9f3a"], [0.8888888888888888, "#fdca26"], [1.0, "#f0f921"]]}, "colorway": ["#636efa", "#EF553B", "#00cc96", "#ab63fa", "#FFA15A", "#19d3f3", "#FF6692", "#B6E880", "#FF97FF", "#FECB52"], "font": {"color": "#2a3f5f"}, "geo": {"bgcolor": "white", "lakecolor": "white", "landcolor": "#E5ECF6", "showlakes": true, "showland": true, "subunitcolor": "white"}, "hoverlabel": {"align": "left"}, "hovermode": "closest", "mapbox": {"style": "light"}, "paper_bgcolor": "white", "plot_bgcolor": "#E5ECF6", "polar": {"angularaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "radialaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "scene": {"xaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "yaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}, "zaxis": {"backgroundcolor": "#E5ECF6", "gridcolor": "white", "gridwidth": 2, "linecolor": "white", "showbackground": true, "ticks": "", "zerolinecolor": "white"}}, "shapedefaults": {"line": {"color": "#2a3f5f"}}, "ternary": {"aaxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "baxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}, "bgcolor": "#E5ECF6", "caxis": {"gridcolor": "white", "linecolor": "white", "ticks": ""}}, "title": {"x": 0.05}, "xaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}, "yaxis": {"automargin": true, "gridcolor": "white", "linecolor": "white", "ticks": "", "zerolinecolor": "white", "zerolinewidth": 2}}}, "title": {"text": "Number of Covid Cases over Time", "x": 0.5, "xanchor": "center"}, "updatemenus": [{"buttons": [{"args": [null, {"frame": {"duration": 500, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 500, "easing": "linear"}}], "label": "▶", "method": "animate"}, {"args": [[null], {"frame": {"duration": 0, "redraw": true}, "fromcurrent": true, "mode": "immediate", "transition": {"duration": 0, "easing": "linear"}}], "label": "◼", "method": "animate"}], "direction": "left", "pad": {"r": 10, "t": 70}, "showactive": false, "type": "buttons", "x": 0.1, "xanchor": "right", "y": 0, "yanchor": "top"}]},
{"responsive": true}
).then(function(){
Plotly.addFrames('d61a1113-b0b4-4576-91d6-5d6bef2b5a30', [{"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2019-12-31<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SGP", "GRC", "LTU", "CAN", "BRA", "IDN", "MYS", "RUS", "OMN", "BLR", "NOR", "FRA", "AZE", "JPN", "LUX", "AUS", "CHE", null, "EGY", "ISR", "AFG", "KWT", "FIN", "HRV", "ESP", "PHL", "LKA", "GEO", "DNK", "KHM", "DZA", "ITA", "LBN", "BHR", "THA", "NPL", "IRN", "ECU", "DEU", "DOM", "SWE", "USA", "ARM", "NGA", "IRQ", "NZL", "GBR", "CZE", "MEX", "PAK", "ISL", "SMR", "MKD", "QAT", "IND", "MCO", "EST", "NLD", "IRL", "CHN", "ARE", "KOR", "ROU", "VNM", "AUT", "BEL", "TWN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SGP", "GRC", "LTU", "CAN", "BRA", "IDN", "MYS", "RUS", "OMN", "BLR", "NOR", "FRA", "AZE", "JPN", "LUX", "AUS", "CHE", null, "EGY", "ISR", "AFG", "KWT", "FIN", "HRV", "ESP", "PHL", "LKA", "GEO", "DNK", "KHM", "DZA", "ITA", "LBN", "BHR", "THA", "NPL", "IRN", "ECU", "DEU", "DOM", "SWE", "USA", "ARM", "NGA", "IRQ", "NZL", "GBR", "CZE", "MEX", "PAK", "ISL", "SMR", "MKD", "QAT", "IND", "MCO", "EST", "NLD", "IRL", "CHN", "ARE", "KOR", "ROU", "VNM", "AUT", "BEL", "TWN"], "marker": {"color": "#636efa", "size": [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 27, 27, 27, 27, 27, 27, 27, 27], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2019-12-31"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["PHL", "MCO", "FRA", "SGP", "VNM", "CAN", "GRC", "RUS", "ITA", "LTU", "IDN", "DEU", "DZA", "BRA", "CHN", "QAT", "LBN", "ARE", "NPL", "PAK", "IRN", "AUT", "DOM", "TWN", "SWE", "BHR", "USA", "THA", "ECU", "ARM", "NGA", "IRQ", "EST", "NZL", "IND", "MEX", "CZE", "GBR", "ROU", "LKA", "OMN", "MKD", "KHM", "BEL", "IRL", "SMR", "AUS", "KWT", null, "JPN", "CHE", "HRV", "NLD", "MYS", "AZE", "EGY", "LUX", "ESP", "NOR", "FIN", "ISR", "KOR", "GEO", "BLR", "AFG", "DNK", "ISL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["PHL", "MCO", "FRA", "SGP", "VNM", "CAN", "GRC", "RUS", "ITA", "LTU", "IDN", "DEU", "DZA", "BRA", "CHN", "QAT", "LBN", "ARE", "NPL", "PAK", "IRN", "AUT", "DOM", "TWN", "SWE", "BHR", "USA", "THA", "ECU", "ARM", "NGA", "IRQ", "EST", "NZL", "IND", "MEX", "CZE", "GBR", "ROU", "LKA", "OMN", "MKD", "KHM", "BEL", "IRL", "SMR", "AUS", "KWT", null, "JPN", "CHE", "HRV", "NLD", "MYS", "AZE", "EGY", "LUX", "ESP", "NOR", "FIN", "ISR", "KOR", "GEO", "BLR", "AFG", "DNK", "ISL"], "marker": {"color": "#636efa", "size": [27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27, 27], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MEX", "HRV", "ISL", "CAN", "KHM", "ECU", "LBN", "MYS", "NZL", "FIN", null, "USA", "IRQ", "ARM", "ARE", "ITA", "KOR", "NOR", "GRC", "GEO", "AZE", "DZA", "CHN", "SMR", "DNK", "MKD", "IDN", "IRN", "BHR", "NGA", "MCO", "RUS", "GBR", "CHE", "TWN", "ISR", "LKA", "FRA", "BEL", "LUX", "EGY", "PHL", "OMN", "AUT", "IRL", "THA", "AUS", "PAK", "QAT", "ROU", "BLR", "SGP", "ESP", "VNM", "BRA", "LTU", "AFG", "DEU", "KWT", "DOM", "EST", "NPL", "IND", "CZE", "SWE", "JPN", "NLD"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MEX", "HRV", "ISL", "CAN", "KHM", "ECU", "LBN", "MYS", "NZL", "FIN", null, "USA", "IRQ", "ARM", "ARE", "ITA", "KOR", "NOR", "GRC", "GEO", "AZE", "DZA", "CHN", "SMR", "DNK", "MKD", "IDN", "IRN", "BHR", "NGA", "MCO", "RUS", "GBR", "CHE", "TWN", "ISR", "LKA", "FRA", "BEL", "LUX", "EGY", "PHL", "OMN", "AUT", "IRL", "THA", "AUS", "PAK", "QAT", "ROU", "BLR", "SGP", "ESP", "VNM", "BRA", "LTU", "AFG", "DEU", "KWT", "DOM", "EST", "NPL", "IND", "CZE", "SWE", "JPN", "NLD"], "marker": {"color": "#636efa", "size": [27, 27, 27, 28, 28, 28, 28, 28, 28, 28, 28, 29, 29, 29, 29, 29, 34, 34, 34, 34, 34, 34, 2129, 2129, 2129, 2129, 2129, 2129, 2129, 2129, 2129, 2131, 2131, 2131, 2132, 2132, 2132, 2132, 2132, 2132, 2132, 2132, 2132, 2132, 2132, 2137, 2139, 2139, 2139, 2139, 2139, 2142, 2143, 2143, 2143, 2143, 2143, 2145, 2145, 2145, 2145, 2145, 2145, 2145, 2146, 2147, 2147], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["PHL", "DEU", "NOR", "MCO", "DNK", "NPL", "IDN", "AUT", "GBR", "LKA", "KOR", "HRV", "CHN", "ISL", "OMN", "ARE", "PAK", "MKD", "GRC", null, "IND", "LBN", "ESP", "AUS", "BHR", "IRN", "KWT", "JPN", "AZE", "CAN", "SGP", "USA", "SWE", "CZE", "ROU", "ARM", "ITA", "QAT", "THA", "BLR", "LTU", "RUS", "FRA", "KHM", "GEO", "SMR", "TWN", "DZA", "MEX", "NZL", "CHE", "ECU", "EST", "IRQ", "IRL", "DOM", "MYS", "NLD", "FIN", "EGY", "AFG", "VNM", "ISR", "LUX", "BRA", "NGA", "BEL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["PHL", "DEU", "NOR", "MCO", "DNK", "NPL", "IDN", "AUT", "GBR", "LKA", "KOR", "HRV", "CHN", "ISL", "OMN", "ARE", "PAK", "MKD", "GRC", null, "IND", "LBN", "ESP", "AUS", "BHR", "IRN", "KWT", "JPN", "AZE", "CAN", "SGP", "USA", "SWE", "CZE", "ROU", "ARM", "ITA", "QAT", "THA", "BLR", "LTU", "RUS", "FRA", "KHM", "GEO", "SMR", "TWN", "DZA", "MEX", "NZL", "CHE", "ECU", "EST", "IRQ", "IRL", "DOM", "MYS", "NLD", "FIN", "EGY", "AFG", "VNM", "ISR", "LUX", "BRA", "NGA", "BEL"], "marker": {"color": "#636efa", "size": [2147, 2201, 2210, 2210, 2211, 2211, 2211, 2214, 2219, 2219, 2814, 2814, 3388, 3388, 3388, 3390, 3392, 3392, 3395, 3395, 3395, 3396, 3428, 3429, 3432, 3637, 3637, 3646, 3646, 3650, 3654, 3657, 3658, 3658, 3658, 3659, 3899, 3900, 3900, 3900, 3900, 3900, 3943, 3943, 3944, 3944, 3945, 3945, 3947, 3947, 3953, 3954, 3954, 3960, 3961, 3961, 3961, 3966, 3966, 3966, 3966, 3966, 3966, 3967, 3968, 3968, 3968], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-13<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["AZE", "KWT", "NGA", "AFG", "IRQ", "BLR", "GRC", "IRL", "CAN", "HRV", "CHE", "EGY", "SGP", "JPN", "RUS", "BRA", "LTU", "LUX", "AUS", "MYS", "FRA", "NOR", "PHL", "LKA", null, "NZL", "IDN", "GBR", "CZE", "ECU", "MEX", "USA", "GEO", "ISR", "BHR", "ROU", "CHN", "DEU", "NLD", "MCO", "NPL", "THA", "MKD", "EST", "DZA", "TWN", "KHM", "VNM", "BEL", "IND", "ARE", "DNK", "SMR", "ITA", "AUT", "LBN", "OMN", "ISL", "KOR", "IRN", "SWE", "DOM", "PAK", "FIN", "ARM", "ESP", "QAT"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["AZE", "KWT", "NGA", "AFG", "IRQ", "BLR", "GRC", "IRL", "CAN", "HRV", "CHE", "EGY", "SGP", "JPN", "RUS", "BRA", "LTU", "LUX", "AUS", "MYS", "FRA", "NOR", "PHL", "LKA", null, "NZL", "IDN", "GBR", "CZE", "ECU", "MEX", "USA", "GEO", "ISR", "BHR", "ROU", "CHN", "DEU", "NLD", "MCO", "NPL", "THA", "MKD", "EST", "DZA", "TWN", "KHM", "VNM", "BEL", "IND", "ARE", "DNK", "SMR", "ITA", "AUT", "LBN", "OMN", "ISL", "KOR", "IRN", "SWE", "DOM", "PAK", "FIN", "ARM", "ESP", "QAT"], "marker": {"color": "#636efa", "size": [3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3968, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-13"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-14<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["VNM", "ESP", "LUX", "KWT", "BEL", "ARE", "ISR", "IND", "JPN", "NLD", "EGY", "OMN", "MCO", "AFG", null, "RUS", "AUS", "TWN", "EST", "HRV", "KOR", "FIN", "IRL", "CHN", "ROU", "MYS", "NOR", "MKD", "QAT", "AZE", "AUT", "IDN", "ISL", "CHE", "BLR", "GBR", "MEX", "LKA", "PHL", "THA", "PAK", "DOM", "NPL", "IRQ", "DEU", "ARM", "CZE", "BHR", "ECU", "GRC", "LBN", "NZL", "DZA", "CAN", "KHM", "SWE", "LTU", "GEO", "FRA", "NGA", "DNK", "IRN", "BRA", "SMR", "SGP", "ITA", "USA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["VNM", "ESP", "LUX", "KWT", "BEL", "ARE", "ISR", "IND", "JPN", "NLD", "EGY", "OMN", "MCO", "AFG", null, "RUS", "AUS", "TWN", "EST", "HRV", "KOR", "FIN", "IRL", "CHN", "ROU", "MYS", "NOR", "MKD", "QAT", "AZE", "AUT", "IDN", "ISL", "CHE", "BLR", "GBR", "MEX", "LKA", "PHL", "THA", "PAK", "DOM", "NPL", "IRQ", "DEU", "ARM", "CZE", "BHR", "ECU", "GRC", "LBN", "NZL", "DZA", "CAN", "KHM", "SWE", "LTU", "GEO", "FRA", "NGA", "DNK", "IRN", "BRA", "SMR", "SGP", "ITA", "USA"], "marker": {"color": "#636efa", "size": [3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-14"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-15<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["VNM", "THA", "ISR", "KOR", "DOM", "SWE", "IRN", "QAT", "FIN", "ISL", "PAK", "EGY", "MEX", "GEO", "BEL", "IND", "ARE", "SMR", "MCO", "DNK", "ITA", "TWN", "KHM", "DZA", "MKD", "ROU", "DEU", "CHN", "NPL", "BHR", "EST", "AUT", "ESP", "LBN", "BLR", "LTU", "IRL", "IDN", "AUS", "ECU", "HRV", "ARM", "CZE", "RUS", "CHE", "MYS", "GBR", "PHL", "NOR", "LKA", "CAN", "LUX", null, "NZL", "USA", "GRC", "FRA", "OMN", "SGP", "BRA", "JPN", "NLD", "IRQ", "AZE", "AFG", "KWT", "NGA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["VNM", "THA", "ISR", "KOR", "DOM", "SWE", "IRN", "QAT", "FIN", "ISL", "PAK", "EGY", "MEX", "GEO", "BEL", "IND", "ARE", "SMR", "MCO", "DNK", "ITA", "TWN", "KHM", "DZA", "MKD", "ROU", "DEU", "CHN", "NPL", "BHR", "EST", "AUT", "ESP", "LBN", "BLR", "LTU", "IRL", "IDN", "AUS", "ECU", "HRV", "ARM", "CZE", "RUS", "CHE", "MYS", "GBR", "PHL", "NOR", "LKA", "CAN", "LUX", null, "NZL", "USA", "GRC", "FRA", "OMN", "SGP", "BRA", "JPN", "NLD", "IRQ", "AZE", "AFG", "KWT", "NGA"], "marker": {"color": "#636efa", "size": [3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3969, 3970, 3970, 3970, 3970, 3970, 3970, 3970], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-15"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-16<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["JPN", null, "IND", "BLR", "ITA", "KHM", "NZL", "BRA", "NPL", "CHE", "IRN", "NGA", "IRQ", "BHR", "ESP", "ISR", "AZE", "NLD", "MKD", "SWE", "THA", "CAN", "IDN", "TWN", "IRL", "LKA", "MCO", "BEL", "HRV", "GBR", "ROU", "EST", "EGY", "DEU", "FIN", "ISL", "USA", "LUX", "SGP", "DOM", "KWT", "DNK", "GEO", "OMN", "SMR", "VNM", "RUS", "DZA", "AUS", "NOR", "CZE", "KOR", "MYS", "GRC", "AFG", "QAT", "LTU", "AUT", "FRA", "PAK", "MEX", "ARE", "ECU", "CHN", "PHL", "ARM", "LBN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["JPN", null, "IND", "BLR", "ITA", "KHM", "NZL", "BRA", "NPL", "CHE", "IRN", "NGA", "IRQ", "BHR", "ESP", "ISR", "AZE", "NLD", "MKD", "SWE", "THA", "CAN", "IDN", "TWN", "IRL", "LKA", "MCO", "BEL", "HRV", "GBR", "ROU", "EST", "EGY", "DEU", "FIN", "ISL", "USA", "LUX", "SGP", "DOM", "KWT", "DNK", "GEO", "OMN", "SMR", "VNM", "RUS", "DZA", "AUS", "NOR", "CZE", "KOR", "MYS", "GRC", "AFG", "QAT", "LTU", "AUT", "FRA", "PAK", "MEX", "ARE", "ECU", "CHN", "PHL", "ARM", "LBN"], "marker": {"color": "#636efa", "size": [3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-16"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-17<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MYS", "JPN", "PAK", "OMN", "NLD", "SMR", "LBN", "NGA", "KHM", null, "AUS", "ITA", "ARM", "AFG", "RUS", "GBR", "MEX", "CZE", "NPL", "NOR", "CAN", "GEO", "BHR", "LKA", "ISR", "FIN", "SGP", "QAT", "GRC", "DEU", "IRL", "DNK", "IRN", "MCO", "KWT", "LUX", "CHN", "NZL", "ISL", "AUT", "KOR", "TWN", "LTU", "IRQ", "EST", "BRA", "ECU", "PHL", "ROU", "ESP", "DOM", "THA", "SWE", "HRV", "USA", "DZA", "BLR", "IDN", "VNM", "IND", "EGY", "BEL", "AZE", "CHE", "ARE", "FRA", "MKD"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MYS", "JPN", "PAK", "OMN", "NLD", "SMR", "LBN", "NGA", "KHM", null, "AUS", "ITA", "ARM", "AFG", "RUS", "GBR", "MEX", "CZE", "NPL", "NOR", "CAN", "GEO", "BHR", "LKA", "ISR", "FIN", "SGP", "QAT", "GRC", "DEU", "IRL", "DNK", "IRN", "MCO", "KWT", "LUX", "CHN", "NZL", "ISL", "AUT", "KOR", "TWN", "LTU", "IRQ", "EST", "BRA", "ECU", "PHL", "ROU", "ESP", "DOM", "THA", "SWE", "HRV", "USA", "DZA", "BLR", "IDN", "VNM", "IND", "EGY", "BEL", "AZE", "CHE", "ARE", "FRA", "MKD"], "marker": {"color": "#636efa", "size": [3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3970, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3974, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-17"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-18<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ARM", "FRA", "EGY", "LTU", "NZL", "NGA", "BHR", "IRQ", "BRA", "MYS", "KHM", "DZA", "SMR", "ITA", "GEO", "CHE", "SWE", "DOM", "DNK", "THA", "RUS", "LUX", "IRL", "DEU", "USA", "PHL", "NPL", "MEX", "ECU", "IRN", "QAT", "BLR", "MKD", "LKA", "AZE", "KOR", "FIN", "EST", "ESP", "JPN", "OMN", "ISL", "KWT", "VNM", "TWN", null, "ISR", "AUS", "NOR", "IDN", "HRV", "ROU", "AFG", "SGP", "AUT", "CZE", "GBR", "BEL", "IND", "PAK", "NLD", "LBN", "CAN", "ARE", "CHN", "MCO", "GRC"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ARM", "FRA", "EGY", "LTU", "NZL", "NGA", "BHR", "IRQ", "BRA", "MYS", "KHM", "DZA", "SMR", "ITA", "GEO", "CHE", "SWE", "DOM", "DNK", "THA", "RUS", "LUX", "IRL", "DEU", "USA", "PHL", "NPL", "MEX", "ECU", "IRN", "QAT", "BLR", "MKD", "LKA", "AZE", "KOR", "FIN", "EST", "ESP", "JPN", "OMN", "ISL", "KWT", "VNM", "TWN", null, "ISR", "AUS", "NOR", "IDN", "HRV", "ROU", "AFG", "SGP", "AUT", "CZE", "GBR", "BEL", "IND", "PAK", "NLD", "LBN", "CAN", "ARE", "CHN", "MCO", "GRC"], "marker": {"color": "#636efa", "size": [3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3975, 3992, 3992, 3992], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-18"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-19<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SMR", "ISL", "ROU", "ARE", "TWN", "CHE", "IDN", "EGY", "AZE", "SWE", "FRA", "BEL", "LUX", "KWT", "EST", "IND", "IRQ", "MCO", "ESP", "PHL", "KOR", "AUT", "BRA", "THA", "MKD", "ECU", "LTU", "NZL", "DZA", "NLD", "ISR", "DNK", "GBR", "LKA", "DEU", "SGP", "AFG", "MEX", "CZE", "NPL", "PAK", "CAN", "BHR", "RUS", "NGA", "GRC", "BLR", "ARM", "QAT", "LBN", "CHN", "FIN", "USA", "JPN", "IRL", "MYS", null, "KHM", "GEO", "AUS", "DOM", "ITA", "IRN", "NOR", "HRV", "OMN", "VNM"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SMR", "ISL", "ROU", "ARE", "TWN", "CHE", "IDN", "EGY", "AZE", "SWE", "FRA", "BEL", "LUX", "KWT", "EST", "IND", "IRQ", "MCO", "ESP", "PHL", "KOR", "AUT", "BRA", "THA", "MKD", "ECU", "LTU", "NZL", "DZA", "NLD", "ISR", "DNK", "GBR", "LKA", "DEU", "SGP", "AFG", "MEX", "CZE", "NPL", "PAK", "CAN", "BHR", "RUS", "NGA", "GRC", "BLR", "ARM", "QAT", "LBN", "CHN", "FIN", "USA", "JPN", "IRL", "MYS", null, "KHM", "GEO", "AUS", "DOM", "ITA", "IRN", "NOR", "HRV", "OMN", "VNM"], "marker": {"color": "#636efa", "size": [3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 3992, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-19"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-20<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["TWN", "EGY", "QAT", "NLD", "MYS", "EST", "BEL", "ROU", "IRL", "AFG", "VNM", "FIN", "CHE", "ISR", "LUX", "RUS", "BRA", "CHN", "GRC", "KWT", "DEU", "MEX", "BHR", "ISL", "ARM", "NGA", "NPL", "IRN", "IDN", "LBN", "AZE", "BLR", "CAN", "PAK", "CZE", "NOR", "SGP", "LKA", "HRV", "GBR", "JPN", "OMN", "AUS", "ITA", "DNK", "ESP", "ARE", "FRA", "NZL", "LTU", "THA", "ECU", "PHL", "MCO", "AUT", "KOR", "IRQ", "MKD", "IND", null, "DOM", "KHM", "USA", "SMR", "SWE", "DZA", "GEO"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["TWN", "EGY", "QAT", "NLD", "MYS", "EST", "BEL", "ROU", "IRL", "AFG", "VNM", "FIN", "CHE", "ISR", "LUX", "RUS", "BRA", "CHN", "GRC", "KWT", "DEU", "MEX", "BHR", "ISL", "ARM", "NGA", "NPL", "IRN", "IDN", "LBN", "AZE", "BLR", "CAN", "PAK", "CZE", "NOR", "SGP", "LKA", "HRV", "GBR", "JPN", "OMN", "AUS", "ITA", "DNK", "ESP", "ARE", "FRA", "NZL", "LTU", "THA", "ECU", "PHL", "MCO", "AUT", "KOR", "IRQ", "MKD", "IND", null, "DOM", "KHM", "USA", "SMR", "SWE", "DZA", "GEO"], "marker": {"color": "#636efa", "size": [4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4128, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4147, 4148, 4148, 4148, 4148, 4148, 4148, 4148, 4148, 4148, 4148, 4148, 4148], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-20"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-21<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["KOR", "ESP", "IND", "NOR", "ROU", "TWN", "EST", "IDN", "MKD", "HRV", "CHN", "EGY", "OMN", "FRA", "ECU", "BRA", "PHL", "NZL", "CHE", "IRQ", "GEO", "SMR", "SWE", "DOM", "IRL", "DEU", "DNK", "NGA", "RUS", "QAT", "IRN", "GRC", "CAN", "PAK", "CZE", "SGP", "LKA", "FIN", null, "ISL", "KHM", "THA", "GBR", "ITA", "ISR", "USA", "DZA", "AUS", "AZE", "JPN", "LTU", "VNM", "BEL", "BLR", "LUX", "MYS", "AFG", "KWT", "MCO", "BHR", "NLD", "AUT", "NPL", "LBN", "ARM", "MEX", "ARE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["KOR", "ESP", "IND", "NOR", "ROU", "TWN", "EST", "IDN", "MKD", "HRV", "CHN", "EGY", "OMN", "FRA", "ECU", "BRA", "PHL", "NZL", "CHE", "IRQ", "GEO", "SMR", "SWE", "DOM", "IRL", "DEU", "DNK", "NGA", "RUS", "QAT", "IRN", "GRC", "CAN", "PAK", "CZE", "SGP", "LKA", "FIN", null, "ISL", "KHM", "THA", "GBR", "ITA", "ISR", "USA", "DZA", "AUS", "AZE", "JPN", "LTU", "VNM", "BEL", "BLR", "LUX", "MYS", "AFG", "KWT", "MCO", "BHR", "NLD", "AUT", "NPL", "LBN", "ARM", "MEX", "ARE"], "marker": {"color": "#636efa", "size": [4148, 4148, 4148, 4148, 4148, 4149, 4149, 4149, 4149, 4149, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4300, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-21"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-22<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NGA", "ARM", "FRA", "NOR", "EGY", "CZE", "BLR", "PAK", "ESP", "LUX", "LTU", "CAN", "CHE", "RUS", "NZL", "GRC", "MKD", "ISL", "QAT", "LBN", "KWT", "IRL", "BRA", "THA", "ECU", "BHR", "DEU", "JPN", "SGP", "NPL", "GBR", "IRN", "ITA", "KHM", null, "OMN", "SWE", "HRV", "MEX", "USA", "DNK", "LKA", "NLD", "SMR", "AUS", "GEO", "AZE", "IDN", "PHL", "IRQ", "DOM", "DZA", "BEL", "KOR", "IND", "ISR", "MYS", "AUT", "VNM", "FIN", "EST", "AFG", "CHN", "ROU", "ARE", "MCO", "TWN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NGA", "ARM", "FRA", "NOR", "EGY", "CZE", "BLR", "PAK", "ESP", "LUX", "LTU", "CAN", "CHE", "RUS", "NZL", "GRC", "MKD", "ISL", "QAT", "LBN", "KWT", "IRL", "BRA", "THA", "ECU", "BHR", "DEU", "JPN", "SGP", "NPL", "GBR", "IRN", "ITA", "KHM", null, "OMN", "SWE", "HRV", "MEX", "USA", "DNK", "LKA", "NLD", "SMR", "AUS", "GEO", "AZE", "IDN", "PHL", "IRQ", "DOM", "DZA", "BEL", "KOR", "IND", "ISR", "MYS", "AUT", "VNM", "FIN", "EST", "AFG", "CHN", "ROU", "ARE", "MCO", "TWN"], "marker": {"color": "#636efa", "size": [4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4301, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4303, 4443, 4443, 4443, 4443, 4443], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-22"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-23<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NOR", "FRA", "MYS", "BRA", "LTU", "PHL", "MEX", "IND", "DNK", "ITA", "THA", "AZE", "LUX", "ARE", "IDN", "ESP", "HRV", "SGP", "BHR", "ISR", "JPN", "CHN", "NPL", "GBR", "ECU", "EGY", "IRN", "LKA", "IRQ", "NGA", "BLR", "FIN", "TWN", "EST", "SWE", "CHE", "AFG", "SMR", null, "OMN", "MCO", "VNM", "DZA", "IRL", "KWT", "USA", "CAN", "ROU", "GRC", "DOM", "NLD", "BEL", "LBN", "ISL", "PAK", "QAT", "AUS", "KOR", "RUS", "GEO", "CZE", "AUT", "MKD", "DEU", "NZL", "KHM", "ARM"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NOR", "FRA", "MYS", "BRA", "LTU", "PHL", "MEX", "IND", "DNK", "ITA", "THA", "AZE", "LUX", "ARE", "IDN", "ESP", "HRV", "SGP", "BHR", "ISR", "JPN", "CHN", "NPL", "GBR", "ECU", "EGY", "IRN", "LKA", "IRQ", "NGA", "BLR", "FIN", "TWN", "EST", "SWE", "CHE", "AFG", "SMR", null, "OMN", "MCO", "VNM", "DZA", "IRL", "KWT", "USA", "CAN", "ROU", "GRC", "DOM", "NLD", "BEL", "LBN", "ISL", "PAK", "QAT", "AUS", "KOR", "RUS", "GEO", "CZE", "AUT", "MKD", "DEU", "NZL", "KHM", "ARM"], "marker": {"color": "#636efa", "size": [4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4443, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540, 4540], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-23"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-24<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ITA", "SMR", "SGP", "GEO", "DEU", "LBN", "SWE", "ARE", "DOM", "THA", "MYS", "KHM", "EST", "OMN", "ECU", "IND", "IRQ", "USA", "AUS", "DZA", "GBR", "CHN", "PHL", "IDN", "AZE", null, "ROU", "NOR", "LKA", "JPN", "MEX", "BRA", "IRL", "DNK", "LUX", "GRC", "VNM", "ISL", "CHE", "KOR", "RUS", "QAT", "CZE", "MKD", "ARM", "NZL", "AFG", "MCO", "BLR", "ESP", "AUT", "NGA", "NLD", "BHR", "CAN", "PAK", "TWN", "FRA", "KWT", "LTU", "HRV", "EGY", "NPL", "IRN", "FIN", "ISR", "BEL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ITA", "SMR", "SGP", "GEO", "DEU", "LBN", "SWE", "ARE", "DOM", "THA", "MYS", "KHM", "EST", "OMN", "ECU", "IND", "IRQ", "USA", "AUS", "DZA", "GBR", "CHN", "PHL", "IDN", "AZE", null, "ROU", "NOR", "LKA", "JPN", "MEX", "BRA", "IRL", "DNK", "LUX", "GRC", "VNM", "ISL", "CHE", "KOR", "RUS", "QAT", "CZE", "MKD", "ARM", "NZL", "AFG", "MCO", "BLR", "ESP", "AUT", "NGA", "NLD", "BHR", "CAN", "PAK", "TWN", "FRA", "KWT", "LTU", "HRV", "EGY", "NPL", "IRN", "FIN", "ISR", "BEL"], "marker": {"color": "#636efa", "size": [4540, 4540, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4543, 4802, 4802, 4802, 4802, 4802, 4802, 4802, 4802, 4803, 4803, 4803, 4803, 4803, 4803, 4803, 4805, 4805, 4805, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806, 4806], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-24"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-25<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["KHM", "GBR", "SWE", "CHN", "AUT", "CAN", "AUS", "GRC", "BEL", "TWN", "ARM", "NGA", "DEU", "PAK", "BHR", "LKA", "MEX", "ISR", "USA", "IRN", "NPL", "JPN", "DNK", "CZE", "SGP", "ITA", null, "ROU", "VNM", "LBN", "DOM", "NZL", "MKD", "IRQ", "AZE", "IDN", "RUS", "CHE", "ECU", "LUX", "EST", "MCO", "LTU", "BRA", "THA", "ARE", "ESP", "FRA", "HRV", "FIN", "BLR", "EGY", "PHL", "AFG", "NOR", "DZA", "ISL", "IRL", "GEO", "MYS", "IND", "QAT", "OMN", "NLD", "KOR", "KWT", "SMR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["KHM", "GBR", "SWE", "CHN", "AUT", "CAN", "AUS", "GRC", "BEL", "TWN", "ARM", "NGA", "DEU", "PAK", "BHR", "LKA", "MEX", "ISR", "USA", "IRN", "NPL", "JPN", "DNK", "CZE", "SGP", "ITA", null, "ROU", "VNM", "LBN", "DOM", "NZL", "MKD", "IRQ", "AZE", "IDN", "RUS", "CHE", "ECU", "LUX", "EST", "MCO", "LTU", "BRA", "THA", "ARE", "ESP", "FRA", "HRV", "FIN", "BLR", "EGY", "PHL", "AFG", "NOR", "DZA", "ISL", "IRL", "GEO", "MYS", "IND", "QAT", "OMN", "NLD", "KOR", "KWT", "SMR"], "marker": {"color": "#636efa", "size": [4806, 4806, 4806, 5247, 5247, 5247, 5248, 5248, 5248, 5250, 5250, 5250, 5250, 5250, 5250, 5250, 5250, 5250, 5251, 5251, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5252, 5253, 5253, 5253, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5256, 5259, 5259, 5259, 5259, 5259, 5259, 5259, 5259], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-25"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-26<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LUX", "KWT", "BEL", "CZE", "MCO", "KOR", "ISR", "BLR", "VNM", "EGY", "IRN", "NLD", "ROU", "ESP", "AFG", "QAT", "GRC", "ARM", "MEX", "RUS", "BRA", "JPN", "CHE", "NPL", "ISL", "DNK", "LBN", "CAN", "IRL", "MKD", "BHR", "OMN", "TWN", "IND", "DZA", "LKA", "KHM", "PAK", "MYS", "NOR", "ECU", "PHL", "IDN", "USA", "IRQ", "GEO", "SMR", "ITA", null, "GBR", "NZL", "AUT", "FRA", "CHN", "SWE", "AUS", "DEU", "HRV", "DOM", "FIN", "THA", "NGA", "SGP", "LTU", "EST", "AZE", "ARE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX", "KWT", "BEL", "CZE", "MCO", "KOR", "ISR", "BLR", "VNM", "EGY", "IRN", "NLD", "ROU", "ESP", "AFG", "QAT", "GRC", "ARM", "MEX", "RUS", "BRA", "JPN", "CHE", "NPL", "ISL", "DNK", "LBN", "CAN", "IRL", "MKD", "BHR", "OMN", "TWN", "IND", "DZA", "LKA", "KHM", "PAK", "MYS", "NOR", "ECU", "PHL", "IDN", "USA", "IRQ", "GEO", "SMR", "ITA", null, "GBR", "NZL", "AUT", "FRA", "CHN", "SWE", "AUS", "DEU", "HRV", "DOM", "FIN", "THA", "NGA", "SGP", "LTU", "EST", "AZE", "ARE"], "marker": {"color": "#636efa", "size": [5259, 5259, 5259, 5259, 5259, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5260, 5261, 5261, 5261, 5261, 5261, 5261, 5262, 5262, 5262, 5262, 5262, 5262, 5262, 5262, 5262, 5262, 5262, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5263, 5928, 5928, 5931, 5931, 5931, 5931, 5931, 5931, 5931, 5932, 5932, 5932, 5932, 5932], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-26"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-27<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["KOR", "GBR", "AUS", "LBN", "CHN", "OMN", "CZE", "IND", "NOR", "MCO", "MKD", "HRV", "SGP", "AZE", "NPL", "ESP", "ISL", "IDN", "LKA", "PAK", null, "ARE", "KWT", "JPN", "AUT", "FRA", "BHR", "IRL", "NGA", "QAT", "DEU", "RUS", "CHE", "PHL", "BRA", "LUX", "BLR", "NLD", "EGY", "ITA", "KHM", "CAN", "THA", "LTU", "DOM", "ECU", "SWE", "DZA", "IRQ", "SMR", "USA", "VNM", "GEO", "NZL", "EST", "FIN", "MYS", "BEL", "ROU", "GRC", "TWN", "ISR", "AFG", "ARM", "IRN", "MEX", "DNK"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["KOR", "GBR", "AUS", "LBN", "CHN", "OMN", "CZE", "IND", "NOR", "MCO", "MKD", "HRV", "SGP", "AZE", "NPL", "ESP", "ISL", "IDN", "LKA", "PAK", null, "ARE", "KWT", "JPN", "AUT", "FRA", "BHR", "IRL", "NGA", "QAT", "DEU", "RUS", "CHE", "PHL", "BRA", "LUX", "BLR", "NLD", "EGY", "ITA", "KHM", "CAN", "THA", "LTU", "DOM", "ECU", "SWE", "DZA", "IRQ", "SMR", "USA", "VNM", "GEO", "NZL", "EST", "FIN", "MYS", "BEL", "ROU", "GRC", "TWN", "ISR", "AFG", "ARM", "IRN", "MEX", "DNK"], "marker": {"color": "#636efa", "size": [5933, 5933, 5933, 5933, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6720, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6721, 6724, 6724, 6724, 6724, 6724, 6724, 6724, 6724, 6727, 6727, 6727, 6727, 6727, 6727, 6727, 6727, 6727, 6727, 6729, 6729, 6729, 6729, 6729, 6729, 6729], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-27"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-28<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["TWN", "MKD", "AZE", "HRV", "KOR", "LTU", "ROU", "MCO", "ISR", "IDN", "AUT", "BEL", "ECU", "IRQ", "GEO", "FRA", "EGY", "NOR", "MYS", "NLD", "NZL", "VNM", "AFG", "IRL", "KWT", "QAT", "GBR", "IND", "EST", "ARE", "CHE", "ISL", "ESP", "BRA", "FIN", "LUX", "THA", "BLR", "CHN", "RUS", "CAN", "DEU", "JPN", "DNK", "KHM", "IRN", "BHR", "ITA", "CZE", null, "USA", "SGP", "MEX", "NGA", "PAK", "PHL", "SMR", "DOM", "AUS", "LKA", "GRC", "NPL", "LBN", "SWE", "ARM", "OMN", "DZA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["TWN", "MKD", "AZE", "HRV", "KOR", "LTU", "ROU", "MCO", "ISR", "IDN", "AUT", "BEL", "ECU", "IRQ", "GEO", "FRA", "EGY", "NOR", "MYS", "NLD", "NZL", "VNM", "AFG", "IRL", "KWT", "QAT", "GBR", "IND", "EST", "ARE", "CHE", "ISL", "ESP", "BRA", "FIN", "LUX", "THA", "BLR", "CHN", "RUS", "CAN", "DEU", "JPN", "DNK", "KHM", "IRN", "BHR", "ITA", "CZE", null, "USA", "SGP", "MEX", "NGA", "PAK", "PHL", "SMR", "DOM", "AUS", "LKA", "GRC", "NPL", "LBN", "SWE", "ARM", "OMN", "DZA"], "marker": {"color": "#636efa", "size": [6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6731, 6737, 6737, 8490, 8490, 8491, 8492, 8493, 8493, 8494, 8494, 8494, 8494, 8494, 8494, 8494, 8495, 8495, 8495, 8495, 8495, 8495, 8495, 8495, 8496, 8496, 8496, 8496, 8496, 8496, 8496, 8496], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-28"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-29<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["AUT", "LBN", "CZE", "ROU", "NLD", "NPL", "VNM", "NGA", "IRL", "DEU", "QAT", "CAN", "BEL", "GRC", "KOR", "ISR", "CHN", "FIN", "JPN", "SGP", "ARM", "IND", "EST", "KWT", "MEX", "AFG", "MYS", "IRN", "NZL", "TWN", "BHR", "NOR", "DNK", "SMR", "BRA", "MCO", "HRV", "AZE", "MKD", "KHM", "LKA", "FRA", "DOM", "ITA", "AUS", "IDN", "SWE", "ECU", "DZA", "IRQ", "GEO", "GBR", "OMN", "LTU", "EGY", "RUS", "PAK", "ISL", "CHE", "ARE", "LUX", "ESP", "USA", null, "THA", "BLR", "PHL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["AUT", "LBN", "CZE", "ROU", "NLD", "NPL", "VNM", "NGA", "IRL", "DEU", "QAT", "CAN", "BEL", "GRC", "KOR", "ISR", "CHN", "FIN", "JPN", "SGP", "ARM", "IND", "EST", "KWT", "MEX", "AFG", "MYS", "IRN", "NZL", "TWN", "BHR", "NOR", "DNK", "SMR", "BRA", "MCO", "HRV", "AZE", "MKD", "KHM", "LKA", "FRA", "DOM", "ITA", "AUS", "IDN", "SWE", "ECU", "DZA", "IRQ", "GEO", "GBR", "OMN", "LTU", "EGY", "RUS", "PAK", "ISL", "CHE", "ARE", "LUX", "ESP", "USA", null, "THA", "BLR", "PHL"], "marker": {"color": "#636efa", "size": [8496, 8496, 8496, 8496, 8496, 8496, 8496, 8496, 8496, 8499, 8499, 8500, 8500, 8500, 8500, 8500, 9966, 9966, 9969, 9971, 9971, 9971, 9971, 9971, 9971, 9971, 9974, 9974, 9974, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9975, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976, 9976], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-29"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-30<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GBR", "SGP", "LTU", "RUS", "ECU", "MYS", "NOR", "LBN", "QAT", "GEO", "MEX", "PHL", "OMN", "SMR", "ROU", "DZA", "DEU", "AUT", "FIN", "KOR", "EGY", "LUX", "CZE", "MCO", "HRV", "AFG", "VNM", "EST", "DOM", "IND", "FRA", "DNK", "ARM", "USA", "AUS", "PAK", "GRC", "KWT", "ISL", "IRL", "AZE", "BLR", "NZL", "NPL", "CAN", "ESP", "JPN", "NLD", "IRQ", "THA", "ARE", "BRA", "IRN", "BHR", "CHE", "MKD", "NGA", "SWE", "CHN", null, "BEL", "ISR", "TWN", "IDN", "ITA", "KHM", "LKA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GBR", "SGP", "LTU", "RUS", "ECU", "MYS", "NOR", "LBN", "QAT", "GEO", "MEX", "PHL", "OMN", "SMR", "ROU", "DZA", "DEU", "AUT", "FIN", "KOR", "EGY", "LUX", "CZE", "MCO", "HRV", "AFG", "VNM", "EST", "DOM", "IND", "FRA", "DNK", "ARM", "USA", "AUS", "PAK", "GRC", "KWT", "ISL", "IRL", "AZE", "BLR", "NZL", "NPL", "CAN", "ESP", "JPN", "NLD", "IRQ", "THA", "ARE", "BRA", "IRN", "BHR", "CHE", "MKD", "NGA", "SWE", "CHN", null, "BEL", "ISR", "TWN", "IDN", "ITA", "KHM", "LKA"], "marker": {"color": "#636efa", "size": [9976, 9979, 9979, 9979, 9979, 9979, 9979, 9979, 9979, 9979, 9979, 9980, 9980, 9980, 9980, 9980, 9980, 9980, 9981, 9981, 9981, 9981, 9981, 9981, 9981, 9981, 9981, 9981, 9981, 9982, 9983, 9983, 9983, 9983, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9985, 9989, 9989, 9989, 9989, 9992, 9992, 9992, 9992, 9992, 9992, 9992, 9992, 11732, 11732, 11732, 11732, 11732, 11732, 11732, 11732, 11732], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-30"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-01-31<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ESP", "DOM", "MEX", "SWE", "PHL", "ITA", "NOR", "ARE", "DZA", "NGA", "SMR", "DEU", "USA", "KHM", "BHR", "OMN", "AUS", null, "PAK", "LKA", "MCO", "IDN", "CZE", "MKD", "ISL", "HRV", "JPN", "SGP", "NPL", "LBN", "CAN", "GRC", "ARM", "DNK", "IRN", "AZE", "IRQ", "GBR", "AUT", "EGY", "IND", "CHE", "MYS", "THA", "BRA", "FIN", "LUX", "RUS", "AFG", "VNM", "QAT", "IRL", "GEO", "NLD", "NZL", "EST", "TWN", "KWT", "KOR", "FRA", "CHN", "LTU", "ECU", "ROU", "ISR", "BLR", "BEL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ESP", "DOM", "MEX", "SWE", "PHL", "ITA", "NOR", "ARE", "DZA", "NGA", "SMR", "DEU", "USA", "KHM", "BHR", "OMN", "AUS", null, "PAK", "LKA", "MCO", "IDN", "CZE", "MKD", "ISL", "HRV", "JPN", "SGP", "NPL", "LBN", "CAN", "GRC", "ARM", "DNK", "IRN", "AZE", "IRQ", "GBR", "AUT", "EGY", "IND", "CHE", "MYS", "THA", "BRA", "FIN", "LUX", "RUS", "AFG", "VNM", "QAT", "IRL", "GEO", "NLD", "NZL", "EST", "TWN", "KWT", "KOR", "FRA", "CHN", "LTU", "ECU", "ROU", "ISR", "BLR", "BEL"], "marker": {"color": "#636efa", "size": [11732, 11732, 11732, 11732, 11732, 11735, 11735, 11735, 11735, 11735, 11735, 11736, 11737, 11737, 11737, 11737, 11738, 11738, 11738, 11738, 11738, 11738, 11738, 11738, 11738, 11738, 11741, 11744, 11744, 11744, 11744, 11744, 11744, 11744, 11744, 11744, 11744, 11746, 11746, 11746, 11746, 11746, 11747, 11747, 11747, 11747, 11747, 11747, 11747, 11750, 11750, 11750, 11750, 11750, 11750, 11750, 11751, 11751, 11754, 11755, 13735, 13735, 13735, 13735, 13735, 13735, 13735], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-01-31"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["IRL", "NOR", "QAT", "CAN", "CZE", null, "GBR", "GRC", "OMN", "AZE", "TWN", "ROU", "NLD", "EST", "IDN", "SGP", "BEL", "MEX", "VNM", "JPN", "SWE", "NGA", "LKA", "USA", "ECU", "DOM", "THA", "IRQ", "PHL", "HRV", "NZL", "DZA", "FRA", "LTU", "SMR", "KHM", "GEO", "ITA", "DNK", "BRA", "ARM", "FIN", "CHE", "PAK", "DEU", "BLR", "ISR", "LBN", "IRN", "NPL", "EGY", "LUX", "MYS", "BHR", "AFG", "ARE", "RUS", "ISL", "IND", "MCO", "CHN", "KWT", "ESP", "MKD", "KOR", "AUS", "AUT"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["IRL", "NOR", "QAT", "CAN", "CZE", null, "GBR", "GRC", "OMN", "AZE", "TWN", "ROU", "NLD", "EST", "IDN", "SGP", "BEL", "MEX", "VNM", "JPN", "SWE", "NGA", "LKA", "USA", "ECU", "DOM", "THA", "IRQ", "PHL", "HRV", "NZL", "DZA", "FRA", "LTU", "SMR", "KHM", "GEO", "ITA", "DNK", "BRA", "ARM", "FIN", "CHE", "PAK", "DEU", "BLR", "ISR", "LBN", "IRN", "NPL", "EGY", "LUX", "MYS", "BHR", "AFG", "ARE", "RUS", "ISL", "IND", "MCO", "CHN", "KWT", "ESP", "MKD", "KOR", "AUS", "AUT"], "marker": {"color": "#636efa", "size": [13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735, 13735], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LUX", "PAK", "IRL", "MEX", "DEU", "MKD", "QAT", "ROU", "CHN", "LBN", "BHR", "SMR", "KOR", "LTU", "RUS", "AFG", "EGY", "DNK", "NPL", "IRN", null, "SGP", "LKA", "MCO", "SWE", "CHE", "IDN", "ARM", "FIN", "IRQ", "EST", "DOM", "ISR", "OMN", "BLR", "AUT", "CZE", "NGA", "JPN", "ESP", "NZL", "HRV", "CAN", "IND", "ITA", "THA", "GRC", "ECU", "ARE", "FRA", "KWT", "PHL", "USA", "AUS", "MYS", "BEL", "NOR", "GEO", "AZE", "DZA", "KHM", "BRA", "GBR", "TWN", "NLD", "ISL", "VNM"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX", "PAK", "IRL", "MEX", "DEU", "MKD", "QAT", "ROU", "CHN", "LBN", "BHR", "SMR", "KOR", "LTU", "RUS", "AFG", "EGY", "DNK", "NPL", "IRN", null, "SGP", "LKA", "MCO", "SWE", "CHE", "IDN", "ARM", "FIN", "IRQ", "EST", "DOM", "ISR", "OMN", "BLR", "AUT", "CZE", "NGA", "JPN", "ESP", "NZL", "HRV", "CAN", "IND", "ITA", "THA", "GRC", "ECU", "ARE", "FRA", "KWT", "PHL", "USA", "AUS", "MYS", "BEL", "NOR", "GEO", "AZE", "DZA", "KHM", "BRA", "GBR", "TWN", "NLD", "ISL", "VNM"], "marker": {"color": "#636efa", "size": [13735, 13735, 13735, 13735, 13736, 13736, 13736, 13736, 16326, 16326, 16326, 16326, 16329, 16329, 16329, 16329, 16329, 16329, 16329, 16329, 16329, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16331, 16335, 16335, 16335, 16335, 16335, 16336, 16336, 16336, 16336, 16336, 16337, 16337, 16337, 16338, 16339, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16341, 16343], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LBN", "ISR", "AUS", "IDN", "IRN", "SGP", "EGY", "DNK", "KWT", "USA", "THA", "QAT", "IRL", "AZE", "ESP", "CHE", "BHR", "GRC", "DOM", "LTU", "DEU", "NGA", "ECU", null, "SMR", "NZL", "FIN", "MKD", "PAK", "CHN", "ITA", "GBR", "SWE", "ROU", "ARM", "BRA", "NPL", "ISL", "HRV", "KOR", "BEL", "EST", "TWN", "OMN", "NLD", "KHM", "NOR", "IRQ", "LKA", "CAN", "FRA", "RUS", "MYS", "AFG", "MCO", "VNM", "GEO", "MEX", "AUT", "DZA", "PHL", "LUX", "IND", "ARE", "CZE", "JPN", "BLR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LBN", "ISR", "AUS", "IDN", "IRN", "SGP", "EGY", "DNK", "KWT", "USA", "THA", "QAT", "IRL", "AZE", "ESP", "CHE", "BHR", "GRC", "DOM", "LTU", "DEU", "NGA", "ECU", null, "SMR", "NZL", "FIN", "MKD", "PAK", "CHN", "ITA", "GBR", "SWE", "ROU", "ARM", "BRA", "NPL", "ISL", "HRV", "KOR", "BEL", "EST", "TWN", "OMN", "NLD", "KHM", "NOR", "IRQ", "LKA", "CAN", "FRA", "RUS", "MYS", "AFG", "MCO", "VNM", "GEO", "MEX", "AUT", "DZA", "PHL", "LUX", "IND", "ARE", "CZE", "JPN", "BLR"], "marker": {"color": "#636efa", "size": [16349, 16352, 16355, 16357, 16742, 16746, 16747, 16748, 16749, 16769, 16770, 16772, 16772, 16774, 16791, 16797, 16803, 16803, 16804, 16804, 16822, 16822, 16827, 16827, 16834, 16834, 16837, 16837, 16837, 17042, 17603, 17616, 17617, 17617, 17617, 17617, 17617, 17619, 17621, 18307, 18308, 18308, 18308, 18308, 18314, 18314, 18318, 18324, 18324, 18328, 18358, 18358, 18362, 18362, 18362, 18362, 18362, 18363, 18367, 18369, 18369, 18369, 18369, 18369, 18372, 18387, 18387], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-13<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ECU", "BLR", "ARM", "CAN", "GRC", "GEO", "MEX", "LTU", "KOR", "FIN", "AFG", "DEU", "BEL", "ISR", "DNK", "AZE", "BHR", "TWN", "IRN", "DOM", "CZE", "VNM", "NLD", "KWT", null, "BRA", "ITA", "MCO", "AUS", "PAK", "QAT", "AUT", "NPL", "RUS", "EST", "ROU", "LKA", "IRQ", "KHM", "OMN", "IND", "CHN", "NZL", "GBR", "SMR", "THA", "SWE", "MKD", "PHL", "IRL", "ESP", "HRV", "LUX", "DZA", "IDN", "EGY", "JPN", "ARE", "ISL", "FRA", "NGA", "USA", "NOR", "SGP", "MYS", "LBN", "CHE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ECU", "BLR", "ARM", "CAN", "GRC", "GEO", "MEX", "LTU", "KOR", "FIN", "AFG", "DEU", "BEL", "ISR", "DNK", "AZE", "BHR", "TWN", "IRN", "DOM", "CZE", "VNM", "NLD", "KWT", null, "BRA", "ITA", "MCO", "AUS", "PAK", "QAT", "AUT", "NPL", "RUS", "EST", "ROU", "LKA", "IRQ", "KHM", "OMN", "IND", "CHN", "NZL", "GBR", "SMR", "THA", "SWE", "MKD", "PHL", "IRL", "ESP", "HRV", "LUX", "DZA", "IDN", "EGY", "JPN", "ARE", "ISL", "FRA", "NGA", "USA", "NOR", "SGP", "MYS", "LBN", "CHE"], "marker": {"color": "#636efa", "size": [18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18387, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 18388, 33529, 33529, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33530, 33534, 33534, 33534, 33534, 33534, 33535, 33535, 33538, 33538, 33538, 33538], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-13"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-14<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NOR", "PHL", "ITA", "TWN", "MEX", "DZA", "DOM", "IRQ", "ECU", "USA", "GEO", "NGA", "ESP", "SWE", "SMR", "DEU", "KHM", "AZE", "LUX", "MYS", "FRA", "SGP", "LBN", "IND", "NLD", null, "VNM", "KWT", "CZE", "THA", "BRA", "AUT", "ISL", "AUS", "NPL", "RUS", "ROU", "MKD", "LKA", "EST", "CHN", "MCO", "OMN", "NZL", "GBR", "ARE", "PAK", "QAT", "CHE", "ISR", "CAN", "ARM", "BHR", "AFG", "LTU", "GRC", "KOR", "JPN", "FIN", "IRL", "HRV", "DNK", "EGY", "BLR", "BEL", "IDN", "IRN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NOR", "PHL", "ITA", "TWN", "MEX", "DZA", "DOM", "IRQ", "ECU", "USA", "GEO", "NGA", "ESP", "SWE", "SMR", "DEU", "KHM", "AZE", "LUX", "MYS", "FRA", "SGP", "LBN", "IND", "NLD", null, "VNM", "KWT", "CZE", "THA", "BRA", "AUT", "ISL", "AUS", "NPL", "RUS", "ROU", "MKD", "LKA", "EST", "CHN", "MCO", "OMN", "NZL", "GBR", "ARE", "PAK", "QAT", "CHE", "ISR", "CAN", "ARM", "BHR", "AFG", "LTU", "GRC", "KOR", "JPN", "FIN", "IRL", "HRV", "DNK", "EGY", "BLR", "BEL", "IDN", "IRN"], "marker": {"color": "#636efa", "size": [33538, 33538, 33538, 33538, 33538, 33538, 33538, 33538, 33538, 33539, 33539, 33539, 33539, 33539, 33539, 33539, 33539, 33539, 33539, 33540, 33540, 33548, 33548, 33548, 33548, 33595, 33595, 33595, 33595, 33595, 33595, 33595, 33595, 33596, 33596, 33596, 33596, 33596, 33596, 33596, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37752, 37753, 37753, 37753, 37753, 37753, 37753, 37753, 37753, 37753, 37753], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-14"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-15<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["QAT", "TWN", "CHN", "GEO", "EST", "LTU", "AFG", "IRQ", "NZL", "BLR", "DZA", "THA", "NLD", "RUS", "NOR", "EGY", "IRL", "CHE", "KOR", "AUT", "ITA", "BEL", "SMR", "LUX", "FRA", "VNM", "ECU", "ISR", "BRA", "IND", "MCO", "DOM", "ARM", "USA", "MEX", "FIN", "PHL", "OMN", "LBN", "DEU", "ROU", "PAK", "MYS", "SGP", "ESP", "ISL", "LKA", "JPN", "DNK", "GBR", "CAN", "GRC", "NPL", "BHR", "KWT", "IRN", "HRV", "IDN", "SWE", "AUS", "KHM", "CZE", "ARE", "NGA", "MKD", null, "AZE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["QAT", "TWN", "CHN", "GEO", "EST", "LTU", "AFG", "IRQ", "NZL", "BLR", "DZA", "THA", "NLD", "RUS", "NOR", "EGY", "IRL", "CHE", "KOR", "AUT", "ITA", "BEL", "SMR", "LUX", "FRA", "VNM", "ECU", "ISR", "BRA", "IND", "MCO", "DOM", "ARM", "USA", "MEX", "FIN", "PHL", "OMN", "LBN", "DEU", "ROU", "PAK", "MYS", "SGP", "ESP", "ISL", "LKA", "JPN", "DNK", "GBR", "CAN", "GRC", "NPL", "BHR", "KWT", "IRN", "HRV", "IDN", "SWE", "AUS", "KHM", "CZE", "ARE", "NGA", "MKD", null, "AZE"], "marker": {"color": "#636efa", "size": [37753, 37753, 40291, 40291, 40291, 40291, 40291, 40291, 40291, 40291, 40291, 40292, 40292, 40292, 40292, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40293, 40295, 40304, 40304, 40304, 40304, 40312, 40312, 40312, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313, 40313], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-15"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-16<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NOR", "SGP", "LTU", "IDN", "ARE", "MYS", "THA", "PAK", "DOM", "ISL", "QAT", "ARM", "CAN", "BEL", "DNK", "FRA", "AZE", "IRL", "CZE", "IRN", "AUT", "RUS", "GBR", "IRQ", "LUX", "JPN", "NGA", "ISR", "BHR", "EST", "HRV", "GRC", "EGY", "MCO", "KHM", "NPL", "BLR", "AFG", "LKA", "PHL", "ECU", "FIN", "NLD", "KOR", "ITA", "MKD", "USA", "BRA", "SWE", null, "MEX", "ESP", "NZL", "SMR", "AUS", "OMN", "KWT", "IND", "GEO", "VNM", "CHE", "ROU", "DZA", "LBN", "TWN", "CHN", "DEU"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NOR", "SGP", "LTU", "IDN", "ARE", "MYS", "THA", "PAK", "DOM", "ISL", "QAT", "ARM", "CAN", "BEL", "DNK", "FRA", "AZE", "IRL", "CZE", "IRN", "AUT", "RUS", "GBR", "IRQ", "LUX", "JPN", "NGA", "ISR", "BHR", "EST", "HRV", "GRC", "EGY", "MCO", "KHM", "NPL", "BLR", "AFG", "LKA", "PHL", "ECU", "FIN", "NLD", "KOR", "ITA", "MKD", "USA", "BRA", "SWE", null, "MEX", "ESP", "NZL", "SMR", "AUS", "OMN", "KWT", "IND", "GEO", "VNM", "CHE", "ROU", "DZA", "LBN", "TWN", "CHN", "DEU"], "marker": {"color": "#636efa", "size": [40313, 40318, 40318, 40318, 40318, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40319, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40333, 40334, 40334, 40334, 40334, 40334, 40334, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 40468, 42475, 42475], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-16"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-17<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CHE", "FRA", "NOR", "BRA", null, "BHR", "AUT", "SGP", "MKD", "AUS", "FIN", "GBR", "EGY", "NZL", "ISL", "JPN", "QAT", "PAK", "LBN", "MCO", "VNM", "EST", "IRN", "NGA", "DEU", "DZA", "IND", "PHL", "ECU", "OMN", "USA", "ITA", "KOR", "SWE", "SMR", "DOM", "ESP", "MEX", "NLD", "BEL", "TWN", "AZE", "ARE", "KHM", "IRQ", "ROU", "CHN", "GEO", "RUS", "DNK", "LKA", "CZE", "ARM", "NPL", "HRV", "KWT", "LUX", "CAN", "MYS", "LTU", "THA", "IDN", "AFG", "ISR", "IRL", "BLR", "GRC"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CHE", "FRA", "NOR", "BRA", null, "BHR", "AUT", "SGP", "MKD", "AUS", "FIN", "GBR", "EGY", "NZL", "ISL", "JPN", "QAT", "PAK", "LBN", "MCO", "VNM", "EST", "IRN", "NGA", "DEU", "DZA", "IND", "PHL", "ECU", "OMN", "USA", "ITA", "KOR", "SWE", "SMR", "DOM", "ESP", "MEX", "NLD", "BEL", "TWN", "AZE", "ARE", "KHM", "IRQ", "ROU", "CHN", "GEO", "RUS", "DNK", "LKA", "CZE", "ARM", "NPL", "HRV", "KWT", "LUX", "CAN", "MYS", "LTU", "THA", "IDN", "AFG", "ISR", "IRL", "BLR", "GRC"], "marker": {"color": "#636efa", "size": [42475, 42476, 42476, 42476, 42476, 42476, 42476, 42479, 42479, 42479, 42479, 42479, 42479, 42479, 42479, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42486, 42487, 42487, 42487, 42487, 42487, 42487, 42487, 42487, 42489, 42489, 42490, 42490, 42490, 42490, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-17"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-18<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["BEL", "LKA", "ARE", "FRA", "BRA", "PAK", "ROU", "IND", "AUS", "NPL", "OMN", "THA", "GBR", "RUS", "MCO", "IRQ", "MYS", "CAN", "GEO", "LTU", "IDN", "BLR", "AFG", "USA", "ITA", "SWE", "DZA", "DEU", "CZE", "PHL", "ECU", "DOM", "EST", "NLD", "ESP", "TWN", "MEX", "NGA", "KOR", "AZE", "KHM", "SMR", "ARM", "IRN", "HRV", "CHN", "VNM", "NZL", "KWT", null, "MKD", "FIN", "LBN", "ISL", "DNK", "CHE", "NOR", "QAT", "AUT", "GRC", "LUX", "IRL", "SGP", "BHR", "EGY", "JPN", "ISR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["BEL", "LKA", "ARE", "FRA", "BRA", "PAK", "ROU", "IND", "AUS", "NPL", "OMN", "THA", "GBR", "RUS", "MCO", "IRQ", "MYS", "CAN", "GEO", "LTU", "IDN", "BLR", "AFG", "USA", "ITA", "SWE", "DZA", "DEU", "CZE", "PHL", "ECU", "DOM", "EST", "NLD", "ESP", "TWN", "MEX", "NGA", "KOR", "AZE", "KHM", "SMR", "ARM", "IRN", "HRV", "CHN", "VNM", "NZL", "KWT", null, "MKD", "FIN", "LBN", "ISL", "DNK", "CHE", "NOR", "QAT", "AUT", "GRC", "LUX", "IRL", "SGP", "BHR", "EGY", "JPN", "ISR"], "marker": {"color": "#636efa", "size": [44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44542, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44543, 44545, 44545, 44545, 44546, 44546, 44546, 44546, 44546, 44546, 44546, 46436, 46436, 46436, 46436, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46535, 46537, 46537, 46537, 46537, 46537], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-18"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-19<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["FIN", "AFG", "ISR", "NZL", "TWN", "ESP", "MYS", "AUT", "BEL", "USA", "ARM", "CAN", "JPN", "IDN", "SWE", "HRV", "DEU", "DOM", "ROU", "EST", "KOR", "IRQ", "NLD", "PHL", "DNK", "GRC", "IRN", "OMN", "ARE", "LKA", "NPL", "PAK", "GBR", "AUS", "CZE", "MCO", null, "MKD", "LBN", "ISL", "SGP", "BHR", "NGA", "MEX", "KHM", "QAT", "NOR", "CHE", "CHN", "VNM", "EGY", "FRA", "IRL", "LUX", "BRA", "KWT", "GEO", "AZE", "THA", "LTU", "ECU", "RUS", "DZA", "BLR", "IND", "SMR", "ITA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["FIN", "AFG", "ISR", "NZL", "TWN", "ESP", "MYS", "AUT", "BEL", "USA", "ARM", "CAN", "JPN", "IDN", "SWE", "HRV", "DEU", "DOM", "ROU", "EST", "KOR", "IRQ", "NLD", "PHL", "DNK", "GRC", "IRN", "OMN", "ARE", "LKA", "NPL", "PAK", "GBR", "AUS", "CZE", "MCO", null, "MKD", "LBN", "ISL", "SGP", "BHR", "NGA", "MEX", "KHM", "QAT", "NOR", "CHE", "CHN", "VNM", "EGY", "FRA", "IRL", "LUX", "BRA", "KWT", "GEO", "AZE", "THA", "LTU", "ECU", "RUS", "DZA", "BLR", "IND", "SMR", "ITA"], "marker": {"color": "#636efa", "size": [46537, 46537, 46537, 46537, 46537, 46537, 46537, 46537, 46537, 46537, 46537, 46537, 46544, 46544, 46544, 46544, 46544, 46544, 46544, 46544, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46559, 46647, 46647, 46647, 46647, 46651, 46651, 46651, 46651, 46651, 46651, 46651, 46651, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-19"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-20<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NZL", "FIN", "MCO", "ISL", "NPL", "FRA", "PAK", "IND", "OMN", "MKD", "NGA", "KWT", "NLD", "IRQ", "ITA", "LTU", "DEU", "IDN", "GEO", "LBN", "JPN", "LUX", "QAT", "GRC", "ISR", "MEX", "IRN", "NOR", "IRL", "PHL", "MYS", "GBR", "ESP", "ARE", "SMR", "DNK", "CHE", "BEL", "TWN", "BRA", "AFG", "CAN", "SWE", "VNM", "KHM", "RUS", "AZE", "DOM", "HRV", "EST", "AUS", "KOR", "AUT", "THA", "CHN", "ECU", "BHR", "USA", "DZA", "CZE", "LKA", "ROU", "EGY", "BLR", "ARM", null, "SGP"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NZL", "FIN", "MCO", "ISL", "NPL", "FRA", "PAK", "IND", "OMN", "MKD", "NGA", "KWT", "NLD", "IRQ", "ITA", "LTU", "DEU", "IDN", "GEO", "LBN", "JPN", "LUX", "QAT", "GRC", "ISR", "MEX", "IRN", "NOR", "IRL", "PHL", "MYS", "GBR", "ESP", "ARE", "SMR", "DNK", "CHE", "BEL", "TWN", "BRA", "AFG", "CAN", "SWE", "VNM", "KHM", "RUS", "AZE", "DOM", "HRV", "EST", "AUS", "KOR", "AUT", "THA", "CHN", "ECU", "BHR", "USA", "DZA", "CZE", "LKA", "ROU", "EGY", "BLR", "ARM", null, "SGP"], "marker": {"color": "#636efa", "size": [48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48401, 48419, 48419, 48419, 48419, 48419, 48419, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48421, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48423, 48457, 48457, 48457, 48851, 48851, 48851, 48851, 48851, 48851, 48851, 48851, 48851, 48851, 48851, 48930, 48933], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-20"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-21<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LUX", "LBN", "NZL", "IRQ", "PHL", "MYS", "DEU", "IND", "MCO", "AUS", "LTU", "DZA", "NGA", "KOR", "NOR", "DOM", "ARM", "RUS", "MKD", null, "ESP", "FRA", "GEO", "SMR", "ECU", "IDN", "IRL", "LKA", "USA", "BRA", "QAT", "CHN", "EGY", "MEX", "CHE", "GBR", "ROU", "GRC", "ARE", "IRN", "BLR", "AZE", "KHM", "VNM", "CAN", "ITA", "AFG", "CZE", "DNK", "OMN", "SWE", "SGP", "THA", "NPL", "BEL", "ISL", "FIN", "TWN", "PAK", "NLD", "JPN", "KWT", "AUT", "ISR", "BHR", "EST", "HRV"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX", "LBN", "NZL", "IRQ", "PHL", "MYS", "DEU", "IND", "MCO", "AUS", "LTU", "DZA", "NGA", "KOR", "NOR", "DOM", "ARM", "RUS", "MKD", null, "ESP", "FRA", "GEO", "SMR", "ECU", "IDN", "IRL", "LKA", "USA", "BRA", "QAT", "CHN", "EGY", "MEX", "CHE", "GBR", "ROU", "GRC", "ARE", "IRN", "BLR", "AZE", "KHM", "VNM", "CAN", "ITA", "AFG", "CZE", "DNK", "OMN", "SWE", "SGP", "THA", "NPL", "BEL", "ISL", "FIN", "TWN", "PAK", "NLD", "JPN", "KWT", "AUT", "ISR", "BHR", "EST", "HRV"], "marker": {"color": "#636efa", "size": [48933, 48933, 48933, 48933, 48933, 48933, 48933, 48933, 48933, 48935, 48935, 48935, 48935, 49010, 49010, 49010, 49010, 49010, 49010, 49023, 49023, 49023, 49023, 49023, 49023, 49023, 49023, 49023, 49024, 49024, 49024, 49915, 49915, 49915, 49915, 49915, 49915, 49915, 49915, 49918, 49918, 49918, 49918, 49918, 49919, 49919, 49919, 49919, 49919, 49919, 49919, 49920, 49920, 49920, 49920, 49920, 49920, 49920, 49920, 49920, 49929, 49929, 49929, 49929, 49929, 49929, 49929], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-21"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-22<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ARE", "JPN", "SGP", "CHN", "NLD", "OMN", "USA", "BLR", "BHR", "IDN", "MEX", "CZE", "ARM", "PAK", "LTU", "ITA", "AUT", "ESP", "DZA", "KOR", "HRV", "AUS", "ISR", "MCO", "DEU", "TWN", "NOR", "NZL", "KWT", "PHL", "LBN", "ISL", "IND", "DOM", "AZE", "BEL", "CHE", "ECU", "DNK", "GRC", "GBR", "THA", "SMR", "GEO", "IRL", "NPL", "NGA", "BRA", "EGY", "VNM", "QAT", "AFG", "LKA", "SWE", "MYS", "LUX", null, "MKD", "IRN", "FRA", "KHM", "ROU", "IRQ", "CAN", "FIN", "RUS", "EST"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ARE", "JPN", "SGP", "CHN", "NLD", "OMN", "USA", "BLR", "BHR", "IDN", "MEX", "CZE", "ARM", "PAK", "LTU", "ITA", "AUT", "ESP", "DZA", "KOR", "HRV", "AUS", "ISR", "MCO", "DEU", "TWN", "NOR", "NZL", "KWT", "PHL", "LBN", "ISL", "IND", "DOM", "AZE", "BEL", "CHE", "ECU", "DNK", "GRC", "GBR", "THA", "SMR", "GEO", "IRL", "NPL", "NGA", "BRA", "EGY", "VNM", "QAT", "AFG", "LKA", "SWE", "MYS", "LUX", null, "MKD", "IRN", "FRA", "KHM", "ROU", "IRQ", "CAN", "FIN", "RUS", "EST"], "marker": {"color": "#636efa", "size": [49931, 49943, 49944, 50770, 50770, 50770, 50789, 50789, 50789, 50789, 50789, 50789, 50789, 50789, 50789, 50803, 50803, 50803, 50803, 50993, 50993, 50997, 50998, 50998, 50998, 51000, 51000, 51000, 51000, 51000, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51001, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-22"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-23<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SMR", "LBN", "BRA", "ISL", "AZE", "USA", "EST", "LKA", "HRV", "GBR", "MYS", "KHM", "PAK", "CHE", "ISR", "CHN", null, "RUS", "DOM", "VNM", "ARE", "ESP", "BLR", "GEO", "TWN", "LUX", "AUT", "IND", "IRN", "FRA", "IRL", "MKD", "SGP", "ECU", "ARM", "KWT", "JPN", "FIN", "LTU", "DNK", "DEU", "NGA", "ITA", "CAN", "ROU", "GRC", "NOR", "IDN", "IRQ", "SWE", "MEX", "AUS", "NZL", "NPL", "PHL", "KOR", "NLD", "QAT", "BHR", "CZE", "BEL", "EGY", "THA", "MCO", "AFG", "OMN", "DZA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SMR", "LBN", "BRA", "ISL", "AZE", "USA", "EST", "LKA", "HRV", "GBR", "MYS", "KHM", "PAK", "CHE", "ISR", "CHN", null, "RUS", "DOM", "VNM", "ARE", "ESP", "BLR", "GEO", "TWN", "LUX", "AUT", "IND", "IRN", "FRA", "IRL", "MKD", "SGP", "ECU", "ARM", "KWT", "JPN", "FIN", "LTU", "DNK", "DEU", "NGA", "ITA", "CAN", "ROU", "GRC", "NOR", "IDN", "IRQ", "SWE", "MEX", "AUS", "NZL", "NPL", "PHL", "KOR", "NLD", "QAT", "BHR", "CZE", "BEL", "EGY", "THA", "MCO", "AFG", "OMN", "DZA"], "marker": {"color": "#636efa", "size": [51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51014, 51661, 51661, 51661, 51661, 51661, 51663, 51663, 51663, 51663, 51663, 51663, 51663, 51663, 51673, 51673, 51673, 51673, 51676, 51676, 51676, 51676, 51703, 51703, 51703, 51703, 51703, 51703, 51765, 51765, 51765, 51765, 51765, 51765, 51765, 51765, 51765, 51766, 51766, 51766, 51766, 52022, 52022, 52022, 52022, 52022, 52022, 52022, 52022, 52022, 52022, 52022, 52022], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-23"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-24<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["DEU", "BRA", "MYS", "NZL", "CZE", "AUT", "TWN", "DOM", "OMN", "ITA", "CAN", "NOR", "GBR", "AUS", "KOR", "IRQ", "LBN", "IND", "SWE", "LUX", "RUS", "BLR", "ARE", "KHM", "PHL", "EST", "DZA", "IRL", "MEX", "LTU", "AZE", "FIN", "USA", "LKA", "ISL", "ARM", "BHR", "DNK", "AFG", "CHE", "JPN", "BEL", "ROU", "GRC", "EGY", "CHN", null, "NGA", "IDN", "THA", "NLD", "ISR", "SGP", "PAK", "SMR", "VNM", "MKD", "MCO", "IRN", "ESP", "HRV", "KWT", "FRA", "GEO", "QAT", "NPL", "ECU"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["DEU", "BRA", "MYS", "NZL", "CZE", "AUT", "TWN", "DOM", "OMN", "ITA", "CAN", "NOR", "GBR", "AUS", "KOR", "IRQ", "LBN", "IND", "SWE", "LUX", "RUS", "BLR", "ARE", "KHM", "PHL", "EST", "DZA", "IRL", "MEX", "LTU", "AZE", "FIN", "USA", "LKA", "ISL", "ARM", "BHR", "DNK", "AFG", "CHE", "JPN", "BEL", "ROU", "GRC", "EGY", "CHN", null, "NGA", "IDN", "THA", "NLD", "ISR", "SGP", "PAK", "SMR", "VNM", "MKD", "MCO", "IRN", "ESP", "HRV", "KWT", "FRA", "GEO", "QAT", "NPL", "ECU"], "marker": {"color": "#636efa", "size": [52022, 52022, 52022, 52022, 52022, 52022, 52024, 52024, 52024, 52077, 52077, 52077, 52081, 52081, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52242, 52243, 52243, 52243, 52243, 52255, 52255, 52255, 52255, 52255, 52473, 52530, 52530, 52530, 52530, 52530, 52531, 52531, 52531, 52531, 52531, 52531, 52531, 52546, 52546, 52546, 52549, 52549, 52549, 52549, 52549, 52549], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-24"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-25<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["IRQ", "THA", "DNK", "NPL", "SWE", "RUS", "FIN", "EST", "GRC", "IRN", "VNM", "AUT", "KWT", "SGP", "ARE", "ISR", "DZA", "AZE", "GBR", "HRV", "TWN", "ISL", "KOR", "AFG", "BEL", "OMN", "MCO", "NOR", "CAN", "LBN", "MYS", "FRA", "IND", "BLR", "DOM", "ROU", "CHE", "BRA", "LUX", "IDN", "MKD", "ARM", "BHR", "ECU", "PAK", "LKA", "USA", "CHN", "SMR", null, "ESP", "IRL", "MEX", "GEO", "CZE", "EGY", "JPN", "NZL", "QAT", "DEU", "ITA", "PHL", "NGA", "NLD", "KHM", "AUS", "LTU"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["IRQ", "THA", "DNK", "NPL", "SWE", "RUS", "FIN", "EST", "GRC", "IRN", "VNM", "AUT", "KWT", "SGP", "ARE", "ISR", "DZA", "AZE", "GBR", "HRV", "TWN", "ISL", "KOR", "AFG", "BEL", "OMN", "MCO", "NOR", "CAN", "LBN", "MYS", "FRA", "IND", "BLR", "DOM", "ROU", "CHE", "BRA", "LUX", "IDN", "MKD", "ARM", "BHR", "ECU", "PAK", "LKA", "USA", "CHN", "SMR", null, "ESP", "IRL", "MEX", "GEO", "CZE", "EGY", "JPN", "NZL", "QAT", "DEU", "ITA", "PHL", "NGA", "NLD", "KHM", "AUS", "LTU"], "marker": {"color": "#636efa", "size": [52550, 52552, 52552, 52552, 52552, 52552, 52552, 52552, 52552, 52570, 52570, 52570, 52572, 52573, 52573, 52573, 52573, 52573, 52573, 52573, 52575, 52575, 52705, 52706, 52706, 52708, 52708, 52708, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52710, 52711, 52711, 52711, 52711, 52729, 53244, 53244, 53244, 53245, 53245, 53245, 53245, 53245, 53245, 53245, 53245, 53245, 53245, 53342, 53342, 53342, 53342, 53342, 53342, 53342], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-25"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-26<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["QAT", "LKA", "MEX", "USA", "CHN", "NLD", "DZA", "GEO", "ARM", "NPL", "IRL", "CAN", "EGY", "GRC", "JPN", "LTU", "FIN", "OMN", "ISL", "DNK", "AFG", "IDN", "CHE", "ROU", "THA", "SGP", "NGA", "BEL", "NZL", "FRA", "AUT", "DOM", "ARE", "RUS", "KWT", "KOR", "GBR", "BLR", "LBN", "IND", "ISR", "TWN", "PHL", "AUS", "ITA", "NOR", "MYS", "IRQ", "HRV", "EST", "SMR", "AZE", "MCO", "PAK", null, "VNM", "BRA", "ESP", "ECU", "BHR", "SWE", "MKD", "DEU", "LUX", "CZE", "IRN", "KHM"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["QAT", "LKA", "MEX", "USA", "CHN", "NLD", "DZA", "GEO", "ARM", "NPL", "IRL", "CAN", "EGY", "GRC", "JPN", "LTU", "FIN", "OMN", "ISL", "DNK", "AFG", "IDN", "CHE", "ROU", "THA", "SGP", "NGA", "BEL", "NZL", "FRA", "AUT", "DOM", "ARE", "RUS", "KWT", "KOR", "GBR", "BLR", "LBN", "IND", "ISR", "TWN", "PHL", "AUS", "ITA", "NOR", "MYS", "IRQ", "HRV", "EST", "SMR", "AZE", "MCO", "PAK", null, "VNM", "BRA", "ESP", "ECU", "BHR", "SWE", "MKD", "DEU", "LUX", "CZE", "IRN", "KHM"], "marker": {"color": "#636efa", "size": [53342, 53342, 53342, 53342, 53752, 53752, 53753, 53753, 53753, 53753, 53753, 53753, 53753, 53753, 53773, 53773, 53773, 53775, 53775, 53775, 53775, 53775, 53776, 53776, 53779, 53780, 53780, 53780, 53780, 53782, 53784, 53784, 53784, 53784, 53790, 54044, 54044, 54044, 54044, 54044, 54044, 54045, 54045, 54045, 54138, 54138, 54138, 54142, 54143, 54143, 54143, 54143, 54143, 54143, 54143, 54143, 54144, 54148, 54148, 54169, 54169, 54169, 54171, 54171, 54171, 54205, 54205], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-26"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-27<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CZE", "SWE", "PAK", "ITA", "CAN", "FIN", "MYS", "ARE", "ROU", "SGP", "BHR", "BLR", "JPN", "OMN", "TWN", "THA", "SMR", "MCO", "DEU", "CHE", "PHL", "IRL", "KHM", "NOR", "NPL", "AUS", "IND", "VNM", "LBN", "CHN", "BRA", "DOM", "USA", "NLD", "MKD", "LUX", "ESP", "ECU", null, "FRA", "ARM", "IDN", "NZL", "IRQ", "DZA", "DNK", "GRC", "LKA", "BEL", "AFG", "QAT", "ISL", "KWT", "AZE", "IRN", "RUS", "HRV", "AUT", "NGA", "KOR", "GBR", "EST", "ISR", "EGY", "MEX", "LTU", "GEO"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CZE", "SWE", "PAK", "ITA", "CAN", "FIN", "MYS", "ARE", "ROU", "SGP", "BHR", "BLR", "JPN", "OMN", "TWN", "THA", "SMR", "MCO", "DEU", "CHE", "PHL", "IRL", "KHM", "NOR", "NPL", "AUS", "IND", "VNM", "LBN", "CHN", "BRA", "DOM", "USA", "NLD", "MKD", "LUX", "ESP", "ECU", null, "FRA", "ARM", "IDN", "NZL", "IRQ", "DZA", "DNK", "GRC", "LKA", "BEL", "AFG", "QAT", "ISL", "KWT", "AZE", "IRN", "RUS", "HRV", "AUT", "NGA", "KOR", "GBR", "EST", "ISR", "EGY", "MEX", "LTU", "GEO"], "marker": {"color": "#636efa", "size": [54205, 54206, 54208, 54286, 54287, 54288, 54288, 54288, 54289, 54291, 54301, 54301, 54323, 54323, 54324, 54324, 54324, 54324, 54328, 54328, 54328, 54328, 54328, 54329, 54329, 54330, 54330, 54330, 54331, 54770, 54770, 54770, 54776, 54776, 54777, 54777, 54782, 54782, 54796, 54799, 54799, 54799, 54799, 54799, 54799, 54800, 54801, 54801, 54801, 54801, 54801, 54801, 54816, 54816, 54860, 54860, 54861, 54861, 54861, 55310, 55310, 55310, 55310, 55310, 55310, 55310, 55311], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-27"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-28<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CHN", "IRL", "NGA", "BLR", "IDN", "CHE", "LKA", "IND", "MKD", "THA", "BEL", "OMN", "ISL", "HRV", "KOR", "ISR", "CAN", "ESP", "IRN", "NZL", null, "TWN", "SWE", "ITA", "NOR", "KHM", "BRA", "IRQ", "NLD", "ARE", "MCO", "PHL", "FIN", "FRA", "CZE", "EGY", "DEU", "SGP", "DOM", "AFG", "VNM", "NPL", "BHR", "ARM", "GRC", "RUS", "USA", "LBN", "GBR", "GEO", "SMR", "KWT", "AUT", "ECU", "AZE", "DNK", "PAK", "EST", "LTU", "JPN", "AUS", "LUX", "QAT", "ROU", "MYS", "DZA", "MEX"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CHN", "IRL", "NGA", "BLR", "IDN", "CHE", "LKA", "IND", "MKD", "THA", "BEL", "OMN", "ISL", "HRV", "KOR", "ISR", "CAN", "ESP", "IRN", "NZL", null, "TWN", "SWE", "ITA", "NOR", "KHM", "BRA", "IRQ", "NLD", "ARE", "MCO", "PHL", "FIN", "FRA", "CZE", "EGY", "DEU", "SGP", "DOM", "AFG", "VNM", "NPL", "BHR", "ARM", "GRC", "RUS", "USA", "LBN", "GBR", "GEO", "SMR", "KWT", "AUT", "ECU", "AZE", "DNK", "PAK", "EST", "LTU", "JPN", "AUS", "LUX", "QAT", "ROU", "MYS", "DZA", "MEX"], "marker": {"color": "#636efa", "size": [55640, 55640, 55641, 55642, 55642, 55649, 55649, 55649, 55649, 55649, 55649, 55651, 55651, 55652, 56079, 56080, 56082, 56095, 56201, 56202, 56202, 56204, 56209, 56459, 56462, 56462, 56462, 56463, 56464, 56470, 56470, 56470, 56470, 56491, 56491, 56491, 56517, 56520, 56520, 56520, 56520, 56520, 56520, 56520, 56522, 56522, 56523, 56524, 56527, 56527, 56528, 56545, 56548, 56548, 56548, 56548, 56548, 56549, 56550, 56574, 56574, 56574, 56574, 56574, 56575, 56575, 56575], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-28"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-02-29<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["RUS", "USA", "NZL", "LUX", "AUS", "NLD", "BLR", "GRC", "QAT", "ISR", "BEL", "THA", "DNK", "NPL", "PAK", "GEO", "NOR", "ESP", "ECU", "AZE", "IND", "ROU", "HRV", "EST", "KWT", "IRQ", "SMR", "AUT", "ISL", "GBR", "CHN", "KOR", "PHL", "LBN", "BHR", "AFG", "BRA", "CHE", "KHM", "OMN", "EGY", "MCO", "DOM", "FIN", "LKA", "MKD", "DEU", "DZA", "IRL", "ITA", "TWN", "ARE", "CAN", "ARM", "LTU", null, "VNM", "MEX", "MYS", "NGA", "IDN", "JPN", "SGP", "FRA", "SWE", "IRN", "CZE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["RUS", "USA", "NZL", "LUX", "AUS", "NLD", "BLR", "GRC", "QAT", "ISR", "BEL", "THA", "DNK", "NPL", "PAK", "GEO", "NOR", "ESP", "ECU", "AZE", "IND", "ROU", "HRV", "EST", "KWT", "IRQ", "SMR", "AUT", "ISL", "GBR", "CHN", "KOR", "PHL", "LBN", "BHR", "AFG", "BRA", "CHE", "KHM", "OMN", "EGY", "MCO", "DOM", "FIN", "LKA", "MKD", "DEU", "DZA", "IRL", "ITA", "TWN", "ARE", "CAN", "ARM", "LTU", null, "VNM", "MEX", "MYS", "NGA", "IDN", "JPN", "SGP", "FRA", "SWE", "IRN", "CZE"], "marker": {"color": "#636efa", "size": [56575, 56581, 56581, 56581, 56583, 56584, 56584, 56585, 56585, 56589, 56589, 56591, 56592, 56592, 56592, 56593, 56595, 56604, 56604, 56605, 56605, 56607, 56609, 56609, 56611, 56612, 56612, 56614, 56615, 56617, 57045, 57954, 57954, 57954, 57959, 57959, 57959, 57963, 57963, 57963, 57963, 57964, 57964, 57965, 57965, 57965, 57975, 57975, 57975, 58213, 58218, 58218, 58220, 58220, 58220, 58220, 58220, 58222, 58224, 58224, 58224, 58244, 58246, 58265, 58270, 58413, 58413], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-02-29"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GEO", "ISL", "DEU", "CHN", "RUS", "LKA", "KOR", "BRA", "OMN", "MEX", "CAN", "CHE", "KWT", "SWE", "EGY", "DNK", "IDN", "FIN", "SMR", "DOM", "IRL", "GBR", "AUS", "ARM", "PAK", "QAT", null, "NGA", "AFG", "JPN", "AZE", "CZE", "BLR", "GRC", "DZA", "SGP", "NZL", "VNM", "USA", "IRQ", "THA", "ESP", "ITA", "IRN", "FRA", "BHR", "NPL", "ISR", "LBN", "KHM", "TWN", "EST", "NLD", "LTU", "MCO", "MYS", "BEL", "MKD", "ROU", "NOR", "HRV", "AUT", "ECU", "ARE", "IND", "PHL", "LUX"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GEO", "ISL", "DEU", "CHN", "RUS", "LKA", "KOR", "BRA", "OMN", "MEX", "CAN", "CHE", "KWT", "SWE", "EGY", "DNK", "IDN", "FIN", "SMR", "DOM", "IRL", "GBR", "AUS", "ARM", "PAK", "QAT", null, "NGA", "AFG", "JPN", "AZE", "CZE", "BLR", "GRC", "DZA", "SGP", "NZL", "VNM", "USA", "IRQ", "THA", "ESP", "ITA", "IRN", "FRA", "BHR", "NPL", "ISR", "LBN", "KHM", "TWN", "EST", "NLD", "LTU", "MCO", "MYS", "BEL", "MKD", "ROU", "NOR", "HRV", "AUT", "ECU", "ARE", "IND", "PHL", "LUX"], "marker": {"color": "#636efa", "size": [58413, 58413, 58413, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430, 58430], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["IRQ", "GEO", "PHL", "ECU", "LBN", "EGY", "MKD", "CHN", "ARM", "LKA", null, "FRA", "CHE", "MEX", "DOM", "LTU", "BRA", "ESP", "IRL", "SMR", "IND", "DZA", "USA", "IDN", "NZL", "EST", "HRV", "CAN", "ISR", "TWN", "BEL", "KHM", "SWE", "ARE", "AZE", "NGA", "GRC", "VNM", "AUT", "AFG", "CZE", "NLD", "THA", "BLR", "IRN", "PAK", "JPN", "OMN", "NPL", "MYS", "ROU", "ITA", "BHR", "SGP", "KWT", "FIN", "ISL", "NOR", "DEU", "AUS", "LUX", "GBR", "MCO", "RUS", "DNK", "KOR", "QAT"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["IRQ", "GEO", "PHL", "ECU", "LBN", "EGY", "MKD", "CHN", "ARM", "LKA", null, "FRA", "CHE", "MEX", "DOM", "LTU", "BRA", "ESP", "IRL", "SMR", "IND", "DZA", "USA", "IDN", "NZL", "EST", "HRV", "CAN", "ISR", "TWN", "BEL", "KHM", "SWE", "ARE", "AZE", "NGA", "GRC", "VNM", "AUT", "AFG", "CZE", "NLD", "THA", "BLR", "IRN", "PAK", "JPN", "OMN", "NPL", "MYS", "ROU", "ITA", "BHR", "SGP", "KWT", "FIN", "ISL", "NOR", "DEU", "AUS", "LUX", "GBR", "MCO", "RUS", "DNK", "KOR", "QAT"], "marker": {"color": "#636efa", "size": [58430, 58430, 58430, 58430, 58430, 58430, 58430, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61242, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61245, 61246, 61246, 61246, 61246, 61246, 61246, 61246, 61246, 61246, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61247, 61248, 61248, 61248, 61248, 61248, 61248, 61248, 61248, 61248], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SXM", "NOR", "SAU", "DEU", "NLD", "AND", "ITA", "IND", "TUN", "CZE", "JPN", "TWN", "FRA", "GBR", "CHE", "PRT", "CAN", "AUS", "LVA", "SWE", "CHN", "ECU", "HRV", "ESP", "KOR", "RUS", "AUT", "KWT", "IRN", "SGP", "ISL", "SEN", "USA", "IRQ", "MAR", "JOR", "BEL", "DNK", "OMN", "LBN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SXM", "NOR", "SAU", "DEU", "NLD", "AND", "ITA", "IND", "TUN", "CZE", "JPN", "TWN", "FRA", "GBR", "CHE", "PRT", "CAN", "AUS", "LVA", "SWE", "CHN", "ECU", "HRV", "ESP", "KOR", "RUS", "AUT", "KWT", "IRN", "SGP", "ISL", "SEN", "USA", "IRQ", "MAR", "JOR", "BEL", "DNK", "OMN", "LBN"], "marker": {"color": "#636efa", "size": [61249, 61255, 61256, 61284, 61289, 61290, 61436, 61438, 61439, 61441, 61441, 61442, 61490, 61494, 61500, 61502, 61505, 61509, 61510, 61511, 61638, 61639, 61640, 61671, 62271, 62272, 62276, 62286, 62809, 62811, 62814, 62815, 62829, 62831, 62832, 62833, 62839, 62840, 62841, 62844], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-13<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["FIN", "CAN", "VCT", "SWE", "ROU", "MKD", "DZA", "IRN", "LUX", "EGY", "ALB", "IRQ", "VNM", "KHM", "EST", "LKA", "MLT", "FRA", "MYS", "RUS", "MEX", "TUN", "CHE", "ZAF", "GRC", "KWT", "BTN", "PAN", "GHA", "AZE", "KOR", "GBR", "PRY", "PER", "HRV", "SRB", "AUT", "PHL", "CRI", "ISR", "ISL", "MAR", "SGP", "HUN", "JPN", "JAM", "TTO", "NLD", "GUY", "PAK", "SVK", "TUR", "LVA", "BLR", "UKR", "BHR", "CZE", "ARE", "SVN", "DNK", "ITA", "BEL", "CUW", "SAU", "SEN", "NOR", "CHN", "GEO", "USA", "BOL", "SMR", "ESP", "BRN", "ABW", "GAB", "ARG", "LIE", "AUS", "ARM", "BRA", "BIH", "POL", "TWN", "MDA", "CHL", "IRL", "BGR", "LBN", "DEU", "IND", "PRT"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["FIN", "CAN", "VCT", "SWE", "ROU", "MKD", "DZA", "IRN", "LUX", "EGY", "ALB", "IRQ", "VNM", "KHM", "EST", "LKA", "MLT", "FRA", "MYS", "RUS", "MEX", "TUN", "CHE", "ZAF", "GRC", "KWT", "BTN", "PAN", "GHA", "AZE", "KOR", "GBR", "PRY", "PER", "HRV", "SRB", "AUT", "PHL", "CRI", "ISR", "ISL", "MAR", "SGP", "HUN", "JPN", "JAM", "TTO", "NLD", "GUY", "PAK", "SVK", "TUR", "LVA", "BLR", "UKR", "BHR", "CZE", "ARE", "SVN", "DNK", "ITA", "BEL", "CUW", "SAU", "SEN", "NOR", "CHN", "GEO", "USA", "BOL", "SMR", "ESP", "BRN", "ABW", "GAB", "ARG", "LIE", "AUS", "ARM", "BRA", "BIH", "POL", "TWN", "MDA", "CHL", "IRL", "BGR", "LBN", "DEU", "IND", "PRT"], "marker": {"color": "#636efa", "size": [62940, 62975, 62976, 63134, 63153, 63155, 63159, 64234, 64253, 64273, 64285, 64289, 64294, 64296, 64307, 64308, 64311, 64906, 64915, 64920, 64925, 64931, 65143, 65147, 65181, 65189, 65189, 65202, 65204, 65204, 65314, 65448, 65449, 65454, 65463, 65469, 65584, 65587, 65588, 65602, 65634, 65635, 65644, 65647, 65703, 65707, 65708, 65819, 65820, 65821, 65832, 65833, 65839, 65848, 65850, 65850, 65872, 65883, 65922, 66082, 68733, 68818, 68819, 68836, 68838, 68970, 68992, 68993, 69344, 69345, 69346, 70210, 70224, 70226, 70227, 70239, 70240, 70270, 70272, 70297, 70297, 70315, 70316, 70318, 70328, 70355, 70371, 70376, 71178, 71180, 71199], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-13"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-14<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MEX", "TUN", "ARM", "JPN", "MYS", "BGR", "IRL", "NLD", "BHR", "TTO", "LIE", "IRQ", "LTU", "BRN", "BOL", "THA", "BEL", "AND", "VNM", "MDV", "ARG", "LVA", "ISR", "NZL", "CHE", "AUT", "MDA", "TWN", "GBR", "LUX", "MLT", "AUS", "ALB", "KWT", "KEN", "MAR", "USA", "DZA", "ITA", "BRA", "JAM", "PRT", "GIN", "FRA", "PAK", "CZE", "COD", "DNK", "NOR", "CYP", "ETH", "GRC", "PAN", "LKA", "PRY", "PER", "SRB", "IRN", "PSE", "MKD", "SVK", "CUB", "COL", "IND", "CRI", "HRV", "KOR", "ESP", "ZAF", "SVN", "RUS", "IDN", "CHN", "ISL", "OMN", "HUN", "CHL", "HND", "EST", "SEN", "SGP", "DOM", "SMR", "ECU", "DEU", "SWE", "CAN", "POL", "SDN", "EGY", "QAT", "SAU", "ROU", "PHL", "GEO"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MEX", "TUN", "ARM", "JPN", "MYS", "BGR", "IRL", "NLD", "BHR", "TTO", "LIE", "IRQ", "LTU", "BRN", "BOL", "THA", "BEL", "AND", "VNM", "MDV", "ARG", "LVA", "ISR", "NZL", "CHE", "AUT", "MDA", "TWN", "GBR", "LUX", "MLT", "AUS", "ALB", "KWT", "KEN", "MAR", "USA", "DZA", "ITA", "BRA", "JAM", "PRT", "GIN", "FRA", "PAK", "CZE", "COD", "DNK", "NOR", "CYP", "ETH", "GRC", "PAN", "LKA", "PRY", "PER", "SRB", "IRN", "PSE", "MKD", "SVK", "CUB", "COL", "IND", "CRI", "HRV", "KOR", "ESP", "ZAF", "SVN", "RUS", "IDN", "CHN", "ISL", "OMN", "HUN", "CHL", "HND", "EST", "SEN", "SGP", "DOM", "SMR", "ECU", "DEU", "SWE", "CAN", "POL", "SDN", "EGY", "QAT", "SAU", "ROU", "PHL", "GEO"], "marker": {"color": "#636efa", "size": [71209, 71212, 71219, 71281, 71320, 71328, 71349, 71539, 71587, 71588, 71588, 71599, 71602, 71614, 71621, 71633, 71793, 71794, 71799, 71800, 71803, 71806, 71826, 71827, 72094, 72237, 72239, 72243, 72360, 72372, 72375, 72416, 72426, 72446, 72447, 72448, 72959, 72961, 75508, 75529, 75531, 75565, 75566, 76351, 76351, 76385, 76386, 76514, 76514, 76522, 76523, 76580, 76589, 76592, 76593, 76609, 76626, 77915, 77920, 77924, 77933, 77934, 77941, 77949, 77952, 77958, 78065, 79292, 79299, 79344, 79359, 79394, 79413, 79413, 79415, 79424, 79434, 79435, 79487, 79500, 79513, 79519, 79525, 79531, 80224, 80379, 80417, 80436, 80437, 80450, 80508, 80532, 80557, 80569, 80574], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-14"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-15<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["AUS", "TTO", "ISL", "OMN", "USA", "BGD", "HUN", "CUB", "ECU", "LCA", "BEL", "TGO", "MDV", "ITA", "QAT", "SVK", "VNM", "SVN", "AUT", "THA", "COL", "LIE", "BRA", "TWN", "GAB", "IND", "BOL", "ISR", "CRI", "ARM", "SMR", "CIV", "ALB", "HRV", "KOR", "BTN", "RUS", "POL", "LTU", "ZAF", "CYP", "EST", "NZL", "GEO", "DOM", "ARG", "PSE", "MAR", "KEN", "MRT", "MNG", "ATG", "KWT", "SAU", "GRC", "PAN", "KAZ", "URY", "AZE", "LVA", "DZA", "GBR", "RWA", "PER", "SEN", "SYC", "PHL", "SRB", "GHA", "NLD", "NAM", "NPL", "MEX", "VEN", "GIN", "PRT", "JPN", "TUN", "FRA", "EGY", "GNQ", "PAK", "JOR", "GTM", "MDA", "BHR", "ARE", "COD", "LBN", "DNK", "MCO", "DEU", "SGP", "MLT", "CZE", "NOR", "CMR", "SWZ", "KHM", "CHE", "BGR", "CHL", "MYS", "LKA", "ESP", "CHN", "AFG", "IRL", "IRN", "BRN", "SWE", "CAN", "IDN", "SUR", "ETH", "BFA", "NGA", "ROU", "IRQ", "FIN", "SDN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["AUS", "TTO", "ISL", "OMN", "USA", "BGD", "HUN", "CUB", "ECU", "LCA", "BEL", "TGO", "MDV", "ITA", "QAT", "SVK", "VNM", "SVN", "AUT", "THA", "COL", "LIE", "BRA", "TWN", "GAB", "IND", "BOL", "ISR", "CRI", "ARM", "SMR", "CIV", "ALB", "HRV", "KOR", "BTN", "RUS", "POL", "LTU", "ZAF", "CYP", "EST", "NZL", "GEO", "DOM", "ARG", "PSE", "MAR", "KEN", "MRT", "MNG", "ATG", "KWT", "SAU", "GRC", "PAN", "KAZ", "URY", "AZE", "LVA", "DZA", "GBR", "RWA", "PER", "SEN", "SYC", "PHL", "SRB", "GHA", "NLD", "NAM", "NPL", "MEX", "VEN", "GIN", "PRT", "JPN", "TUN", "FRA", "EGY", "GNQ", "PAK", "JOR", "GTM", "MDA", "BHR", "ARE", "COD", "LBN", "DNK", "MCO", "DEU", "SGP", "MLT", "CZE", "NOR", "CMR", "SWZ", "KHM", "CHE", "BGR", "CHL", "MYS", "LKA", "ESP", "CHN", "AFG", "IRL", "IRN", "BRN", "SWE", "CAN", "IDN", "SUR", "ETH", "BFA", "NGA", "ROU", "IRQ", "FIN", "SDN"], "marker": {"color": "#636efa", "size": [80626, 80626, 80647, 80647, 81424, 81424, 81430, 81430, 81435, 81437, 81567, 81567, 81567, 81657, 81674, 81688, 81692, 81732, 81883, 81883, 81901, 81901, 81924, 81930, 81930, 81937, 81937, 81999, 82000, 82007, 82026, 82029, 82034, 82040, 82116, 82116, 82130, 82166, 82169, 82169, 82176, 82212, 82214, 82214, 82214, 82225, 82228, 82239, 82239, 82240, 82240, 82241, 82245, 82245, 82283, 82290, 82296, 82302, 82308, 82315, 82326, 82759, 82760, 82765, 82767, 82769, 82816, 82821, 82821, 82976, 82978, 82978, 82993, 83003, 83003, 83060, 83103, 83103, 83941, 83941, 83942, 83951, 83951, 83952, 83964, 83965, 83965, 83965, 83992, 84015, 84017, 84750, 84764, 84770, 84834, 85120, 85121, 85122, 85124, 85362, 85372, 85390, 85431, 85436, 86958, 86980, 86983, 87021, 88386, 88389, 88538, 88606, 88633, 88634, 88634, 88635, 88635, 88659, 88659, 88714, 88714], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-15"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-16<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LUX", "ZAF", "MEX", "OMN", "ETH", "DZA", "EST", "JPN", "GBR", "BHR", "PRY", "URY", "SRB", "PER", "IDN", "MKD", "IRN", "ISL", "CHN", "AUT", "SYC", "NOR", "THA", "BHS", "ARE", "HUN", "GHA", "MRT", "SGP", "IRL", "VAT", "KEN", "GUY", "MDV", "MAR", "SVN", "PSE", "AFG", "BFA", "KAZ", "BLR", "MLT", "VNM", "XKX", "LKA", "CZE", "BEL", "KHM", "AND", "CHE", "PAN", "ITA", "CHL", "TGO", "DNK", "GRC", "UZB", "KWT", "HND", "CAF", "AZE", "PHL", "COD", "SWZ", "GNQ", "SMR", "COG", "JAM", "USA", "IND", "CMR", "QAT", "ARG", "ECU", "JOR", "VEN", "EGY", "DOM", "PAK", "TWN", "GEO", "TUR", "PRT", "TUN", "COL", "NGA", "LIE", "BRA", "GAB", "FRA", "ARM", "CAN", "BGR", "LTU", "POL", "BIH", "SWE", "CRI", "NLD", "AUS", "MYS", "LBN", "HRV", "IRQ", "CYP", "BRN", "KOR", "FIN", "MCO", "LVA", "TTO", "RWA", "UKR", "SEN", "RUS", "SDN", "DEU", "NAM", "ESP", "ROU", "CIV", "GTM", "ISR", "SAU", "ALB", "LCA", "MDA", "SVK"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX", "ZAF", "MEX", "OMN", "ETH", "DZA", "EST", "JPN", "GBR", "BHR", "PRY", "URY", "SRB", "PER", "IDN", "MKD", "IRN", "ISL", "CHN", "AUT", "SYC", "NOR", "THA", "BHS", "ARE", "HUN", "GHA", "MRT", "SGP", "IRL", "VAT", "KEN", "GUY", "MDV", "MAR", "SVN", "PSE", "AFG", "BFA", "KAZ", "BLR", "MLT", "VNM", "XKX", "LKA", "CZE", "BEL", "KHM", "AND", "CHE", "PAN", "ITA", "CHL", "TGO", "DNK", "GRC", "UZB", "KWT", "HND", "CAF", "AZE", "PHL", "COD", "SWZ", "GNQ", "SMR", "COG", "JAM", "USA", "IND", "CMR", "QAT", "ARG", "ECU", "JOR", "VEN", "EGY", "DOM", "PAK", "TWN", "GEO", "TUR", "PRT", "TUN", "COL", "NGA", "LIE", "BRA", "GAB", "FRA", "ARM", "CAN", "BGR", "LTU", "POL", "BIH", "SWE", "CRI", "NLD", "AUS", "MYS", "LBN", "HRV", "IRQ", "CYP", "BRN", "KOR", "FIN", "MCO", "LVA", "TTO", "RWA", "UKR", "SEN", "RUS", "SDN", "DEU", "NAM", "ESP", "ROU", "CIV", "GTM", "ISR", "SAU", "ALB", "LCA", "MDA", "SVK"], "marker": {"color": "#636efa", "size": [88753, 88780, 88792, 88794, 88797, 88808, 88864, 88898, 89149, 89152, 89153, 89155, 89164, 89192, 89213, 89213, 90422, 90462, 90487, 90692, 90693, 90863, 90895, 90896, 90897, 90905, 90905, 90905, 90917, 90957, 90957, 90959, 90962, 90966, 90976, 91014, 91014, 91020, 91020, 91023, 91029, 91032, 91036, 91038, 91046, 91130, 91327, 91332, 91335, 92176, 92176, 98406, 98420, 98420, 98468, 98571, 98577, 98585, 98588, 98589, 98589, 98618, 98618, 98618, 98618, 98624, 98625, 98627, 99450, 99453, 99454, 99518, 99529, 99538, 99552, 99557, 99574, 99574, 99575, 99575, 99575, 99591, 99667, 99669, 99680, 99680, 99683, 99762, 99762, 100686, 100696, 100756, 100766, 100771, 100792, 100807, 100915, 100923, 101099, 101148, 101338, 101344, 101355, 101394, 101403, 101413, 101487, 101544, 101548, 101553, 101555, 101559, 101559, 101564, 101568, 101568, 102611, 102611, 104611, 104637, 104637, 104637, 104709, 104741, 104745, 104745, 104748, 104765], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-16"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-17<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CZE", "ETH", "GTM", "NPL", "COD", "JOR", "GIN", "BHS", "BGR", "MLT", "SGP", "UKR", "PAK", "TUR", "ARE", "LUX", "GNQ", "DNK", "CHE", "BHR", "VEN", "GBR", "NAM", "MCO", "LCA", "LBN", "DEU", "FIN", "MDA", "SAU", "DOM", "IRQ", "EGY", "AUS", "ECU", "TZA", "SWE", "QAT", "USA", "CMR", "SMR", "GEO", "POL", "LTU", "LBR", "ARM", "CAN", "LIE", "GAB", "PRT", "SDN", "LVA", "SEN", "PHL", "KAZ", "MMR", "PSE", "LKA", "KEN", "AFG", "MAR", "MRT", "XKX", "CAF", "KWT", "GRC", "UZB", "BFA", "PAN", "GHA", "AZE", "DZA", "PRY", "URY", "PER", "IRN", "SYC", "RWA", "MKD", "AUT", "SRB", "MEX", "KHM", "JPN", "NOR", "CHN", "MYS", "IND", "BRA", "MDV", "THA", "HND", "ISL", "BRN", "ISR", "ROU", "CYP", "NLD", "CHL", "TTO", "SWZ", "SOM", "BGD", "VAT", "HRV", "OMN", "GUY", "COG", "CRI", "ALB", "TWN", "EST", "VNM", "BTN", "FRA", "TUN", "NGA", "IRL", "COL", "RUS", "TGO", "SVN", "ITA", "BEL", "KOR", "ESP", "CIV", "BEN", "ARG", "IDN", "BOL", "HUN", "ZAF", "BLR", "JAM", "AND", "SVK", "BIH"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CZE", "ETH", "GTM", "NPL", "COD", "JOR", "GIN", "BHS", "BGR", "MLT", "SGP", "UKR", "PAK", "TUR", "ARE", "LUX", "GNQ", "DNK", "CHE", "BHR", "VEN", "GBR", "NAM", "MCO", "LCA", "LBN", "DEU", "FIN", "MDA", "SAU", "DOM", "IRQ", "EGY", "AUS", "ECU", "TZA", "SWE", "QAT", "USA", "CMR", "SMR", "GEO", "POL", "LTU", "LBR", "ARM", "CAN", "LIE", "GAB", "PRT", "SDN", "LVA", "SEN", "PHL", "KAZ", "MMR", "PSE", "LKA", "KEN", "AFG", "MAR", "MRT", "XKX", "CAF", "KWT", "GRC", "UZB", "BFA", "PAN", "GHA", "AZE", "DZA", "PRY", "URY", "PER", "IRN", "SYC", "RWA", "MKD", "AUT", "SRB", "MEX", "KHM", "JPN", "NOR", "CHN", "MYS", "IND", "BRA", "MDV", "THA", "HND", "ISL", "BRN", "ISR", "ROU", "CYP", "NLD", "CHL", "TTO", "SWZ", "SOM", "BGD", "VAT", "HRV", "OMN", "GUY", "COG", "CRI", "ALB", "TWN", "EST", "VNM", "BTN", "FRA", "TUN", "NGA", "IRL", "COL", "RUS", "TGO", "SVN", "ITA", "BEL", "KOR", "ESP", "CIV", "BEN", "ARG", "IDN", "BOL", "HUN", "ZAF", "BLR", "JAM", "AND", "SVK", "BIH"], "marker": {"color": "#636efa", "size": [104811, 104812, 104817, 104817, 104818, 104819, 104819, 104819, 104830, 104839, 104856, 104858, 105014, 105043, 105055, 105059, 105059, 105116, 105116, 105123, 105141, 105293, 105293, 105295, 105295, 105316, 106490, 106495, 106501, 106516, 106516, 106516, 106532, 106609, 106630, 106631, 106720, 106758, 107645, 107645, 107649, 107652, 107704, 107707, 107708, 107730, 107850, 107850, 107850, 107936, 107936, 107941, 107942, 107944, 107946, 107946, 107947, 107957, 107957, 107962, 107971, 107971, 107971, 107971, 107982, 108003, 108005, 108022, 108048, 108052, 108052, 108064, 108065, 108086, 108101, 109154, 109155, 109155, 109161, 109317, 109319, 109348, 109360, 109370, 109462, 109572, 109697, 109729, 109763, 109763, 109826, 109828, 109849, 109853, 109863, 109908, 109918, 110196, 110277, 110278, 110278, 110279, 110281, 110281, 110289, 110291, 110291, 110291, 110297, 110306, 110314, 110348, 110352, 110352, 111562, 111564, 111564, 111618, 111630, 111660, 111660, 111694, 115694, 115893, 115977, 117415, 117416, 117417, 117426, 117443, 117444, 117455, 117466, 117475, 117475, 117484, 117507, 117510], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-17"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-18<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["DOM", "UZB", "HUN", "ITA", "GRC", "PAN", "ECU", "AFG", "IRL", "MAR", "AUS", "IND", "MRT", "CUB", "MNE", "BEL", "EGY", "HND", "MNG", "ALB", "KWT", "IRQ", "CAF", "CRI", "XKX", "ISR", "TUN", "SAU", "LCA", "EST", "SYC", "THA", "MCO", "ZAF", "KOR", "AUT", "URY", "MEX", "SRB", "MKD", "PHL", "IDN", "BTN", "NZL", "RUS", "SEN", "BEN", "KHM", "ISL", "LBN", "ESP", "PRY", "MDA", "CHN", "AZE", "DZA", "GHA", "CIV", "IRN", "JPN", "GBR", "DEU", "AND", "HRV", "RWA", "BRN", "SOM", "PER", "OMN", "VAT", "SDN", "LVA", "CZE", "CYP", "SWZ", "LBR", "ARG", "GUY", "BHR", "LTU", "TTO", "ARE", "LKA", "TWN", "BGD", "KEN", "COD", "GMB", "POL", "BIH", "DNK", "UKR", "TGO", "MLT", "PRT", "GIN", "COL", "NGA", "SVK", "CHL", "FRA", "TUR", "GAB", "BGR", "NOR", "CAN", "GNQ", "PAK", "JAM", "LIE", "NLD", "GTM", "MYS", "BRA", "ARM", "CHE", "LUX", "COG", "BOL", "ETH", "NPL", "CMR", "SWE", "JOR", "TZA", "KAZ", "VEN", "BFA", "PSE", "QAT", "USA", "BLR", "ROU", "SMR", "NAM", "MDV", "FIN", "GEO", "BRB", "SGP", "VNM", "SVN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["DOM", "UZB", "HUN", "ITA", "GRC", "PAN", "ECU", "AFG", "IRL", "MAR", "AUS", "IND", "MRT", "CUB", "MNE", "BEL", "EGY", "HND", "MNG", "ALB", "KWT", "IRQ", "CAF", "CRI", "XKX", "ISR", "TUN", "SAU", "LCA", "EST", "SYC", "THA", "MCO", "ZAF", "KOR", "AUT", "URY", "MEX", "SRB", "MKD", "PHL", "IDN", "BTN", "NZL", "RUS", "SEN", "BEN", "KHM", "ISL", "LBN", "ESP", "PRY", "MDA", "CHN", "AZE", "DZA", "GHA", "CIV", "IRN", "JPN", "GBR", "DEU", "AND", "HRV", "RWA", "BRN", "SOM", "PER", "OMN", "VAT", "SDN", "LVA", "CZE", "CYP", "SWZ", "LBR", "ARG", "GUY", "BHR", "LTU", "TTO", "ARE", "LKA", "TWN", "BGD", "KEN", "COD", "GMB", "POL", "BIH", "DNK", "UKR", "TGO", "MLT", "PRT", "GIN", "COL", "NGA", "SVK", "CHL", "FRA", "TUR", "GAB", "BGR", "NOR", "CAN", "GNQ", "PAK", "JAM", "LIE", "NLD", "GTM", "MYS", "BRA", "ARM", "CHE", "LUX", "COG", "BOL", "ETH", "NPL", "CMR", "SWE", "JOR", "TZA", "KAZ", "VEN", "BFA", "PSE", "QAT", "USA", "BLR", "ROU", "SMR", "NAM", "MDV", "FIN", "GEO", "BRB", "SGP", "VNM", "SVN"], "marker": {"color": "#636efa", "size": [117510, 117518, 117518, 121044, 121079, 121096, 121149, 121150, 121219, 121226, 121305, 121317, 121317, 121320, 121322, 121480, 121520, 121521, 121524, 121528, 121535, 121565, 121565, 121574, 121591, 121758, 121762, 121762, 121762, 121782, 121782, 121782, 121782, 121805, 121898, 122214, 122235, 122246, 122261, 122273, 122318, 122356, 122356, 122368, 122389, 122393, 122393, 122393, 122441, 122441, 124428, 124430, 124431, 124464, 124473, 124473, 124473, 124473, 125651, 125656, 126063, 127207, 127207, 127220, 127222, 127224, 127224, 127255, 127255, 127255, 127255, 127280, 127370, 127370, 127370, 127371, 127385, 127385, 127401, 127409, 127411, 127426, 127439, 127449, 127452, 127453, 127453, 127454, 127515, 127515, 127607, 127616, 127616, 127624, 127741, 127741, 127749, 127750, 127763, 127808, 128905, 128956, 128956, 128975, 129114, 129259, 129259, 129259, 129262, 129262, 129554, 129554, 129674, 129731, 129757, 130207, 130266, 130266, 130267, 130267, 130267, 130273, 130319, 130338, 130338, 130360, 130360, 130360, 130362, 130365, 132131, 132131, 132164, 132166, 132166, 132166, 132213, 132214, 132215, 132238, 132238, 132260], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-18"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-19<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GAB", "LIE", "IND", "BOL", "MDA", "COL", "NOR", "ESP", "KOR", "BTN", "EGY", "PYF", "DJI", "RUS", "SWE", "MCO", "TZA", "SEN", "AUS", "SAU", "PRT", "IRQ", "GEO", "QAT", "POL", "DEU", "BIH", "COG", "ISR", "DOM", "CIV", "GMB", "ROU", "SMR", "LCA", "TWN", "BRN", "HRV", "LTU", "LBR", "CMR", "CRI", "BRA", "LBN", "FIN", "ECU", "ARM", "USA", "SWZ", "ALB", "JPN", "NZL", "FRA", "NIC", "DNK", "TGO", "CHE", "BRB", "SGP", "MDV", "PSE", "BFA", "NPL", "MYS", "CAN", "ETH", "BLR", "NAM", "VEN", "KAZ", "SVN", "LVA", "VNM", "IRL", "VAT", "KEN", "JOR", "KHM", "COD", "BGD", "TUN", "GIN", "SVK", "TUR", "CHL", "BGR", "PAK", "JAM", "NLD", "GNQ", "NGA", "LUX", "GTM", "MLT", "CZE", "UKR", "CYP", "SLV", "ARE", "GUY", "LKA", "BHR", "TTO", "ARG", "GUM", "MAR", "AFG", "CUB", "CHN", "SOM", "MRT", "GHA", "GBR", "OMN", "AND", "IRN", "RWA", "PER", "ISL", "URY", "PHL", "SYC", "MEX", "THA", "ZAF", "BEN", "AUT", "SRB", "MKD", "KGZ", "IDN", "ZMB", "SDN", "PRY", "DZA", "AZE", "KWT", "BEL", "GRC", "MNE", "EST", "UZB", "MNG", "PAN", "ITA", "HUN", "HND", "XKX", "CAF"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GAB", "LIE", "IND", "BOL", "MDA", "COL", "NOR", "ESP", "KOR", "BTN", "EGY", "PYF", "DJI", "RUS", "SWE", "MCO", "TZA", "SEN", "AUS", "SAU", "PRT", "IRQ", "GEO", "QAT", "POL", "DEU", "BIH", "COG", "ISR", "DOM", "CIV", "GMB", "ROU", "SMR", "LCA", "TWN", "BRN", "HRV", "LTU", "LBR", "CMR", "CRI", "BRA", "LBN", "FIN", "ECU", "ARM", "USA", "SWZ", "ALB", "JPN", "NZL", "FRA", "NIC", "DNK", "TGO", "CHE", "BRB", "SGP", "MDV", "PSE", "BFA", "NPL", "MYS", "CAN", "ETH", "BLR", "NAM", "VEN", "KAZ", "SVN", "LVA", "VNM", "IRL", "VAT", "KEN", "JOR", "KHM", "COD", "BGD", "TUN", "GIN", "SVK", "TUR", "CHL", "BGR", "PAK", "JAM", "NLD", "GNQ", "NGA", "LUX", "GTM", "MLT", "CZE", "UKR", "CYP", "SLV", "ARE", "GUY", "LKA", "BHR", "TTO", "ARG", "GUM", "MAR", "AFG", "CUB", "CHN", "SOM", "MRT", "GHA", "GBR", "OMN", "AND", "IRN", "RWA", "PER", "ISL", "URY", "PHL", "SYC", "MEX", "THA", "ZAF", "BEN", "AUT", "SRB", "MKD", "KGZ", "IDN", "ZMB", "SDN", "PRY", "DZA", "AZE", "KWT", "BEL", "GRC", "MNE", "EST", "UZB", "MNG", "PAN", "ITA", "HUN", "HND", "XKX", "CAF"], "marker": {"color": "#636efa", "size": [132262, 132280, 132308, 132308, 132314, 132351, 132466, 135004, 135156, 135156, 135186, 135189, 135190, 135223, 135357, 135357, 135359, 135364, 135475, 135513, 135707, 135717, 135717, 135727, 135776, 136818, 136833, 136835, 136841, 136851, 136852, 136852, 136895, 136900, 136900, 136931, 136943, 136955, 136963, 136963, 136963, 136982, 137119, 137132, 137182, 137239, 137276, 140264, 140264, 140268, 140312, 140320, 141724, 141725, 141816, 141816, 142176, 142176, 142223, 142223, 142226, 142232, 142232, 142349, 142470, 142471, 142481, 142481, 142481, 142485, 142496, 142506, 142521, 142595, 142595, 142598, 142615, 142615, 142626, 142628, 142633, 142633, 142643, 142736, 142773, 142784, 142899, 142901, 143247, 143249, 143254, 143324, 143326, 143336, 143424, 143429, 143447, 143448, 143448, 143449, 143449, 143468, 143470, 143488, 143491, 143501, 143501, 143505, 143580, 143580, 143580, 143581, 144261, 144276, 144315, 145507, 145511, 145539, 145542, 145571, 145586, 145588, 145613, 145613, 145644, 145644, 145958, 145980, 145991, 145994, 145994, 145996, 145997, 145997, 146010, 146016, 146028, 146271, 146302, 146308, 146341, 146348, 146349, 146372, 150579, 150602, 150605, 150605, 150605], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-19"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-20<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MLT", "LTU", "VEN", "PYF", "FIN", "FRO", "MEX", "LCA", "URY", "RWA", "EGY", "ZMB", "RUS", "MUS", "GNQ", "ROU", "DZA", "MYS", "ETH", "QAT", "UZB", "LUX", "ARG", "ALB", "AFG", "MDV", "SLV", "MRT", "AND", "FRA", "SWZ", "EST", "VNM", "CAN", "GAB", "BRB", "BLR", "CYP", "SVN", "HTI", "CUW", "VAT", "TGO", "CUB", "HUN", "BEL", "ITA", "SOM", "OMN", "ISL", "THA", "HND", "ZAF", "TTO", "GUY", "BHS", "GRL", "BHR", "ARE", "UKR", "CZE", "GUM", "BGD", "GTM", "PAK", "TUR", "GIN", "JPN", "TUN", "JAM", "NLD", "GGY", "BEN", "BMU", "BTN", "BGR", "CHL", "TCD", "LKA", "CHE", "BFA", "KHM", "NGA", "CAF", "IRN", "MKD", "SDN", "SWE", "IRQ", "CMR", "FJI", "CYM", "NIC", "IRL", "CHN", "NZL", "KOR", "HRV", "CIV", "ISR", "IND", "BOL", "CRI", "BIH", "TWN", "COG", "BRA", "COL", "ESP", "BRN", "NOR", "IDN", "COD", "DNK", "SVK", "SYC", "MCO", "SEN", "LVA", "DJI", "KGZ", "SRB", "LBN", "AUT", "PHL", "SGP", "PER", "GHA", "GBR", "AZE", "JEY", "PRY", "DEU", "DOM", "LIE", "ARM", "LBR", "PRT", "GMB", "POL", "SAU", "SMR", "USA", "ABW", "TZA", "AUS", "MDA", "ECU", "GEO", "GIB", "JOR", "MNG", "KAZ", "NAM", "KWT", "MNE", "KEN", "GRC", "XKX", "PAN", "NPL", "PSE", "MAR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MLT", "LTU", "VEN", "PYF", "FIN", "FRO", "MEX", "LCA", "URY", "RWA", "EGY", "ZMB", "RUS", "MUS", "GNQ", "ROU", "DZA", "MYS", "ETH", "QAT", "UZB", "LUX", "ARG", "ALB", "AFG", "MDV", "SLV", "MRT", "AND", "FRA", "SWZ", "EST", "VNM", "CAN", "GAB", "BRB", "BLR", "CYP", "SVN", "HTI", "CUW", "VAT", "TGO", "CUB", "HUN", "BEL", "ITA", "SOM", "OMN", "ISL", "THA", "HND", "ZAF", "TTO", "GUY", "BHS", "GRL", "BHR", "ARE", "UKR", "CZE", "GUM", "BGD", "GTM", "PAK", "TUR", "GIN", "JPN", "TUN", "JAM", "NLD", "GGY", "BEN", "BMU", "BTN", "BGR", "CHL", "TCD", "LKA", "CHE", "BFA", "KHM", "NGA", "CAF", "IRN", "MKD", "SDN", "SWE", "IRQ", "CMR", "FJI", "CYM", "NIC", "IRL", "CHN", "NZL", "KOR", "HRV", "CIV", "ISR", "IND", "BOL", "CRI", "BIH", "TWN", "COG", "BRA", "COL", "ESP", "BRN", "NOR", "IDN", "COD", "DNK", "SVK", "SYC", "MCO", "SEN", "LVA", "DJI", "KGZ", "SRB", "LBN", "AUT", "PHL", "SGP", "PER", "GHA", "GBR", "AZE", "JEY", "PRY", "DEU", "DOM", "LIE", "ARM", "LBR", "PRT", "GMB", "POL", "SAU", "SMR", "USA", "ABW", "TZA", "AUS", "MDA", "ECU", "GEO", "GIB", "JOR", "MNG", "KAZ", "NAM", "KWT", "MNE", "KEN", "GRC", "XKX", "PAN", "NPL", "PSE", "MAR"], "marker": {"color": "#636efa", "size": [150610, 150625, 150625, 150625, 150656, 150728, 150774, 150774, 150789, 150789, 150803, 150803, 150855, 150858, 150859, 150876, 150885, 150995, 150996, 151004, 151004, 151139, 151170, 151181, 151181, 151181, 151181, 151182, 151204, 153065, 153065, 153074, 153083, 153239, 153239, 153240, 153251, 153251, 153284, 153286, 153288, 153288, 153288, 153293, 153305, 153614, 158936, 158936, 158936, 159016, 159051, 159063, 159097, 159097, 159097, 159099, 159101, 159114, 159141, 159148, 159320, 159329, 159329, 159330, 159506, 159674, 159674, 159751, 159761, 159762, 160171, 160172, 160173, 160175, 160175, 160188, 160292, 160293, 160304, 161182, 161189, 161189, 161189, 161189, 162235, 162241, 162241, 162363, 162376, 162380, 162381, 162382, 162382, 162573, 162672, 162683, 162770, 162793, 162796, 163040, 163066, 163069, 163087, 163095, 163095, 163095, 163288, 163314, 166745, 166750, 166879, 166934, 166934, 166970, 166986, 166986, 166988, 166988, 167003, 167003, 167006, 167038, 167054, 167604, 167632, 167664, 167753, 167757, 168404, 168414, 168419, 168421, 174361, 174361, 174361, 174368, 174368, 174511, 174511, 174579, 174646, 174663, 179498, 179500, 179500, 179644, 179657, 179688, 179694, 179704, 179708, 179708, 179720, 179720, 179726, 179731, 179731, 179777, 179779, 179807, 179807, 179810, 179819], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-20"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-21<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SVK", "NOR", "MDA", "SAU", "MAR", "NIC", "UKR", "NER", "NGA", "TZA", "SMR", "USA", "SVN", "MLT", "RWA", "MRT", "SWE", "SUR", "MKD", "PRT", "SDN", "NPL", "ZMB", "ARE", "POL", "URY", "CHE", "NAM", "PAK", "LKA", "ESP", "ROU", "MSR", "ZAF", "VEN", "OMN", "PER", "TTO", "MNG", "GBR", "SOM", "TGO", "PRY", "PNG", "UZB", "PAN", "VNM", "MDV", "SGP", "QAT", "THA", "MEX", "MCO", "TWN", "TUR", "SEN", "LCA", "RUS", "MUS", "TUN", "SRB", "NLD", "KOR", "MNE", "NZL", "NCL", "SYC", "PHL", "PSE", "ZWE", "ISL", "BGD", "LIE", "ITA", "BRB", "LTU", "SWZ", "GIN", "ATG", "PYF", "BLR", "COL", "ISR", "KGZ", "CUB", "GGY", "BEL", "GAB", "BEN", "GUY", "BMU", "IRL", "HTI", "ARG", "BTN", "BOL", "VAT", "LUX", "BIH", "GMB", "IRQ", "DJI", "DOM", "JAM", "GTM", "CIV", "FIN", "HRV", "AUS", "LBN", "GHA", "AUT", "FJI", "KWT", "ARM", "GRL", "XKX", "CRI", "KEN", "FRO", "EGY", "COG", "LBR", "JPN", "BHR", "ETH", "ECU", "DZA", "BRA", "FRA", "GUM", "AZE", "JEY", "JOR", "KAZ", "SLV", "BHS", "GNQ", "IMN", "LVA", "DEU", "COD", "CAN", "CPV", "CZE", "HUN", "BFA", "CAF", "GIB", "HND", "CYM", "KHM", "GRC", "IDN", "MYS", "DNK", "CMR", "TCD", "IRN", "MDG", "IND", "BGR", "CYP", "ALB", "EST", "AND", "BRN", "AFG", "GEO", "CHN", "CHL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SVK", "NOR", "MDA", "SAU", "MAR", "NIC", "UKR", "NER", "NGA", "TZA", "SMR", "USA", "SVN", "MLT", "RWA", "MRT", "SWE", "SUR", "MKD", "PRT", "SDN", "NPL", "ZMB", "ARE", "POL", "URY", "CHE", "NAM", "PAK", "LKA", "ESP", "ROU", "MSR", "ZAF", "VEN", "OMN", "PER", "TTO", "MNG", "GBR", "SOM", "TGO", "PRY", "PNG", "UZB", "PAN", "VNM", "MDV", "SGP", "QAT", "THA", "MEX", "MCO", "TWN", "TUR", "SEN", "LCA", "RUS", "MUS", "TUN", "SRB", "NLD", "KOR", "MNE", "NZL", "NCL", "SYC", "PHL", "PSE", "ZWE", "ISL", "BGD", "LIE", "ITA", "BRB", "LTU", "SWZ", "GIN", "ATG", "PYF", "BLR", "COL", "ISR", "KGZ", "CUB", "GGY", "BEL", "GAB", "BEN", "GUY", "BMU", "IRL", "HTI", "ARG", "BTN", "BOL", "VAT", "LUX", "BIH", "GMB", "IRQ", "DJI", "DOM", "JAM", "GTM", "CIV", "FIN", "HRV", "AUS", "LBN", "GHA", "AUT", "FJI", "KWT", "ARM", "GRL", "XKX", "CRI", "KEN", "FRO", "EGY", "COG", "LBR", "JPN", "BHR", "ETH", "ECU", "DZA", "BRA", "FRA", "GUM", "AZE", "JEY", "JOR", "KAZ", "SLV", "BHS", "GNQ", "IMN", "LVA", "DEU", "COD", "CAN", "CPV", "CZE", "HUN", "BFA", "CAF", "GIB", "HND", "CYM", "KHM", "GRC", "IDN", "MYS", "DNK", "CMR", "TCD", "IRN", "MDG", "IND", "BGR", "CYP", "ALB", "EST", "AND", "BRN", "AFG", "GEO", "CHN", "CHL"], "marker": {"color": "#636efa", "size": [179833, 180023, 180040, 180076, 180099, 180099, 180099, 180100, 180104, 180104, 180129, 185503, 185525, 185536, 185542, 185542, 185742, 185742, 185764, 185999, 185999, 185999, 185999, 185999, 186069, 186085, 187037, 187038, 187055, 187068, 189901, 189932, 189933, 189988, 189991, 190000, 190029, 190029, 190030, 190736, 190736, 190744, 190749, 190750, 190760, 190823, 190825, 190825, 190865, 190875, 191029, 191068, 191069, 191096, 191407, 191418, 191418, 191472, 191481, 191496, 191505, 192039, 192186, 192187, 192201, 192203, 192204, 192204, 192205, 192206, 192285, 192292, 192301, 198287, 198291, 198312, 198312, 198313, 198313, 198325, 198325, 198355, 198390, 198390, 198395, 198395, 198857, 198857, 198857, 198857, 198857, 198983, 198983, 199013, 199014, 199018, 199018, 199157, 199157, 199157, 199173, 199173, 199186, 199189, 199193, 199198, 199248, 199270, 199435, 199449, 199454, 199907, 199907, 199918, 199932, 199932, 199935, 199961, 199961, 199969, 200015, 200015, 200015, 200072, 200088, 200090, 200317, 200329, 200612, 202229, 202231, 202231, 202238, 202251, 202255, 202257, 202258, 202258, 202259, 202284, 206333, 206333, 206458, 206460, 206670, 206688, 206695, 206695, 206695, 206695, 206697, 206720, 206751, 206833, 206963, 207067, 207080, 207080, 208317, 208320, 208360, 208382, 208391, 208391, 208407, 208407, 208412, 208414, 208417, 208496, 208588], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-21"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-22<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CPV", "KAZ", "ECU", "NOR", "NPL", "JOR", "CHN", "JEY", "DEU", "AZE", "JPN", "PHL", "ARE", "BHR", "GUM", "SDN", "FRA", "BHS", "CZE", "SMR", "KEN", "NAM", "KGZ", "FIN", "ISL", "CIV", "CHL", "AUS", "TZA", "CYP", "RWA", "GHA", "AUT", "ROU", "LKA", "GBR", "EGY", "MNG", "KWT", "GRL", "OMN", "MNE", "CAF", "MSR", "XKX", "SVK", "CRI", "MAR", "UKR", "LCA", "CYM", "IND", "TCD", "GTM", "GEO", "COG", "KHM", "THA", "SVN", "DJI", "GAB", "GUY", "BEN", "SYC", "BMU", "HND", "PRT", "NIC", "IRN", "IRL", "NER", "NGA", "HTI", "BRN", "BRA", "ESP", "IRQ", "GMB", "TWN", "BEL", "MKD", "BIH", "BGR", "VAT", "BOL", "CHE", "BTN", "SRB", "IMN", "SEN", "COL", "PAK", "TTO", "BGD", "COD", "GGY", "TUN", "CAN", "DOM", "POL", "SAU", "UGA", "JAM", "ZWE", "NLD", "SWE", "SUR", "ITA", "TGO", "ISR", "SGP", "QAT", "DNK", "BLR", "CMR", "BRB", "NZL", "NCL", "GIN", "IDN", "PER", "HUN", "TLS", "PYF", "TUR", "BFA", "LUX", "LVA", "MLT", "LIE", "LBN", "MCO", "MDA", "ETH", "GNQ", "ZMB", "ERI", "MDV", "CUB", "FJI", "URY", "MEX", "AGO", "EST", "LBR", "DZA", "UZB", "PRY", "ARM", "AFG", "GIB", "PAN", "RUS", "ALB", "MUS", "ARG", "SWZ", "KOR", "SOM", "MYS", "PSE", "GRC", "USA", "VNM", "ATG", "MDG", "VEN", "ZAF", "FRO", "LTU", "SLV", "HRV", "AND", "MRT", "PNG"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CPV", "KAZ", "ECU", "NOR", "NPL", "JOR", "CHN", "JEY", "DEU", "AZE", "JPN", "PHL", "ARE", "BHR", "GUM", "SDN", "FRA", "BHS", "CZE", "SMR", "KEN", "NAM", "KGZ", "FIN", "ISL", "CIV", "CHL", "AUS", "TZA", "CYP", "RWA", "GHA", "AUT", "ROU", "LKA", "GBR", "EGY", "MNG", "KWT", "GRL", "OMN", "MNE", "CAF", "MSR", "XKX", "SVK", "CRI", "MAR", "UKR", "LCA", "CYM", "IND", "TCD", "GTM", "GEO", "COG", "KHM", "THA", "SVN", "DJI", "GAB", "GUY", "BEN", "SYC", "BMU", "HND", "PRT", "NIC", "IRN", "IRL", "NER", "NGA", "HTI", "BRN", "BRA", "ESP", "IRQ", "GMB", "TWN", "BEL", "MKD", "BIH", "BGR", "VAT", "BOL", "CHE", "BTN", "SRB", "IMN", "SEN", "COL", "PAK", "TTO", "BGD", "COD", "GGY", "TUN", "CAN", "DOM", "POL", "SAU", "UGA", "JAM", "ZWE", "NLD", "SWE", "SUR", "ITA", "TGO", "ISR", "SGP", "QAT", "DNK", "BLR", "CMR", "BRB", "NZL", "NCL", "GIN", "IDN", "PER", "HUN", "TLS", "PYF", "TUR", "BFA", "LUX", "LVA", "MLT", "LIE", "LBN", "MCO", "MDA", "ETH", "GNQ", "ZMB", "ERI", "MDV", "CUB", "FJI", "URY", "MEX", "AGO", "EST", "LBR", "DZA", "UZB", "PRY", "ARM", "AFG", "GIB", "PAN", "RUS", "ALB", "MUS", "ARG", "SWZ", "KOR", "SOM", "MYS", "PSE", "GRC", "USA", "VNM", "ATG", "MDG", "VEN", "ZAF", "FRO", "LTU", "SLV", "HRV", "AND", "MRT", "PNG"], "marker": {"color": "#636efa", "size": [208589, 208592, 208698, 208882, 208882, 208897, 208980, 208980, 212256, 212265, 212304, 212454, 212467, 212488, 212489, 212489, 214336, 214336, 214427, 214427, 214427, 214427, 214435, 214506, 214570, 214573, 214676, 214900, 214903, 214920, 214920, 214925, 215300, 215359, 215371, 216406, 216435, 216439, 216456, 216456, 216460, 216460, 216462, 216462, 216462, 216503, 216507, 216517, 216532, 216532, 216532, 216621, 216622, 216626, 216632, 216633, 216637, 216870, 216912, 216912, 216912, 216912, 216912, 216912, 216912, 216914, 217174, 217174, 218140, 218242, 218242, 218252, 218252, 218257, 218481, 223427, 223448, 223448, 223466, 224024, 224039, 224087, 224123, 224123, 224124, 225361, 225361, 225375, 225376, 225385, 225437, 225588, 225628, 225635, 225644, 225644, 225650, 225910, 225988, 226099, 226217, 226218, 226218, 226219, 226856, 226979, 226983, 233540, 233546, 233717, 233764, 233775, 233846, 233865, 233865, 233873, 233886, 233888, 233888, 234029, 234084, 234112, 234113, 234115, 234392, 234416, 234602, 234615, 234624, 234626, 234693, 234699, 234713, 234713, 234715, 234715, 234716, 234716, 234720, 234721, 234746, 234794, 234796, 234819, 234820, 234820, 234820, 234824, 234848, 234848, 234848, 234893, 234946, 234952, 234954, 235021, 235021, 235119, 235119, 235272, 235276, 235311, 242434, 242441, 242441, 242441, 242441, 242476, 242488, 242524, 242524, 242604, 242617, 242617, 242617], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-22"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-23<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SMR", "EGY", "NOR", "ECU", "ETH", "UKR", "GAB", "PHL", "NLD", "NIC", "DJI", "SUR", "AGO", "BHS", "JPN", "MDV", "URY", "SYC", "FRA", "BMU", "VNM", "CHN", "DEU", "BEN", "PNG", "BHR", "MDG", "SDN", "AZE", "PRT", "GUM", "ARE", "GUY", "LBR", "HND", "GTM", "CHE", "LVA", "THA", "CAN", "TTO", "DNK", "PAK", "GIB", "ITA", "QAT", "GRC", "HUN", "ATG", "BRB", "SEN", "LTU", "TGO", "BLR", "IDN", "TLS", "NZL", "CMR", "VEN", "GIN", "PYF", "ZAF", "LIE", "BGD", "SWZ", "ISR", "JAM", "KHM", "COG", "BEL", "UZB", "UGA", "SGP", "ERI", "JEY", "SAU", "COD", "MUS", "TUR", "IMN", "DZA", "DOM", "MEX", "SWE", "PSE", "TUN", "RUS", "GGY", "DMA", "PER", "BRN", "CPV", "NCL", "LUX", "SRB", "ESP", "PAN", "TZA", "CAF", "VAT", "MSR", "COL", "PRY", "ARM", "ISL", "MAR", "AUS", "MDA", "XKX", "BOL", "HRV", "CRI", "LCA", "GHA", "ARG", "SVK", "CIV", "BIH", "AUT", "MCO", "TCD", "RWA", "SYR", "GBR", "ROU", "OMN", "TWN", "MNE", "MKD", "MNG", "FJI", "ZMB", "BGR", "GMB", "KWT", "LBN", "GRL", "IRQ", "MLT", "KEN", "LKA", "KOR", "CZE", "AND", "HTI", "NAM", "SLV", "USA", "BRA", "AFG", "KGZ", "POL", "KAZ", "NPL", "MRT", "SVN", "NER", "IRN", "IND", "GRD", "ALB", "BTN", "MYS", "EST", "IRL", "CYM", "GNQ", "SOM", "GEO", "JOR", "CHL", "FIN", "CUB", "BFA", "FRO", "CYP", "ZWE", "NGA", "MOZ"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SMR", "EGY", "NOR", "ECU", "ETH", "UKR", "GAB", "PHL", "NLD", "NIC", "DJI", "SUR", "AGO", "BHS", "JPN", "MDV", "URY", "SYC", "FRA", "BMU", "VNM", "CHN", "DEU", "BEN", "PNG", "BHR", "MDG", "SDN", "AZE", "PRT", "GUM", "ARE", "GUY", "LBR", "HND", "GTM", "CHE", "LVA", "THA", "CAN", "TTO", "DNK", "PAK", "GIB", "ITA", "QAT", "GRC", "HUN", "ATG", "BRB", "SEN", "LTU", "TGO", "BLR", "IDN", "TLS", "NZL", "CMR", "VEN", "GIN", "PYF", "ZAF", "LIE", "BGD", "SWZ", "ISR", "JAM", "KHM", "COG", "BEL", "UZB", "UGA", "SGP", "ERI", "JEY", "SAU", "COD", "MUS", "TUR", "IMN", "DZA", "DOM", "MEX", "SWE", "PSE", "TUN", "RUS", "GGY", "DMA", "PER", "BRN", "CPV", "NCL", "LUX", "SRB", "ESP", "PAN", "TZA", "CAF", "VAT", "MSR", "COL", "PRY", "ARM", "ISL", "MAR", "AUS", "MDA", "XKX", "BOL", "HRV", "CRI", "LCA", "GHA", "ARG", "SVK", "CIV", "BIH", "AUT", "MCO", "TCD", "RWA", "SYR", "GBR", "ROU", "OMN", "TWN", "MNE", "MKD", "MNG", "FJI", "ZMB", "BGR", "GMB", "KWT", "LBN", "GRL", "IRQ", "MLT", "KEN", "LKA", "KOR", "CZE", "AND", "HTI", "NAM", "SLV", "USA", "BRA", "AFG", "KGZ", "POL", "KAZ", "NPL", "MRT", "SVN", "NER", "IRN", "IND", "GRD", "ALB", "BTN", "MYS", "EST", "IRL", "CYM", "GNQ", "SOM", "GEO", "JOR", "CHL", "FIN", "CUB", "BFA", "FRO", "CYP", "ZWE", "NGA", "MOZ"], "marker": {"color": "#636efa", "size": [242617, 242626, 242832, 243089, 243091, 243097, 243100, 243100, 243673, 243674, 243674, 243674, 243674, 243674, 243717, 243717, 243740, 243740, 245299, 245303, 245327, 245477, 248788, 248788, 248788, 248816, 248816, 248816, 248828, 249148, 249160, 249160, 249160, 249160, 249161, 249163, 250057, 250072, 250194, 250393, 250394, 250463, 250601, 250606, 256166, 256179, 256273, 256309, 256309, 256312, 256323, 256361, 256361, 256361, 256425, 256425, 256461, 256461, 256461, 256461, 256462, 256496, 256506, 256509, 256512, 256700, 256700, 256735, 256735, 257321, 257334, 257334, 257357, 257357, 257360, 257479, 257482, 257496, 257785, 257785, 257793, 257883, 257948, 258108, 258115, 258130, 258262, 258278, 258279, 258324, 258329, 258329, 258330, 258458, 258497, 262143, 262211, 262217, 262218, 262218, 262218, 262243, 262243, 262273, 262368, 262387, 262998, 263012, 263019, 263026, 263055, 263072, 263072, 263075, 263116, 263123, 263131, 263164, 263771, 263776, 263776, 263778, 263779, 264444, 264510, 264513, 264525, 264532, 264561, 264561, 264562, 264563, 264585, 264585, 264597, 264615, 264615, 264634, 264651, 264659, 264668, 264732, 264902, 264927, 264927, 264927, 264927, 273386, 273804, 273814, 273814, 273912, 273916, 273916, 273916, 273947, 273947, 274975, 275094, 275095, 275108, 275108, 275231, 275251, 275372, 275372, 275372, 275372, 275377, 275392, 275487, 275592, 275602, 275602, 275625, 275636, 275636, 275644, 275645], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-23"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-24<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["VEN", "COL", "ZAF", "SRB", "NZL", "POL", "IRQ", "DNK", "MLT", "QAT", "GEO", "BLR", "CMR", "ATG", "PNG", "BRA", "SOM", "AND", "BGR", "CUB", "CYP", "BLZ", "GUY", "BFA", "MRT", "BEN", "NGA", "CHE", "VNM", "HTI", "IRL", "BMU", "NER", "GMB", "SYC", "ALB", "CUW", "BTN", "PRT", "NIC", "HND", "MDV", "BOL", "MUS", "ISR", "SYR", "MKD", "TWN", "MEX", "ARG", "PAN", "IMN", "BIH", "ESP", "ERI", "BEL", "SWZ", "LUX", "KHM", "VAT", "THA", "SGP", "DJI", "GAB", "GNQ", "IRN", "TUR", "NCL", "MAR", "XKX", "MDA", "KEN", "CRI", "CYM", "MOZ", "ISL", "SVK", "MMR", "SLV", "NAM", "PRY", "KOR", "KAZ", "GRD", "IND", "FRA", "EST", "CHN", "FRO", "AZE", "EGY", "MSR", "VIR", "CPV", "JEY", "MYS", "SMR", "JOR", "NPL", "AGO", "DEU", "CAF", "ARM", "LVA", "SVN", "ZWE", "FIN", "HRV", "KGZ", "USA", "CHL", "AUS", "ABW", "LBN", "TZA", "OMN", "CIV", "LKA", "RWA", "AFG", "ZMB", "MNE", "CZE", "ROU", "KWT", "FJI", "GHA", "MNG", "GBR", "GIB", "LCA", "TCD", "MCO", "AUT", "GRL", "URY", "GUM", "COD", "JAM", "COG", "UGA", "BHS", "SAU", "SWE", "GGY", "DZA", "DOM", "TUN", "RUS", "LIE", "BGD", "UZB", "DMA", "CAN", "ITA", "GRC", "PAK", "SEN", "BRB", "LTU", "TGO", "IDN", "TLS", "HUN", "GIN", "PYF", "TTO", "NOR", "BRN", "LBR", "ARE", "UKR", "JPN", "SUR", "PER", "BHR", "SDN", "PHL", "ETH", "PSE", "ECU", "GTM", "MDG", "NLD"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["VEN", "COL", "ZAF", "SRB", "NZL", "POL", "IRQ", "DNK", "MLT", "QAT", "GEO", "BLR", "CMR", "ATG", "PNG", "BRA", "SOM", "AND", "BGR", "CUB", "CYP", "BLZ", "GUY", "BFA", "MRT", "BEN", "NGA", "CHE", "VNM", "HTI", "IRL", "BMU", "NER", "GMB", "SYC", "ALB", "CUW", "BTN", "PRT", "NIC", "HND", "MDV", "BOL", "MUS", "ISR", "SYR", "MKD", "TWN", "MEX", "ARG", "PAN", "IMN", "BIH", "ESP", "ERI", "BEL", "SWZ", "LUX", "KHM", "VAT", "THA", "SGP", "DJI", "GAB", "GNQ", "IRN", "TUR", "NCL", "MAR", "XKX", "MDA", "KEN", "CRI", "CYM", "MOZ", "ISL", "SVK", "MMR", "SLV", "NAM", "PRY", "KOR", "KAZ", "GRD", "IND", "FRA", "EST", "CHN", "FRO", "AZE", "EGY", "MSR", "VIR", "CPV", "JEY", "MYS", "SMR", "JOR", "NPL", "AGO", "DEU", "CAF", "ARM", "LVA", "SVN", "ZWE", "FIN", "HRV", "KGZ", "USA", "CHL", "AUS", "ABW", "LBN", "TZA", "OMN", "CIV", "LKA", "RWA", "AFG", "ZMB", "MNE", "CZE", "ROU", "KWT", "FJI", "GHA", "MNG", "GBR", "GIB", "LCA", "TCD", "MCO", "AUT", "GRL", "URY", "GUM", "COD", "JAM", "COG", "UGA", "BHS", "SAU", "SWE", "GGY", "DZA", "DOM", "TUN", "RUS", "LIE", "BGD", "UZB", "DMA", "CAN", "ITA", "GRC", "PAK", "SEN", "BRB", "LTU", "TGO", "IDN", "TLS", "HUN", "GIN", "PYF", "TTO", "NOR", "BRN", "LBR", "ARE", "UKR", "JPN", "SUR", "PER", "BHR", "SDN", "PHL", "ETH", "PSE", "ECU", "GTM", "MDG", "NLD"], "marker": {"color": "#636efa", "size": [275693, 275764, 275892, 275926, 275966, 276081, 276114, 276179, 276196, 276203, 276210, 276215, 276244, 276244, 276244, 276589, 276589, 276609, 276625, 276630, 276651, 276652, 276652, 276663, 276663, 276666, 276676, 277720, 277725, 277728, 277947, 277947, 277948, 277949, 277949, 277960, 277963, 277963, 278423, 278423, 278426, 278426, 278427, 278435, 278806, 278806, 278828, 278858, 278909, 278944, 278976, 278987, 278991, 283508, 283508, 283850, 283850, 283927, 283928, 283928, 284034, 284088, 284090, 284090, 284093, 285504, 285797, 285799, 285818, 285848, 285863, 285864, 285888, 285888, 285888, 285908, 285914, 285916, 285918, 285918, 285923, 285999, 286001, 286001, 286054, 289892, 289918, 290017, 290020, 290027, 290060, 290060, 290077, 290077, 290080, 290292, 290328, 290341, 290342, 290342, 294780, 294780, 294784, 294825, 294853, 294853, 294927, 294998, 295000, 306236, 306350, 306464, 306472, 306491, 306491, 306502, 306502, 306512, 306529, 306535, 306535, 306541, 306612, 306755, 306756, 306757, 306760, 306760, 307727, 307727, 307727, 307727, 307727, 308582, 308584, 308588, 308590, 308590, 308590, 308590, 308598, 308598, 308649, 308759, 308762, 308849, 308892, 308906, 308906, 308906, 308912, 308915, 308915, 309131, 313920, 313991, 314094, 314106, 314106, 314142, 314145, 314210, 314210, 314230, 314230, 314235, 314236, 314475, 314478, 314478, 314523, 314549, 314588, 314589, 314621, 314626, 314626, 314708, 314708, 314708, 314900, 314901, 314915, 315460], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-24"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-25<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["CMR", "HUN", "LKA", "IRN", "CAN", "PNG", "MDG", "SYC", "OMN", "AFG", "IDN", "BGR", "CHL", "CYP", "AGO", "SDN", "CHE", "ZWE", "DNK", "CPV", "MYS", "MKD", "MDV", "SVK", "CYM", "KHM", "ISL", "NOR", "BFA", "ERI", "HND", "PHL", "COD", "CAF", "SGP", "SWE", "ZMB", "IND", "SUR", "ALB", "EST", "CZE", "AND", "CHN", "TCD", "RUS", "GRC", "DEU", "BEL", "SAU", "GTM", "PRY", "BHS", "FRA", "URY", "FRO", "AZE", "EGY", "JEY", "VIR", "KOR", "GUM", "JOR", "SMR", "GNQ", "BHR", "LBR", "ARE", "UKR", "DOM", "TUR", "GGY", "TCA", "UGA", "BRN", "COG", "NPL", "JAM", "ETH", "SVN", "PER", "LBY", "PSE", "JPN", "ECU", "UZB", "TUN", "GRD", "NAM", "MCO", "AUT", "RWA", "CIV", "TZA", "LBN", "ABW", "KGZ", "AUS", "GIB", "USA", "LAO", "FIN", "HRV", "LVA", "GRL", "LCA", "MNG", "GBR", "VCT", "MMR", "MOZ", "CRI", "KEN", "SLV", "MDA", "KAZ", "XKX", "ARM", "MSR", "SOM", "ROU", "KWT", "MNE", "FJI", "MAR", "LIE", "NLD", "BLZ", "DMA", "SWZ", "ESP", "TWN", "ARG", "BRA", "THA", "GAB", "MUS", "GUY", "BGD", "BEN", "SRB", "MRT", "BIH", "VNM", "BOL", "GMB", "BMU", "CUB", "BTN", "VAT", "NIC", "PRT", "HTI", "NER", "NGA", "IRL", "LUX", "SYR", "ZAF", "NCL", "DZA", "COL", "TTO", "GIN", "POL", "PAN", "ITA", "SEN", "BRB", "LTU", "TGO", "PAK", "TLS", "PYF", "IMN", "DJI", "BLR", "NZL", "ATG", "MEX", "IRQ", "QAT", "ISR", "GEO", "VEN", "MLT", "GHA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["CMR", "HUN", "LKA", "IRN", "CAN", "PNG", "MDG", "SYC", "OMN", "AFG", "IDN", "BGR", "CHL", "CYP", "AGO", "SDN", "CHE", "ZWE", "DNK", "CPV", "MYS", "MKD", "MDV", "SVK", "CYM", "KHM", "ISL", "NOR", "BFA", "ERI", "HND", "PHL", "COD", "CAF", "SGP", "SWE", "ZMB", "IND", "SUR", "ALB", "EST", "CZE", "AND", "CHN", "TCD", "RUS", "GRC", "DEU", "BEL", "SAU", "GTM", "PRY", "BHS", "FRA", "URY", "FRO", "AZE", "EGY", "JEY", "VIR", "KOR", "GUM", "JOR", "SMR", "GNQ", "BHR", "LBR", "ARE", "UKR", "DOM", "TUR", "GGY", "TCA", "UGA", "BRN", "COG", "NPL", "JAM", "ETH", "SVN", "PER", "LBY", "PSE", "JPN", "ECU", "UZB", "TUN", "GRD", "NAM", "MCO", "AUT", "RWA", "CIV", "TZA", "LBN", "ABW", "KGZ", "AUS", "GIB", "USA", "LAO", "FIN", "HRV", "LVA", "GRL", "LCA", "MNG", "GBR", "VCT", "MMR", "MOZ", "CRI", "KEN", "SLV", "MDA", "KAZ", "XKX", "ARM", "MSR", "SOM", "ROU", "KWT", "MNE", "FJI", "MAR", "LIE", "NLD", "BLZ", "DMA", "SWZ", "ESP", "TWN", "ARG", "BRA", "THA", "GAB", "MUS", "GUY", "BGD", "BEN", "SRB", "MRT", "BIH", "VNM", "BOL", "GMB", "BMU", "CUB", "BTN", "VAT", "NIC", "PRT", "HTI", "NER", "NGA", "IRL", "LUX", "SYR", "ZAF", "NCL", "DZA", "COL", "TTO", "GIN", "POL", "PAN", "ITA", "SEN", "BRB", "LTU", "TGO", "PAK", "TLS", "PYF", "IMN", "DJI", "BLR", "NZL", "ATG", "MEX", "IRQ", "QAT", "ISR", "GEO", "VEN", "MLT", "GHA"], "marker": {"color": "#636efa", "size": [315476, 315515, 315520, 317282, 317595, 317595, 317597, 317597, 317615, 317617, 317724, 317743, 317919, 317927, 317927, 317928, 318702, 318702, 318833, 318833, 318939, 318951, 318951, 318964, 318964, 318968, 319028, 319223, 319247, 319247, 319253, 319343, 319362, 319362, 319411, 319667, 319667, 319737, 319739, 319762, 319779, 319937, 319968, 320067, 320068, 320125, 320173, 322515, 323041, 323246, 323247, 323257, 323257, 325703, 325730, 325734, 325749, 325788, 325788, 325788, 325888, 325891, 325906, 325906, 325906, 325957, 325957, 326007, 326018, 326085, 326428, 326431, 326432, 326432, 326445, 326445, 326446, 326448, 326448, 326486, 326507, 326508, 326509, 326574, 326675, 326681, 326706, 326706, 326706, 326710, 327506, 327510, 327558, 327558, 327595, 327600, 327626, 328226, 328226, 337015, 337017, 337109, 337185, 337202, 337202, 337202, 337202, 338629, 338629, 338630, 338630, 338649, 338658, 338662, 338678, 338695, 338697, 338768, 338768, 338768, 338954, 338956, 338976, 338977, 339013, 339014, 339825, 339825, 339826, 339826, 346410, 346431, 346517, 346827, 346934, 346934, 346940, 346940, 346946, 346946, 346973, 346973, 347006, 347017, 347021, 347022, 347022, 347030, 347030, 347030, 347030, 347332, 347334, 347339, 347343, 347547, 347771, 347771, 347926, 347928, 347970, 348042, 348048, 348050, 348202, 348300, 353549, 353556, 353557, 353587, 353587, 353691, 353691, 353693, 353703, 353703, 353703, 353750, 353752, 353790, 353840, 353865, 354353, 354362, 354369, 354382, 354408], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-25"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-26<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GIB", "HRV", "CYP", "GRL", "HND", "PAN", "CIV", "OMN", "GRC", "HTI", "GIN", "PHL", "GGY", "PAK", "PSE", "PRY", "COG", "GHA", "GTM", "PER", "KOR", "ESP", "CUW", "ZAF", "HUN", "CHN", "GUY", "GUM", "ISL", "GRD", "CUB", "SOM", "CRI", "VAT", "COL", "PNG", "TLS", "UKR", "ECU", "JPN", "ARE", "ATG", "BHR", "BHS", "SVN", "FRA", "EGY", "AZE", "JEY", "JOR", "SMR", "NPL", "LTU", "VEN", "KAZ", "NLD", "MEX", "PYF", "SWZ", "TGO", "ITA", "BRB", "SEN", "TTO", "JAM", "MUS", "VNM", "DOM", "TUN", "TUR", "TCA", "UGA", "SAU", "BGD", "GNQ", "NAM", "MMR", "TZA", "SLV", "KGZ", "AUS", "USA", "LAO", "LVA", "LBR", "FIN", "LBN", "MCO", "FJI", "FRO", "ARM", "MDA", "URY", "ABW", "KEN", "LBY", "RWA", "VCT", "MOZ", "ETH", "XKX", "MAR", "MSR", "ROU", "UZB", "KWT", "GBR", "MNG", "LCA", "AUT", "DZA", "KNA", "LIE", "MNE", "BLR", "VIR", "NZL", "SWE", "RUS", "DNK", "IDN", "CAN", "CMR", "KHM", "NOR", "SGP", "CHE", "IRN", "BFA", "SYC", "AGO", "MKD", "BGR", "EST", "NCL", "COD", "MDG", "CHL", "ZWE", "LKA", "CZE", "TCD", "CAF", "CYM", "SUR", "SVK", "IND", "SXM", "ZMB", "CPV", "DEU", "SDN", "MYS", "AFG", "POL", "BRN", "NIC", "NER", "GEO", "BTN", "BMU", "GMB", "BEN", "PRT", "MLI", "THA", "MLT", "ARG", "DMA", "IMN", "MRT", "BLZ", "NGA", "IRL", "LUX", "ALB", "QAT", "IRQ", "SYR", "TWN", "BRA", "SRB", "BEL", "BIH", "MDV", "ERI", "GAB", "BOL", "DJI", "AND", "ISR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GIB", "HRV", "CYP", "GRL", "HND", "PAN", "CIV", "OMN", "GRC", "HTI", "GIN", "PHL", "GGY", "PAK", "PSE", "PRY", "COG", "GHA", "GTM", "PER", "KOR", "ESP", "CUW", "ZAF", "HUN", "CHN", "GUY", "GUM", "ISL", "GRD", "CUB", "SOM", "CRI", "VAT", "COL", "PNG", "TLS", "UKR", "ECU", "JPN", "ARE", "ATG", "BHR", "BHS", "SVN", "FRA", "EGY", "AZE", "JEY", "JOR", "SMR", "NPL", "LTU", "VEN", "KAZ", "NLD", "MEX", "PYF", "SWZ", "TGO", "ITA", "BRB", "SEN", "TTO", "JAM", "MUS", "VNM", "DOM", "TUN", "TUR", "TCA", "UGA", "SAU", "BGD", "GNQ", "NAM", "MMR", "TZA", "SLV", "KGZ", "AUS", "USA", "LAO", "LVA", "LBR", "FIN", "LBN", "MCO", "FJI", "FRO", "ARM", "MDA", "URY", "ABW", "KEN", "LBY", "RWA", "VCT", "MOZ", "ETH", "XKX", "MAR", "MSR", "ROU", "UZB", "KWT", "GBR", "MNG", "LCA", "AUT", "DZA", "KNA", "LIE", "MNE", "BLR", "VIR", "NZL", "SWE", "RUS", "DNK", "IDN", "CAN", "CMR", "KHM", "NOR", "SGP", "CHE", "IRN", "BFA", "SYC", "AGO", "MKD", "BGR", "EST", "NCL", "COD", "MDG", "CHL", "ZWE", "LKA", "CZE", "TCD", "CAF", "CYM", "SUR", "SVK", "IND", "SXM", "ZMB", "CPV", "DEU", "SDN", "MYS", "AFG", "POL", "BRN", "NIC", "NER", "GEO", "BTN", "BMU", "GMB", "BEN", "PRT", "MLI", "THA", "MLT", "ARG", "DMA", "IMN", "MRT", "BLZ", "NGA", "IRL", "LUX", "ALB", "QAT", "IRQ", "SYR", "TWN", "BRA", "SRB", "BEL", "BIH", "MDV", "ERI", "GAB", "BOL", "DJI", "AND", "ISR"], "marker": {"color": "#636efa", "size": [354419, 354455, 354463, 354464, 354480, 354625, 354632, 354647, 354725, 354726, 354726, 354810, 354817, 354883, 354885, 354889, 354889, 354904, 354907, 355049, 355153, 363090, 363090, 363242, 363277, 363398, 363398, 363403, 363492, 363492, 363501, 363501, 363525, 363525, 363617, 363617, 363617, 363646, 363775, 363850, 363935, 363935, 363964, 363965, 364013, 366944, 367020, 367026, 367026, 367052, 367073, 367073, 367138, 367153, 367162, 368014, 368084, 368084, 368084, 368089, 373299, 373299, 373312, 373315, 373319, 373325, 373339, 373419, 373478, 374039, 374039, 374044, 374177, 374177, 374179, 374180, 374180, 374180, 374184, 374186, 374186, 388149, 388149, 388173, 388173, 388261, 388290, 388294, 388294, 388304, 388329, 388353, 388381, 388383, 388383, 388383, 388384, 388384, 388388, 388389, 388397, 388452, 388452, 388596, 388606, 388610, 390062, 390062, 390063, 390669, 390702, 390704, 390708, 390714, 390719, 390719, 390813, 391051, 391214, 391347, 391451, 392877, 392877, 392882, 393232, 393242, 394167, 396373, 396388, 396388, 396388, 396417, 396439, 396474, 396479, 396479, 396479, 396699, 396700, 396700, 396960, 396960, 396960, 396960, 396960, 396972, 397059, 397060, 397069, 397070, 402024, 402024, 402196, 402229, 402379, 402382, 402382, 402382, 402385, 402386, 402386, 402386, 402386, 403019, 403021, 403132, 403141, 403256, 403261, 403261, 403261, 403262, 403269, 403504, 403738, 403761, 403772, 403802, 403802, 403821, 404053, 404107, 404775, 404794, 404794, 404797, 404797, 404804, 404813, 404837, 405276], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-26"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-03-27<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SWZ", "EST", "ERI", "RUS", "PRY", "ETH", "FRO", "SVN", "ECU", "FJI", "GMB", "PRT", "CYP", "DJI", "SRB", "GEO", "POL", "DMA", "SYC", "PHL", "DNK", "COD", "DEU", "SXM", "SVK", "CZE", "SGP", "CUW", "SOM", "GHA", "FIN", "SLV", "RWA", "GIB", "KNA", "LCA", "ROU", "VCT", "SMR", "PER", "FRA", "EGY", "SAU", "DOM", "SEN", "PYF", "QAT", "GNQ", "GAB", "AFG", "GRC", "IRL", "NGA", "NER", "NIC", "BTN", "BMU", "LIE", "BEN", "BLZ", "LBY", "LBR", "THA", "IMN", "ISR", "UZB", "BEL", "PNG", "URY", "NZL", "BOL", "BIH", "LTU", "TZA", "CMR", "MRT", "LUX", "KHM", "CHE", "IRN", "MUS", "BFA", "VNM", "NCL", "MEX", "MKD", "BGR", "BRN", "VGB", "VEN", "SYR", "IRQ", "TWN", "BRA", "ATG", "TLS", "TGO", "BLR", "KAZ", "NPL", "KEN", "NAM", "MMR", "MOZ", "GBR", "XKX", "MAR", "MCO", "MSR", "MNE", "MNG", "LBN", "AUS", "AUT", "LVA", "LAO", "USA", "KGZ", "KWT", "CAN", "JOR", "ABW", "ITA", "TTO", "BRB", "VIR", "BGD", "TUN", "TUR", "TCA", "UGA", "JEY", "UKR", null, "NLD", "JPN", "ARM", "ARE", "MDA", "BHR", "BHS", "AZE", "JAM", "IDN", "DZA", "SDN", "GGY", "AND", "ZMB", "HND", "GIN", "LKA", "MDV", "AGO", "PAK", "CHN", "TCD", "COG", "MYS", "CPV", "ALB", "COL", "VAT", "ESP", "HTI", "IND", "GNB", "CAF", "CYM", "GTM", "GUM", "GUY", "GRD", "MLT", "OMN", "SWE", "PAN", "ISL", "ZAF", "CUB", "ARG", "ZWE", "PSE", "CRI", "NOR", "KOR", "MLI", "HRV", "CHL", "SUR", "GRL", "HUN", "MDG", "CIV"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SWZ", "EST", "ERI", "RUS", "PRY", "ETH", "FRO", "SVN", "ECU", "FJI", "GMB", "PRT", "CYP", "DJI", "SRB", "GEO", "POL", "DMA", "SYC", "PHL", "DNK", "COD", "DEU", "SXM", "SVK", "CZE", "SGP", "CUW", "SOM", "GHA", "FIN", "SLV", "RWA", "GIB", "KNA", "LCA", "ROU", "VCT", "SMR", "PER", "FRA", "EGY", "SAU", "DOM", "SEN", "PYF", "QAT", "GNQ", "GAB", "AFG", "GRC", "IRL", "NGA", "NER", "NIC", "BTN", "BMU", "LIE", "BEN", "BLZ", "LBY", "LBR", "THA", "IMN", "ISR", "UZB", "BEL", "PNG", "URY", "NZL", "BOL", "BIH", "LTU", "TZA", "CMR", "MRT", "LUX", "KHM", "CHE", "IRN", "MUS", "BFA", "VNM", "NCL", "MEX", "MKD", "BGR", "BRN", "VGB", "VEN", "SYR", "IRQ", "TWN", "BRA", "ATG", "TLS", "TGO", "BLR", "KAZ", "NPL", "KEN", "NAM", "MMR", "MOZ", "GBR", "XKX", "MAR", "MCO", "MSR", "MNE", "MNG", "LBN", "AUS", "AUT", "LVA", "LAO", "USA", "KGZ", "KWT", "CAN", "JOR", "ABW", "ITA", "TTO", "BRB", "VIR", "BGD", "TUN", "TUR", "TCA", "UGA", "JEY", "UKR", null, "NLD", "JPN", "ARM", "ARE", "MDA", "BHR", "BHS", "AZE", "JAM", "IDN", "DZA", "SDN", "GGY", "AND", "ZMB", "HND", "GIN", "LKA", "MDV", "AGO", "PAK", "CHN", "TCD", "COG", "MYS", "CPV", "ALB", "COL", "VAT", "ESP", "HTI", "IND", "GNB", "CAF", "CYM", "GTM", "GUM", "GUY", "GRD", "MLT", "OMN", "SWE", "PAN", "ISL", "ZAF", "CUB", "ARG", "ZWE", "PSE", "CRI", "NOR", "KOR", "MLI", "HRV", "CHL", "SUR", "GRL", "HUN", "MDG", "CIV"], "marker": {"color": "#636efa", "size": [405278, 405412, 405414, 405596, 405607, 405607, 405615, 405664, 405856, 405856, 405856, 406405, 406419, 406425, 406506, 406512, 406682, 406686, 406686, 406757, 406910, 406919, 412699, 412699, 412709, 413117, 413143, 413144, 413145, 413209, 413287, 413287, 413296, 413305, 413305, 413305, 413428, 413428, 413438, 413460, 417382, 417396, 417508, 417604, 417610, 417615, 417627, 417628, 417629, 417629, 417700, 417955, 417969, 417972, 417972, 417972, 417981, 417986, 417987, 417987, 417987, 417987, 418078, 418081, 418378, 418396, 419694, 419694, 419715, 419770, 419792, 419792, 419817, 419818, 419834, 419835, 419955, 419957, 420957, 423346, 423379, 423411, 423416, 423417, 423527, 423551, 423573, 423580, 423582, 423583, 423587, 423623, 423640, 424122, 424126, 424126, 424127, 424127, 424159, 424159, 424165, 424169, 424171, 424173, 426302, 426310, 426360, 426362, 426366, 426380, 426381, 426416, 427159, 428300, 428323, 428327, 445124, 445124, 445137, 445770, 445789, 445798, 451951, 451956, 451962, 451962, 451971, 451971, 453167, 453168, 453168, 453182, 453225, 453227, 454246, 454342, 454381, 454381, 454409, 454448, 454452, 454481, 454482, 454585, 454626, 454626, 454630, 454666, 454668, 454683, 454684, 454688, 454688, 454689, 454829, 454940, 454942, 454942, 455177, 455178, 455206, 455227, 455231, 463809, 463809, 463884, 463886, 463887, 463890, 463891, 463903, 463903, 463909, 463914, 463924, 464220, 464306, 464371, 464589, 464599, 464686, 464686, 464708, 464738, 464978, 465069, 465071, 465148, 465312, 465312, 465313, 465352, 465356, 465372], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-03-27"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-04-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ITA", "FRA", "LUX", "NLD", "BHR", "VNM", "USA", "CHN", "IND", "OMN", "MEX", "KWT", "RUS", "GBR", "HRV", "AUS", "MCO", "PAK", "ARE", "QAT", "GRC", "JPN", "AUT", "FIN", "ARM", "AZE", "ECU", "DEU", "NPL", "KOR", "DOM", "DZA", "SMR", "THA", "ISR", "EGY", "SWE", null, "MYS", "ISL", "IRQ", "IDN", "CZE", "SGP", "ROU", "DNK", "PHL", "NGA", "LKA", "IRN", "CAN", "CHE", "BRA", "LTU", "EST", "NZL", "AFG", "MKD", "BEL", "TWN", "KHM", "GEO", "IRL", "ESP", "LBN", "NOR", "BLR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ITA", "FRA", "LUX", "NLD", "BHR", "VNM", "USA", "CHN", "IND", "OMN", "MEX", "KWT", "RUS", "GBR", "HRV", "AUS", "MCO", "PAK", "ARE", "QAT", "GRC", "JPN", "AUT", "FIN", "ARM", "AZE", "ECU", "DEU", "NPL", "KOR", "DOM", "DZA", "SMR", "THA", "ISR", "EGY", "SWE", null, "MYS", "ISL", "IRQ", "IDN", "CZE", "SGP", "ROU", "DNK", "PHL", "NGA", "LKA", "IRN", "CAN", "CHE", "BRA", "LTU", "EST", "NZL", "AFG", "MKD", "BEL", "TWN", "KHM", "GEO", "IRL", "ESP", "LBN", "NOR", "BLR"], "marker": {"color": "#636efa", "size": [465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-04-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-04-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MCO", "SGP", "CZE", "MKD", "RUS", "KWT", "EGY", "GEO", "PAK", "CAN", "KOR", "GBR", "FIN", "GRC", "IND", "AUS", "EST", "PHL", "MYS", "LTU", "HRV", "DNK", "AUT", "IDN", "KHM", "SWE", "CHE", "AFG", "ARE", "ISL", "DZA", "OMN", "FRA", "IRL", "ITA", "BHR", "ROU", "NZL", "BEL", "NLD", "CHN", "ESP", "ISR", "LBN", "THA", "BLR", "NOR", "IRN", "TWN", "DOM", "BRA", "AZE", "NPL", "JPN", "DEU", "NGA", "VNM", "USA", "ARM", "SMR", "QAT", null, "IRQ", "LKA", "MEX", "ECU", "LUX"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MCO", "SGP", "CZE", "MKD", "RUS", "KWT", "EGY", "GEO", "PAK", "CAN", "KOR", "GBR", "FIN", "GRC", "IND", "AUS", "EST", "PHL", "MYS", "LTU", "HRV", "DNK", "AUT", "IDN", "KHM", "SWE", "CHE", "AFG", "ARE", "ISL", "DZA", "OMN", "FRA", "IRL", "ITA", "BHR", "ROU", "NZL", "BEL", "NLD", "CHN", "ESP", "ISR", "LBN", "THA", "BLR", "NOR", "IRN", "TWN", "DOM", "BRA", "AZE", "NPL", "JPN", "DEU", "NGA", "VNM", "USA", "ARM", "SMR", "QAT", null, "IRQ", "LKA", "MEX", "ECU", "LUX"], "marker": {"color": "#636efa", "size": [465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465372, 465373, 465373, 465373, 465373, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465374, 465375, 465375, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468612, 468614, 468614, 468615, 468615, 468615, 468615, 468615, 468615, 468615, 468615, 468615, 468615, 468615], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-04-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-04-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["USA", "DZA", "ARG", "BLR", "ROU", "PRT", "BEL", "IRL", "POL", "IRQ", "IRN", "CHE", "EST", "DNK", "SGP", "SWE", "CAN", "CZE", "NOR", "IND", "DEU", "ISL", "CHL", "CHN", "ESP", "PAK", "HRV", "KOR", "NZL", "QAT", "TWN", "RUS", "GBR", "JPN", "AUT", "SMR", "FRA", "BHR", "FIN", "ARE", "AUS", "NLD", "ITA", "MYS", "UKR"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["USA", "DZA", "ARG", "BLR", "ROU", "PRT", "BEL", "IRL", "POL", "IRQ", "IRN", "CHE", "EST", "DNK", "SGP", "SWE", "CAN", "CZE", "NOR", "IND", "DEU", "ISL", "CHL", "CHN", "ESP", "PAK", "HRV", "KOR", "NZL", "QAT", "TWN", "RUS", "GBR", "JPN", "AUT", "SMR", "FRA", "BHR", "FIN", "ARE", "AUS", "NLD", "ITA", "MYS", "UKR"], "marker": {"color": "#636efa", "size": [468637, 468639, 468640, 468643, 468644, 468646, 468651, 468652, 468653, 468658, 469493, 469500, 469501, 469504, 469506, 469515, 469518, 469518, 469526, 469527, 469566, 469576, 469577, 469696, 469733, 469734, 469735, 470251, 470252, 470257, 470258, 470259, 470270, 470284, 470290, 470292, 470326, 470328, 470329, 470335, 470343, 470353, 471020, 471027, 471028], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-04-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-05-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": [null, "AUT", "ARE", "AFG", "SGP", "VNM", "AUS", "CHN", "KOR", "GBR", "HRV", "ESP", "AZE", "THA", "LKA", "BHR", "BLR", "USA", "TWN", "BEL", "DZA", "SMR", "CAN", "EGY", "BRA", "DOM", "DNK", "SWE", "KHM", "ECU", "ARM", "CHE", "CZE", "RUS", "MEX", "FRA", "EST", "IRN", "NOR", "LTU", "MCO", "IRQ", "PHL", "OMN", "IRL", "ISL", "GRC", "MYS", "DEU", "NPL", "NLD", "LUX", "ISR", "QAT", "ITA", "FIN", "GEO", "PAK", "KWT", "NZL", "IDN", "MKD", "JPN", "NGA", "IND", "LBN", "ROU"], "legendgroup": "", "locationmode": "ISO-3", "locations": [null, "AUT", "ARE", "AFG", "SGP", "VNM", "AUS", "CHN", "KOR", "GBR", "HRV", "ESP", "AZE", "THA", "LKA", "BHR", "BLR", "USA", "TWN", "BEL", "DZA", "SMR", "CAN", "EGY", "BRA", "DOM", "DNK", "SWE", "KHM", "ECU", "ARM", "CHE", "CZE", "RUS", "MEX", "FRA", "EST", "IRN", "NOR", "LTU", "MCO", "IRQ", "PHL", "OMN", "IRL", "ISL", "GRC", "MYS", "DEU", "NPL", "NLD", "LUX", "ISR", "QAT", "ITA", "FIN", "GEO", "PAK", "KWT", "NZL", "IDN", "MKD", "JPN", "NGA", "IND", "LBN", "ROU"], "marker": {"color": "#636efa", "size": [471028, 471028, 471028, 471028, 471028, 471028, 471028, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043, 471043], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-05-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-05-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["QAT", "MYS", "AUS", "MCO", "THA", "DOM", "LUX", "HRV", "DNK", "AFG", "DZA", "AUT", "USA", "BLR", "ISR", "FIN", "ARM", "KHM", "PHL", "ITA", "SGP", "CZE", "IDN", "SWE", "NLD", "MEX", "VNM", "GBR", "SMR", "DEU", "EGY", "MKD", "LTU", "GRC", "NZL", "NPL", "KWT", "FRA", "NGA", "AZE", "ROU", "CHE", "BEL", "ISL", "IRN", "ARE", "RUS", "BRA", "LBN", "LKA", null, "NOR", "JPN", "ECU", "GEO", "ESP", "KOR", "IRQ", "EST", "CHN", "PAK", "BHR", "CAN", "IND", "IRL", "TWN", "OMN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["QAT", "MYS", "AUS", "MCO", "THA", "DOM", "LUX", "HRV", "DNK", "AFG", "DZA", "AUT", "USA", "BLR", "ISR", "FIN", "ARM", "KHM", "PHL", "ITA", "SGP", "CZE", "IDN", "SWE", "NLD", "MEX", "VNM", "GBR", "SMR", "DEU", "EGY", "MKD", "LTU", "GRC", "NZL", "NPL", "KWT", "FRA", "NGA", "AZE", "ROU", "CHE", "BEL", "ISL", "IRN", "ARE", "RUS", "BRA", "LBN", "LKA", null, "NOR", "JPN", "ECU", "GEO", "ESP", "KOR", "IRQ", "EST", "CHN", "PAK", "BHR", "CAN", "IND", "IRL", "TWN", "OMN"], "marker": {"color": "#636efa", "size": [471043, 471045, 471046, 471046, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471052, 471058, 471058, 471058, 471058, 471058, 471058, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471059, 471069, 471069, 471074, 471074, 471074, 471074, 471076, 471076, 471076, 474948, 474948, 474948, 474949, 474949, 474949, 474950, 474950], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-05-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-05-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["FRA", "DNK", "GEO", "NLD", "SEN", "PRT", "JPN", "BHR", "THA", "ITA", "SWE", "LIE", "DZA", "CAN", "CHE", "IRN", "NOR", "IND", "BRA", "ISL", "IRQ", "CHL", "SGP", "HUN", "CHN", "ESP", "IRL", "BEL", "KOR", "NZL", "GRC", "BLR", "SVN", "ISR", "DEU", "CZE", "OMN", "ECU", "MYS", "AUT", "GBR", "AUS", "SMR", "USA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["FRA", "DNK", "GEO", "NLD", "SEN", "PRT", "JPN", "BHR", "THA", "ITA", "SWE", "LIE", "DZA", "CAN", "CHE", "IRN", "NOR", "IND", "BRA", "ISL", "IRQ", "CHL", "SGP", "HUN", "CHN", "ESP", "IRL", "BEL", "KOR", "NZL", "GRC", "BLR", "SVN", "ISR", "DEU", "CZE", "OMN", "ECU", "MYS", "AUT", "GBR", "AUS", "SMR", "USA"], "marker": {"color": "#636efa", "size": [475023, 475025, 475025, 475035, 475037, 475038, 475087, 475090, 475094, 475681, 475692, 475693, 475700, 475703, 475723, 476309, 476332, 476354, 476355, 476365, 476370, 476372, 476374, 476376, 476493, 476542, 476546, 476556, 476994, 476995, 476998, 477000, 477001, 477006, 477072, 477075, 477083, 477086, 477100, 477105, 477139, 477150, 477155, 477189], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-05-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-06-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["OMN", "LTU", "FIN", "MEX", "ESP", "FRA", "ITA", "KWT", null, "ECU", "NOR", "PAK", "CHE", "BLR", "HRV", "NPL", "IRN", "AFG", "MYS", "BEL", "KOR", "DNK", "PHL", "IRL", "CHN", "NZL", "VNM", "RUS", "BHR", "IRQ", "EGY", "USA", "MCO", "GBR", "AZE", "GRC", "DZA", "ISL", "ROU", "GEO", "DOM", "NLD", "LUX", "SMR", "MKD", "IDN", "AUS", "CZE", "SWE", "ISR", "IND", "SGP", "QAT", "ARM", "KHM", "LBN", "ARE", "TWN", "CAN", "AUT", "THA", "JPN", "DEU", "NGA", "EST", "LKA", "BRA"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["OMN", "LTU", "FIN", "MEX", "ESP", "FRA", "ITA", "KWT", null, "ECU", "NOR", "PAK", "CHE", "BLR", "HRV", "NPL", "IRN", "AFG", "MYS", "BEL", "KOR", "DNK", "PHL", "IRL", "CHN", "NZL", "VNM", "RUS", "BHR", "IRQ", "EGY", "USA", "MCO", "GBR", "AZE", "GRC", "DZA", "ISL", "ROU", "GEO", "DOM", "NLD", "LUX", "SMR", "MKD", "IDN", "AUS", "CZE", "SWE", "ISR", "IND", "SGP", "QAT", "ARM", "KHM", "LBN", "ARE", "TWN", "CAN", "AUT", "THA", "JPN", "DEU", "NGA", "EST", "LKA", "BRA"], "marker": {"color": "#636efa", "size": [477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189, 477189], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-06-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-06-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GEO", "ARM", "PAK", "SGP", "NLD", "BLR", "AUS", "AFG", "ITA", "KOR", "CZE", "HRV", "BHR", "LBN", "LUX", "DEU", "ISR", "MYS", "FIN", "GRC", "USA", "EGY", "PHL", "DNK", "THA", "VNM", "GBR", "NZL", "BEL", "DZA", "MEX", "IRL", "NOR", "FRA", "BRA", "RUS", "MCO", "LTU", null, "CHE", "ECU", "DOM", "IRN", "CAN", "IND", "EST", "QAT", "SMR", "MKD", "IDN", "KHM", "AUT", "SWE", "ISL", "AZE", "LKA", "IRQ", "OMN", "TWN", "CHN", "NPL", "ESP", "ROU", "KWT", "JPN", "NGA", "ARE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GEO", "ARM", "PAK", "SGP", "NLD", "BLR", "AUS", "AFG", "ITA", "KOR", "CZE", "HRV", "BHR", "LBN", "LUX", "DEU", "ISR", "MYS", "FIN", "GRC", "USA", "EGY", "PHL", "DNK", "THA", "VNM", "GBR", "NZL", "BEL", "DZA", "MEX", "IRL", "NOR", "FRA", "BRA", "RUS", "MCO", "LTU", null, "CHE", "ECU", "DOM", "IRN", "CAN", "IND", "EST", "QAT", "SMR", "MKD", "IDN", "KHM", "AUT", "SWE", "ISL", "AZE", "LKA", "IRQ", "OMN", "TWN", "CHN", "NPL", "ESP", "ROU", "KWT", "JPN", "NGA", "ARE"], "marker": {"color": "#636efa", "size": [477189, 477189, 477189, 477193, 477193, 477193, 477194, 477194, 477194, 477199, 477199, 477199, 477199, 477199, 477199, 477199, 477199, 477201, 477201, 477201, 477202, 477202, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477203, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 477213, 480940, 480940, 480940, 480940, 480940, 480940, 480940, 480940], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-06-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-06-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["BIH", "CAN", "BTN", "EST", "DZA", "SWE", "DEU", "MYS", "ESP", "ROU", "ITA", "FIN", "CZE", "IRL", "KWT", "CHN", "GRC", "ZAF", "IRQ", "KOR", "AUS", "PSE", "USA", "HRV", "ISR", "SVN", "CHE", "CHL", "IND", "TWN", "NOR", "OMN", "NZL", "IRN", "EGY", "BRA", "ISL", "ECU", "BEL", "GBR", "MAR", "LBN", "SGP", "AZE", "FRA", "BHR", "SAU", "ARG", "SMR", "NLD", "ARE", "DNK", "PRT", "JPN", "GEO", "AUT", "SEN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["BIH", "CAN", "BTN", "EST", "DZA", "SWE", "DEU", "MYS", "ESP", "ROU", "ITA", "FIN", "CZE", "IRL", "KWT", "CHN", "GRC", "ZAF", "IRQ", "KOR", "AUS", "PSE", "USA", "HRV", "ISR", "SVN", "CHE", "CHL", "IND", "TWN", "NOR", "OMN", "NZL", "IRN", "EGY", "BRA", "ISL", "ECU", "BEL", "GBR", "MAR", "LBN", "SGP", "AZE", "FRA", "BHR", "SAU", "ARG", "SMR", "NLD", "ARE", "DNK", "PRT", "JPN", "GEO", "AUT", "SEN"], "marker": {"color": "#636efa", "size": [480942, 480954, 480955, 480958, 480963, 480989, 481127, 481127, 481188, 481190, 481959, 481964, 481968, 481975, 481977, 482147, 482169, 482170, 482177, 482695, 482702, 482709, 482783, 482784, 482786, 482791, 482821, 482822, 482823, 482825, 482855, 482856, 482857, 483448, 483449, 483454, 483463, 483466, 483493, 483523, 483524, 483527, 483532, 483535, 483673, 483673, 483677, 483678, 483685, 483729, 483731, 483741, 483745, 483777, 483783, 483795, 483796], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-06-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-07-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MKD", "ITA", "RUS", "JPN", "THA", "FIN", "TWN", "AUT", "IND", "AUS", "DEU", "KWT", "LBN", "IRL", "GRC", "DZA", "ECU", "CAN", "NPL", "PAK", "GBR", "LUX", "SMR", "ROU", "BLR", "LKA", "BRA", "HRV", "NGA", "ARE", "CHE", null, "DOM", "PHL", "BEL", "ISL", "EST", "SGP", "FRA", "AFG", "ISR", "SWE", "ESP", "CHN", "IRQ", "EGY", "GEO", "KHM", "USA", "VNM", "BHR", "QAT", "NZL", "MYS", "NLD", "KOR", "ARM", "MCO", "OMN", "DNK", "IRN", "CZE", "NOR", "IDN", "MEX", "LTU", "AZE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MKD", "ITA", "RUS", "JPN", "THA", "FIN", "TWN", "AUT", "IND", "AUS", "DEU", "KWT", "LBN", "IRL", "GRC", "DZA", "ECU", "CAN", "NPL", "PAK", "GBR", "LUX", "SMR", "ROU", "BLR", "LKA", "BRA", "HRV", "NGA", "ARE", "CHE", null, "DOM", "PHL", "BEL", "ISL", "EST", "SGP", "FRA", "AFG", "ISR", "SWE", "ESP", "CHN", "IRQ", "EGY", "GEO", "KHM", "USA", "VNM", "BHR", "QAT", "NZL", "MYS", "NLD", "KOR", "ARM", "MCO", "OMN", "DNK", "IRN", "CZE", "NOR", "IDN", "MEX", "LTU", "AZE"], "marker": {"color": "#636efa", "size": [483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796, 483796], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-07-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-07-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LUX", "KWT", "IRQ", "LTU", "NPL", null, "CHN", "MCO", "ESP", "MYS", "AZE", "LKA", "ISL", "IRL", "OMN", "NOR", "ROU", "DZA", "PAK", "FRA", "AFG", "NGA", "JPN", "USA", "KOR", "BRA", "ARE", "DNK", "BEL", "TWN", "RUS", "GRC", "NZL", "CHE", "DOM", "AUS", "NLD", "IDN", "QAT", "GEO", "BLR", "CAN", "FIN", "SMR", "BHR", "ARM", "IND", "GBR", "EST", "MKD", "MEX", "SGP", "PHL", "HRV", "CZE", "VNM", "DEU", "ITA", "THA", "KHM", "EGY", "ECU", "AUT", "IRN", "ISR", "SWE", "LBN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LUX", "KWT", "IRQ", "LTU", "NPL", null, "CHN", "MCO", "ESP", "MYS", "AZE", "LKA", "ISL", "IRL", "OMN", "NOR", "ROU", "DZA", "PAK", "FRA", "AFG", "NGA", "JPN", "USA", "KOR", "BRA", "ARE", "DNK", "BEL", "TWN", "RUS", "GRC", "NZL", "CHE", "DOM", "AUS", "NLD", "IDN", "QAT", "GEO", "BLR", "CAN", "FIN", "SMR", "BHR", "ARM", "IND", "GBR", "EST", "MKD", "MEX", "SGP", "PHL", "HRV", "CZE", "VNM", "DEU", "ITA", "THA", "KHM", "EGY", "ECU", "AUT", "IRN", "ISR", "SWE", "LBN"], "marker": {"color": "#636efa", "size": [483796, 483796, 483796, 483796, 483796, 483837, 486997, 486997, 486997, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 486999, 487000, 487000, 487000, 487000, 487000, 487005, 487005, 487005, 487005, 487005, 487005, 487006, 487006, 487006, 487006, 487006, 487006, 487008, 487008, 487008, 487008, 487008, 487008, 487009, 487009, 487009, 487009, 487011, 487011, 487011, 487011, 487013, 487014, 487014, 487014, 487014, 487014, 487014, 487014, 487014, 487014, 487014, 487014], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-07-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-07-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["EST", "IRL", "SWE", "SMR", "GBR", "EGY", "VAT", "AUS", "ISL", "CMR", "FRA", "RUS", "ARG", "FIN", "TWN", "HUN", "CHN", "POL", "CZE", "CHL", "QAT", "LUX", "ITA", "IDN", "CAN", "TGO", "ESP", "DEU", "PHL", "SVK", "MYS", "USA", "ROU", "NZL", "ISR", "BHR", "IRN", "DOM", "SGP", "GRC", "THA", "AZE", "AUT", "LBN", "NOR", "NLD", "HRV", "KOR", "BEL", "DNK", "IND", "PSE", "CRI", "PRT", "SRB", "SVN", "PAK", "CHE", "VNM", "COL", "JPN", "PER", "BRA", "MKD"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["EST", "IRL", "SWE", "SMR", "GBR", "EGY", "VAT", "AUS", "ISL", "CMR", "FRA", "RUS", "ARG", "FIN", "TWN", "HUN", "CHN", "POL", "CZE", "CHL", "QAT", "LUX", "ITA", "IDN", "CAN", "TGO", "ESP", "DEU", "PHL", "SVK", "MYS", "USA", "ROU", "NZL", "ISR", "BHR", "IRN", "DOM", "SGP", "GRC", "THA", "AZE", "AUT", "LBN", "NOR", "NLD", "HRV", "KOR", "BEL", "DNK", "IND", "PSE", "CRI", "PRT", "SRB", "SVN", "PAK", "CHE", "VNM", "COL", "JPN", "PER", "BRA", "MKD"], "marker": {"color": "#636efa", "size": [487019, 487024, 487100, 487101, 487149, 487161, 487162, 487166, 487174, 487175, 487365, 487371, 487377, 487384, 487385, 487387, 487488, 487492, 487499, 487500, 487503, 487505, 488283, 488285, 488291, 488292, 488405, 488689, 488691, 488692, 488725, 488830, 488831, 488832, 488834, 488838, 490072, 490073, 490086, 490099, 490102, 490105, 490138, 490144, 490171, 490217, 490218, 490701, 490760, 490763, 490765, 490774, 490775, 490779, 490780, 490783, 490784, 490906, 490907, 490908, 490967, 490968, 490973, 490975], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-07-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-08-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": [null, "AZE", "ISL", "VNM", "LKA", "IRN", "JPN", "ARE", "SMR", "KWT", "KHM", "CHE", "CAN", "LUX", "AUT", "MEX", "NGA", "LTU", "IND", "EST", "NOR", "IDN", "LBN", "ROU", "ESP", "SWE", "FRA", "DOM", "BRA", "MKD", "BHR", "MYS", "RUS", "BLR", "ARM", "ISR", "HRV", "NZL", "NLD", "GEO", "DZA", "ITA", "FIN", "AFG", "OMN", "DEU", "DNK", "THA", "KOR", "TWN", "BEL", "NPL", "IRL", "AUS", "CHN", "ECU", "PAK", "MCO", "IRQ", "EGY", "QAT", "GRC", "GBR", "PHL", "SGP", "USA", "CZE"], "legendgroup": "", "locationmode": "ISO-3", "locations": [null, "AZE", "ISL", "VNM", "LKA", "IRN", "JPN", "ARE", "SMR", "KWT", "KHM", "CHE", "CAN", "LUX", "AUT", "MEX", "NGA", "LTU", "IND", "EST", "NOR", "IDN", "LBN", "ROU", "ESP", "SWE", "FRA", "DOM", "BRA", "MKD", "BHR", "MYS", "RUS", "BLR", "ARM", "ISR", "HRV", "NZL", "NLD", "GEO", "DZA", "ITA", "FIN", "AFG", "OMN", "DEU", "DNK", "THA", "KOR", "TWN", "BEL", "NPL", "IRL", "AUS", "CHN", "ECU", "PAK", "MCO", "IRQ", "EGY", "QAT", "GRC", "GBR", "PHL", "SGP", "USA", "CZE"], "marker": {"color": "#636efa", "size": [490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975, 490975], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-08-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-08-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["NZL", null, "AUS", "GEO", "LUX", "LTU", "THA", "MCO", "IRN", "DOM", "BLR", "HRV", "EGY", "IND", "ESP", "ISR", "BHR", "CZE", "EST", "ITA", "SWE", "AUT", "KHM", "NLD", "SGP", "IDN", "ARM", "CAN", "MEX", "SMR", "GBR", "LBN", "PHL", "ECU", "DEU", "QAT", "VNM", "KWT", "FIN", "MKD", "GRC", "CHE", "TWN", "JPN", "NOR", "PAK", "ARE", "AFG", "RUS", "ROU", "FRA", "AZE", "USA", "KOR", "BRA", "CHN", "DZA", "NGA", "OMN", "IRQ", "NPL", "DNK", "IRL", "MYS", "LKA", "BEL", "ISL"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["NZL", null, "AUS", "GEO", "LUX", "LTU", "THA", "MCO", "IRN", "DOM", "BLR", "HRV", "EGY", "IND", "ESP", "ISR", "BHR", "CZE", "EST", "ITA", "SWE", "AUT", "KHM", "NLD", "SGP", "IDN", "ARM", "CAN", "MEX", "SMR", "GBR", "LBN", "PHL", "ECU", "DEU", "QAT", "VNM", "KWT", "FIN", "MKD", "GRC", "CHE", "TWN", "JPN", "NOR", "PAK", "ARE", "AFG", "RUS", "ROU", "FRA", "AZE", "USA", "KOR", "BRA", "CHN", "DZA", "NGA", "OMN", "IRQ", "NPL", "DNK", "IRL", "MYS", "LKA", "BEL", "ISL"], "marker": {"color": "#636efa", "size": [490975, 490978, 490978, 490978, 490978, 490978, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490985, 490988, 490988, 490988, 490988, 490988, 490988, 490988, 490988, 490988, 490988, 490989, 490989, 490990, 490990, 490990, 490990, 490990, 490990, 490991, 490991, 490991, 490991, 490993, 490993, 490993, 490993, 490998, 490998, 490998, 490998, 490998, 494416, 494416, 494416, 494416, 494416, 494416, 494416, 494416, 494417, 494417, 494417, 494417], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-08-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-08-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["GBR", "AFG", "SVK", "LVA", "VNM", "CAN", "AUS", "ARE", "SWE", "ISL", "MDV", "SAU", "DEU", "HUN", "ARG", "CHN", "MLT", "TWN", "POL", "CZE", "QAT", "KWT", "IRL", "KHM", "MDA", "ITA", "IRQ", "ESP", "PHL", "BGR", "IRN", "SVN", "SGP", "GRC", "AUT", "JPN", "ZAF", "NLD", "DNK", "BRA", "PRT", "ISR", "IND", "BEL", "KOR", "GEO", "HRV", "ROU", "BHR", "JOR", "NOR", "USA", "FRA", "PRY", "MYS", "SMR", "CHE", "PSE"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["GBR", "AFG", "SVK", "LVA", "VNM", "CAN", "AUS", "ARE", "SWE", "ISL", "MDV", "SAU", "DEU", "HUN", "ARG", "CHN", "MLT", "TWN", "POL", "CZE", "QAT", "KWT", "IRL", "KHM", "MDA", "ITA", "IRQ", "ESP", "PHL", "BGR", "IRN", "SVN", "SGP", "GRC", "AUT", "JPN", "ZAF", "NLD", "DNK", "BRA", "PRT", "ISR", "IND", "BEL", "KOR", "GEO", "HRV", "ROU", "BHR", "JOR", "NOR", "USA", "FRA", "PRY", "MYS", "SMR", "CHE", "PSE"], "marker": {"color": "#636efa", "size": [494460, 494463, 494465, 494466, 494470, 494476, 494487, 494503, 494527, 494537, 494539, 494541, 494704, 494707, 494708, 494754, 494757, 494757, 494758, 494765, 494766, 494769, 494770, 494771, 494772, 496019, 496035, 496091, 496092, 496094, 497170, 497173, 497181, 497202, 497227, 497274, 497275, 497335, 497343, 497343, 497351, 497357, 497360, 497420, 497787, 497790, 497791, 497797, 497804, 497804, 497838, 497933, 498036, 498037, 498047, 498050, 498105, 498108], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-08-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-09-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["BRA", "ARM", "IRN", "IRL", "KWT", "MCO", "NPL", "CHE", "ARE", "TWN", "BHR", "IRQ", "LBN", "NGA", "ITA", "MEX", "ISR", "NLD", "AUS", "BEL", "NZL", "THA", "GBR", "SWE", "DZA", "BLR", "AZE", "AUT", "USA", "IDN", "JPN", "LKA", "GEO", "IND", "ESP", "HRV", "QAT", "FIN", "DEU", "ECU", "LTU", "AFG", "CAN", "DOM", "CZE", "VNM", "LUX", "SMR", "ISL", "MYS", "SGP", "FRA", null, "DNK", "RUS", "OMN", "CHN", "PHL", "GRC", "ROU", "PAK", "EST", "NOR", "MKD", "KOR", "KHM", "EGY"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["BRA", "ARM", "IRN", "IRL", "KWT", "MCO", "NPL", "CHE", "ARE", "TWN", "BHR", "IRQ", "LBN", "NGA", "ITA", "MEX", "ISR", "NLD", "AUS", "BEL", "NZL", "THA", "GBR", "SWE", "DZA", "BLR", "AZE", "AUT", "USA", "IDN", "JPN", "LKA", "GEO", "IND", "ESP", "HRV", "QAT", "FIN", "DEU", "ECU", "LTU", "AFG", "CAN", "DOM", "CZE", "VNM", "LUX", "SMR", "ISL", "MYS", "SGP", "FRA", null, "DNK", "RUS", "OMN", "CHN", "PHL", "GRC", "ROU", "PAK", "EST", "NOR", "MKD", "KOR", "KHM", "EGY"], "marker": {"color": "#636efa", "size": [498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-09-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-09-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["AFG", "ARM", "DNK", "SMR", "QAT", "BEL", "PAK", "LTU", "USA", "JPN", "DOM", "IDN", "CHE", "CZE", "MCO", "SWE", "KHM", null, "AZE", "LKA", "FRA", "KOR", "NGA", "ARE", "ESP", "NLD", "NPL", "BRA", "IRQ", "ITA", "LUX", "NZL", "RUS", "FIN", "PHL", "OMN", "MEX", "BHR", "KWT", "ROU", "GRC", "AUS", "HRV", "THA", "IRL", "MYS", "LBN", "EGY", "CHN", "DZA", "SGP", "AUT", "MKD", "ECU", "IRN", "ISR", "VNM", "TWN", "GBR", "IND", "CAN", "EST", "ISL", "GEO", "BLR", "NOR", "DEU"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["AFG", "ARM", "DNK", "SMR", "QAT", "BEL", "PAK", "LTU", "USA", "JPN", "DOM", "IDN", "CHE", "CZE", "MCO", "SWE", "KHM", null, "AZE", "LKA", "FRA", "KOR", "NGA", "ARE", "ESP", "NLD", "NPL", "BRA", "IRQ", "ITA", "LUX", "NZL", "RUS", "FIN", "PHL", "OMN", "MEX", "BHR", "KWT", "ROU", "GRC", "AUS", "HRV", "THA", "IRL", "MYS", "LBN", "EGY", "CHN", "DZA", "SGP", "AUT", "MKD", "ECU", "IRN", "ISR", "VNM", "TWN", "GBR", "IND", "CAN", "EST", "ISL", "GEO", "BLR", "NOR", "DEU"], "marker": {"color": "#636efa", "size": [498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498108, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498109, 498110, 498110, 498110, 500717, 500717, 500724, 500724, 500724, 500724, 500724, 500724, 500725, 500726, 500727, 500727, 500727, 500727, 500727, 500727, 500727, 500727, 500727], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-09-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-09-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["TWN", "CHL", "IRQ", "PER", "ARG", "CRI", "ALB", "KOR", "BEL", "ISR", "VNM", "ZAF", "GRC", "MDV", "SVN", "ESP", "SVK", "PHL", "LUX", "HUN", "CHN", "DEU", "ITA", "BGD", "CZE", "IRL", "BRA", "SGP", "NOR", "GBR", "SMR", "AUT", "MEX", "CHE", "BGR", "EGY", "IRN", "ECU", "CAN", "AUS", "SWE", "DZA", "FIN", "CMR", "IDN", "MYS", "LVA", "USA", "ROU", "KWT", "FRA", "JPN", "SAU", "PRT", "BHR", "GEO", "LBN", "NLD", "POL", "DNK", "ISL", "QAT"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["TWN", "CHL", "IRQ", "PER", "ARG", "CRI", "ALB", "KOR", "BEL", "ISR", "VNM", "ZAF", "GRC", "MDV", "SVN", "ESP", "SVK", "PHL", "LUX", "HUN", "CHN", "DEU", "ITA", "BGD", "CZE", "IRL", "BRA", "SGP", "NOR", "GBR", "SMR", "AUT", "MEX", "CHE", "BGR", "EGY", "IRN", "ECU", "CAN", "AUS", "SWE", "DZA", "FIN", "CMR", "IDN", "MYS", "LVA", "USA", "ROU", "KWT", "FRA", "JPN", "SAU", "PRT", "BHR", "GEO", "LBN", "NLD", "POL", "DNK", "ISL", "QAT"], "marker": {"color": "#636efa", "size": [500727, 500732, 500739, 500745, 500748, 500752, 500754, 501002, 501033, 501047, 501056, 501057, 501064, 501066, 501070, 501229, 501231, 501235, 501237, 501238, 501283, 501338, 502830, 502833, 502839, 502841, 502853, 502865, 502887, 502954, 502965, 502968, 502970, 503038, 503040, 503074, 503817, 503818, 503823, 503829, 503871, 503874, 503885, 503886, 503888, 503894, 503895, 504016, 504018, 504021, 504431, 504464, 504468, 504477, 504493, 504494, 504504, 504581, 504586, 504593, 504595, 504598], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-09-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-10-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["RUS", "NPL", "DZA", "CZE", "GBR", "PHL", "SMR", "BHR", "KWT", "ROU", "PAK", "GRC", "ITA", "EST", "AFG", "ARM", "EGY", "HRV", "BLR", "VNM", "ISR", "ARE", "DOM", "AUT", "NLD", "FRA", "SGP", "AZE", "AUS", "DEU", "GEO", "MYS", "THA", "FIN", "QAT", "MCO", "LUX", "DNK", "JPN", "ECU", "BEL", "KHM", "ISL", "ESP", "KOR", null, "LTU", "NOR", "IDN", "IND", "CHN", "OMN", "USA", "MEX", "LKA", "CHE", "NZL", "NGA", "SWE", "IRN", "IRQ", "MKD", "IRL", "BRA", "LBN", "TWN", "CAN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["RUS", "NPL", "DZA", "CZE", "GBR", "PHL", "SMR", "BHR", "KWT", "ROU", "PAK", "GRC", "ITA", "EST", "AFG", "ARM", "EGY", "HRV", "BLR", "VNM", "ISR", "ARE", "DOM", "AUT", "NLD", "FRA", "SGP", "AZE", "AUS", "DEU", "GEO", "MYS", "THA", "FIN", "QAT", "MCO", "LUX", "DNK", "JPN", "ECU", "BEL", "KHM", "ISL", "ESP", "KOR", null, "LTU", "NOR", "IDN", "IND", "CHN", "OMN", "USA", "MEX", "LKA", "CHE", "NZL", "NGA", "SWE", "IRN", "IRQ", "MKD", "IRL", "BRA", "LBN", "TWN", "CAN"], "marker": {"color": "#636efa", "size": [504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-10-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-10-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["ISL", "GEO", "SMR", "RUS", "KWT", "QAT", "ROU", "DNK", "LTU", "MEX", "ITA", "BLR", "IDN", "NLD", "LBN", "MKD", "TWN", "VNM", "AUT", "ECU", "DEU", "SGP", "PHL", "DZA", "IRN", "CHN", "BHR", "IRQ", "ESP", "ISR", "ARM", "CZE", "FIN", "NGA", null, "MYS", "CHE", "MCO", "BRA", "AFG", "EGY", "USA", "NPL", "ARE", "GBR", "BEL", "LKA", "SWE", "EST", "KOR", "AZE", "FRA", "LUX", "NZL", "CAN", "DOM", "IRL", "JPN", "THA", "GRC", "KHM", "AUS", "HRV", "NOR", "IND", "OMN", "PAK"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["ISL", "GEO", "SMR", "RUS", "KWT", "QAT", "ROU", "DNK", "LTU", "MEX", "ITA", "BLR", "IDN", "NLD", "LBN", "MKD", "TWN", "VNM", "AUT", "ECU", "DEU", "SGP", "PHL", "DZA", "IRN", "CHN", "BHR", "IRQ", "ESP", "ISR", "ARM", "CZE", "FIN", "NGA", null, "MYS", "CHE", "MCO", "BRA", "AFG", "EGY", "USA", "NPL", "ARE", "GBR", "BEL", "LKA", "SWE", "EST", "KOR", "AZE", "FRA", "LUX", "NZL", "CAN", "DOM", "IRL", "JPN", "THA", "GRC", "KHM", "AUS", "HRV", "NOR", "IND", "OMN", "PAK"], "marker": {"color": "#636efa", "size": [504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504598, 504601, 504601, 504601, 504601, 507575, 507575, 507575, 507576, 507576, 507576, 507576, 507576, 507576, 507582, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507584, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586, 507586], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-10-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-10-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": [null, "CHL", "DNK", "BHR", "LBN", "JPN", "FIN", "IRN", "DOM", "ROU", "IND", "POL", "ARE", "TUN", "SWE", "KWT", "GEO", "QAT", "CAN", "NLD", "PRT", "MNG", "ISL", "SGP", "SAU", "USA", "OMN", "ZAF", "LVA", "PHL", "CHE", "SMR", "CYP", "MYS", "HUN", "PSE", "PAN", "PER", "KOR", "BRN", "COL", "NOR", "BEL", "GBR", "GRC", "ALB", "VNM", "MLT", "FRA", "CZE", "RUS", "CHN", "EGY", "CRI", "NGA", "PAK", "ECU", "ITA", "AUT", "AUS", "DEU", "ESP"], "legendgroup": "", "locationmode": "ISO-3", "locations": [null, "CHL", "DNK", "BHR", "LBN", "JPN", "FIN", "IRN", "DOM", "ROU", "IND", "POL", "ARE", "TUN", "SWE", "KWT", "GEO", "QAT", "CAN", "NLD", "PRT", "MNG", "ISL", "SGP", "SAU", "USA", "OMN", "ZAF", "LVA", "PHL", "CHE", "SMR", "CYP", "MYS", "HUN", "PSE", "PAN", "PER", "KOR", "BRN", "COL", "NOR", "BEL", "GBR", "GRC", "ALB", "VNM", "MLT", "FRA", "CZE", "RUS", "CHN", "EGY", "CRI", "NGA", "PAK", "ECU", "ITA", "AUT", "AUS", "DEU", "ESP"], "marker": {"color": "#636efa", "size": [507577, 507580, 507655, 507685, 507694, 507720, 507730, 508325, 508328, 508330, 508340, 508346, 508360, 508361, 508406, 508407, 508409, 508412, 508427, 508483, 508492, 508493, 508503, 508513, 508517, 508717, 508719, 508723, 508726, 508749, 508791, 508803, 508805, 508823, 508824, 508825, 508826, 508828, 508959, 508960, 508962, 508985, 509024, 509072, 509083, 509087, 509088, 509089, 509375, 509383, 509383, 509403, 509409, 509413, 509414, 509424, 509425, 511222, 511251, 511271, 511508, 512123], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-10-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-11-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["DOM", "LKA", "DZA", "EST", "MEX", "FIN", "OMN", "LTU", "MYS", "USA", "BEL", "AUS", "JPN", "IND", "FRA", "BRA", "GRC", "VNM", "TWN", "MCO", "SMR", "PAK", "KOR", "DNK", "QAT", null, "IRL", "ARE", "AZE", "PHL", "HRV", "NOR", "LUX", "DEU", "SWE", "GEO", "KWT", "NLD", "GBR", "ESP", "ITA", "ISR", "IDN", "BHR", "IRQ", "CZE", "AUT", "MKD", "SGP", "CAN", "CHN", "KHM", "ARM", "ROU", "CHE", "BLR", "EGY", "NZL", "NPL", "ISL", "RUS", "IRN", "NGA", "ECU", "AFG", "THA", "LBN"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["DOM", "LKA", "DZA", "EST", "MEX", "FIN", "OMN", "LTU", "MYS", "USA", "BEL", "AUS", "JPN", "IND", "FRA", "BRA", "GRC", "VNM", "TWN", "MCO", "SMR", "PAK", "KOR", "DNK", "QAT", null, "IRL", "ARE", "AZE", "PHL", "HRV", "NOR", "LUX", "DEU", "SWE", "GEO", "KWT", "NLD", "GBR", "ESP", "ITA", "ISR", "IDN", "BHR", "IRQ", "CZE", "AUT", "MKD", "SGP", "CAN", "CHN", "KHM", "ARM", "ROU", "CHE", "BLR", "EGY", "NZL", "NPL", "ISL", "RUS", "IRN", "NGA", "ECU", "AFG", "THA", "LBN"], "marker": {"color": "#636efa", "size": [512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123, 512123], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-11-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-11-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["SMR", "CAN", "LTU", "GBR", null, "CHE", "IRN", "IND", "FRA", "FIN", "AUT", "QAT", "ECU", "ARM", "PAK", "AZE", "DOM", "ESP", "ROU", "DEU", "CHN", "ISR", "TWN", "VNM", "MKD", "RUS", "IDN", "BLR", "KHM", "OMN", "MCO", "GRC", "IRL", "THA", "HRV", "NZL", "SWE", "EST", "BEL", "KOR", "LUX", "NLD", "ITA", "MYS", "PHL", "NPL", "AFG", "EGY", "ARE", "USA", "LKA", "BRA", "JPN", "CZE", "NOR", "MEX", "GEO", "AUS", "BHR", "LBN", "ISL", "DZA", "IRQ", "KWT", "DNK", "NGA", "SGP"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["SMR", "CAN", "LTU", "GBR", null, "CHE", "IRN", "IND", "FRA", "FIN", "AUT", "QAT", "ECU", "ARM", "PAK", "AZE", "DOM", "ESP", "ROU", "DEU", "CHN", "ISR", "TWN", "VNM", "MKD", "RUS", "IDN", "BLR", "KHM", "OMN", "MCO", "GRC", "IRL", "THA", "HRV", "NZL", "SWE", "EST", "BEL", "KOR", "LUX", "NLD", "ITA", "MYS", "PHL", "NPL", "AFG", "EGY", "ARE", "USA", "LKA", "BRA", "JPN", "CZE", "NOR", "MEX", "GEO", "AUS", "BHR", "LBN", "ISL", "DZA", "IRQ", "KWT", "DNK", "NGA", "SGP"], "marker": {"color": "#636efa", "size": [512123, 512123, 512123, 512127, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 512192, 514682, 514682, 514682, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514683, 514684, 514684, 514684, 514684, 514684, 514684, 514684, 514684, 514684, 514685, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514686, 514688], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-11-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-11-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["MKD", "LTU", "MDA", "IRN", "EST", "IDN", "BFA", "ROU", "EGY", "CAN", "MYS", "SWE", "LVA", "AUS", "BRA", "LBN", "OMN", "CHN", "ITA", "DEU", "ARG", "CZE", "LUX", "PHL", "IRL", "SVK", "PER", "HUN", "SVN", "PRY", "BLR", "PAN", "PSE", "ESP", "VNM", "GRC", "ISR", "KOR", "BEL", "HRV", "ALB", "THA", "MDV", "PAK", "CRI", "TWN", "CHL", "ISL", "COD", "CHE", "AUT", "AFG", "FRA", "SMR", "NOR", "GBR", "ECU", "KWT", "AZE", "IND", "TUN", "QAT", "SAU", "POL", "DNK", "SGP", "IRQ", "NLD", "JPN", "PRT", "BHR", "USA", "SRB", "ARE", "GEO"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["MKD", "LTU", "MDA", "IRN", "EST", "IDN", "BFA", "ROU", "EGY", "CAN", "MYS", "SWE", "LVA", "AUS", "BRA", "LBN", "OMN", "CHN", "ITA", "DEU", "ARG", "CZE", "LUX", "PHL", "IRL", "SVK", "PER", "HUN", "SVN", "PRY", "BLR", "PAN", "PSE", "ESP", "VNM", "GRC", "ISR", "KOR", "BEL", "HRV", "ALB", "THA", "MDV", "PAK", "CRI", "TWN", "CHL", "ISL", "COD", "CHE", "AUT", "AFG", "FRA", "SMR", "NOR", "GBR", "ECU", "KWT", "AZE", "IND", "TUN", "QAT", "SAU", "POL", "DNK", "SGP", "IRQ", "NLD", "JPN", "PRT", "BHR", "USA", "SRB", "ARE", "GEO"], "marker": {"color": "#636efa", "size": [514692, 514694, 514696, 515577, 515580, 515593, 515595, 515603, 515607, 515623, 515635, 515713, 515715, 515727, 515736, 515736, 515736, 515765, 516742, 516899, 516906, 516929, 516931, 516931, 516945, 516947, 516949, 516952, 516967, 516971, 516974, 516980, 516989, 517424, 517428, 517434, 517465, 517707, 517735, 517736, 517740, 517749, 517751, 517751, 517755, 517758, 517762, 517767, 517768, 517884, 517935, 517938, 518310, 518323, 518408, 518460, 518462, 518466, 518468, 518474, 518477, 518483, 518488, 518493, 518644, 518650, 518650, 518711, 518765, 518767, 518768, 519039, 519043, 519058, 519066], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-11-03"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-12-01<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["LBN", "VNM", "AFG", "USA", "MEX", "LTU", "MYS", "ARM", "LUX", "DZA", "SWE", "LKA", "IDN", "FRA", "NGA", "IRN", "CHE", "SMR", "DOM", "KHM", "IRQ", "IRL", "TWN", "BEL", "ECU", "BLR", "QAT", "THA", "NZL", "NLD", "MKD", "PHL", "HRV", "PAK", "GRC", "DEU", "KOR", "OMN", "CHN", "DNK", "SGP", "ESP", "NOR", "ISL", "GEO", null, "CAN", "IND", "CZE", "ISR", "BRA", "ARE", "NPL", "AUT", "AUS", "GBR", "EST", "RUS", "ROU", "AZE", "FIN", "JPN", "ITA", "BHR", "MCO", "KWT", "EGY"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["LBN", "VNM", "AFG", "USA", "MEX", "LTU", "MYS", "ARM", "LUX", "DZA", "SWE", "LKA", "IDN", "FRA", "NGA", "IRN", "CHE", "SMR", "DOM", "KHM", "IRQ", "IRL", "TWN", "BEL", "ECU", "BLR", "QAT", "THA", "NZL", "NLD", "MKD", "PHL", "HRV", "PAK", "GRC", "DEU", "KOR", "OMN", "CHN", "DNK", "SGP", "ESP", "NOR", "ISL", "GEO", null, "CAN", "IND", "CZE", "ISR", "BRA", "ARE", "NPL", "AUT", "AUS", "GBR", "EST", "RUS", "ROU", "AZE", "FIN", "JPN", "ITA", "BHR", "MCO", "KWT", "EGY"], "marker": {"color": "#636efa", "size": [519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066, 519066], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-12-01"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-12-02<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": [null, "IND", "AUT", "MYS", "LKA", "LTU", "CAN", "GEO", "ROU", "MEX", "ARM", "DNK", "NOR", "GBR", "RUS", "EST", "HRV", "MCO", "VNM", "KOR", "PAK", "LUX", "OMN", "DEU", "AUS", "CHN", "ESP", "PHL", "CZE", "IDN", "KWT", "ISL", "SGP", "SWE", "ITA", "KHM", "NGA", "QAT", "IRQ", "ARE", "SMR", "ECU", "JPN", "IRL", "BHR", "BEL", "NZL", "THA", "FIN", "BLR", "EGY", "ISR", "NLD", "USA", "LBN", "TWN", "BRA", "FRA", "MKD", "AFG", "DZA", "IRN", "NPL", "CHE", "GRC", "AZE", "DOM"], "legendgroup": "", "locationmode": "ISO-3", "locations": [null, "IND", "AUT", "MYS", "LKA", "LTU", "CAN", "GEO", "ROU", "MEX", "ARM", "DNK", "NOR", "GBR", "RUS", "EST", "HRV", "MCO", "VNM", "KOR", "PAK", "LUX", "OMN", "DEU", "AUS", "CHN", "ESP", "PHL", "CZE", "IDN", "KWT", "ISL", "SGP", "SWE", "ITA", "KHM", "NGA", "QAT", "IRQ", "ARE", "SMR", "ECU", "JPN", "IRL", "BHR", "BEL", "NZL", "THA", "FIN", "BLR", "EGY", "ISR", "NLD", "USA", "LBN", "TWN", "BRA", "FRA", "MKD", "AFG", "DZA", "IRN", "NPL", "CHE", "GRC", "AZE", "DOM"], "marker": {"color": "#636efa", "size": [519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519105, 519107, 519107, 521135, 521135, 521135, 521135, 521135, 521135, 521135, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521137, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138, 521138], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-12-02"}, {"data": [{"geo": "geo", "hoverlabel": {"namelength": 0}, "hovertemplate": "datesRepStr=2020-12-03<br>cases_sum=%{marker.size}<br>countryterritoryCode=%{location}", "ids": ["EGY", "PSE", "PAN", "SRB", "MYS", "GEO", "POL", "SVN", "PRT", "CUB", "QAT", "SAU", "EST", "FRA", "SVK", "SMR", "CYP", "PHL", "CZE", "ROU", "DEU", "FIN", "SGP", "DNK", "PER", "GRC", "MDA", "KOR", "BRN", "BRA", "IRQ", "BIH", "TWN", "BOL", "IRL", "THA", "BEL", "ISR", "BLR", "ITA", "TUN", "TUR", "NLD", "JAM", "JPN", "BHR", "AZE", "ARM", "MAR", "KWT", "GBR", "AUT", "LBN", "AUS", "LVA", "CHE", "BGR", "IRN", "BFA", "HRV", "ALB", "CIV", "CRI", "MDV", "PAK", "COL", "HND", "VNM", "HUN", "ESP", "LUX", "MLT", "ZAF", "CHN", "CHL", "LKA", "IND", "USA", "NOR", "MEX", "LTU", "CAN", "LIE", "SWE", "IDN", "KHM", "DZA", "ISL", "RUS"], "legendgroup": "", "locationmode": "ISO-3", "locations": ["EGY", "PSE", "PAN", "SRB", "MYS", "GEO", "POL", "SVN", "PRT", "CUB", "QAT", "SAU", "EST", "FRA", "SVK", "SMR", "CYP", "PHL", "CZE", "ROU", "DEU", "FIN", "SGP", "DNK", "PER", "GRC", "MDA", "KOR", "BRN", "BRA", "IRQ", "BIH", "TWN", "BOL", "IRL", "THA", "BEL", "ISR", "BLR", "ITA", "TUN", "TUR", "NLD", "JAM", "JPN", "BHR", "AZE", "ARM", "MAR", "KWT", "GBR", "AUT", "LBN", "AUS", "LVA", "CHE", "BGR", "IRN", "BFA", "HRV", "ALB", "CIV", "CRI", "MDV", "PAK", "COL", "HND", "VNM", "HUN", "ESP", "LUX", "MLT", "ZAF", "CHN", "CHL", "LKA", "IND", "USA", "NOR", "MEX", "LTU", "CAN", "LIE", "SWE", "IDN", "KHM", "DZA", "ISL", "RUS"], "marker": {"color": "#636efa", "size": [521139, 521140, 521147, 521160, 521180, 521181, 521190, 521216, 521234, 521237, 521475, 521500, 521503, 522000, 522003, 522007, 522011, 522027, 522058, 522078, 522349, 522368, 522380, 522632, 522638, 522647, 522648, 522762, 522772, 522790, 522799, 522800, 522800, 522802, 522810, 522821, 522868, 522880, 522883, 525196, 525198, 525199, 525320, 525322, 525373, 525425, 525427, 525430, 525433, 525436, 525519, 525583, 525603, 525617, 525619, 525771, 525774, 526732, 526732, 526735, 526736, 526737, 526746, 526748, 526752, 526758, 526760, 526764, 526765, 527266, 527266, 527268, 527274, 527298, 527304, 527305, 527328, 527615, 527827, 527831, 527831, 527841, 527843, 527979, 527994, 527995, 527995, 528010, 528025], "sizemode": "area", "sizeref": 1320.0625}, "name": "", "showlegend": false, "type": "scattergeo"}], "name": "2020-12-03"}]);
}).then(function(){
var gd = document.getElementById('d61a1113-b0b4-4576-91d6-5d6bef2b5a30'); var x = new MutationObserver(function (mutations, observer) {{ var display = window.getComputedStyle(gd).display; if (!display || display === 'none') {{ console.log([gd, 'removed!']); Plotly.purge(gd); observer.disconnect(); }} }});
// Listen for the removal of the full notebook cells var notebookContainer = gd.closest('#notebook-container'); if (notebookContainer) {{ x.observe(notebookContainer, {childList: true}); }}
// Listen for the clearing of the current output cell var outputEl = gd.closest('.output'); if (outputEl) {{ x.observe(outputEl, {childList: true}); }}
})
};
});
</script>
</div>