Css files not loading with actix
DogsCodesStudio opened this issue · comments
I have
use actix_files::Files;
use actix_web::{get, web, App, HttpResponse, HttpServer};
use handlebars::Handlebars;
use serde_json::json;
#[get("/")]
async fn tests(hb: web::Data<Handlebars<'_>>) -> HttpResponse {
let data = json!({
"user": 25,
"data": 12
});
let body = hb.render("404", &data).unwrap();
HttpResponse::Ok().body(body)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
let mut handlebars = Handlebars::new();
handlebars
.register_templates_directory(".html", "/var/www/templates")
.unwrap();
// let tests = Files::new("/static", ".").show_files_listing();
let handlebars_ref = web::Data::new(handlebars);
HttpServer::new(move || App::new()
.service(Files::new("/var/www/templates", ".").show_files_listing())
.app_data(handlebars_ref.clone())
.service(tests))
.bind(("127.0.0.1", 25565))?
.run()
.await
}
async fn not_found(hb: web::Data<Handlebars<'_>>) -> HttpResponse {
let data = json!({
"user": 25,
"data": 12
});
let body = hb.render("404", &data).unwrap();
HttpResponse::Ok().body(body)
}``` and
[dependencies]
actix-web = "4"
actix-files = "0.6.2"
handlebars = { version = "4.2.1", features = ["dir_source"] }
serde = { version = "1.0.143", features = ["derive"] }
serde_json = "1.0.72"
with my template file being
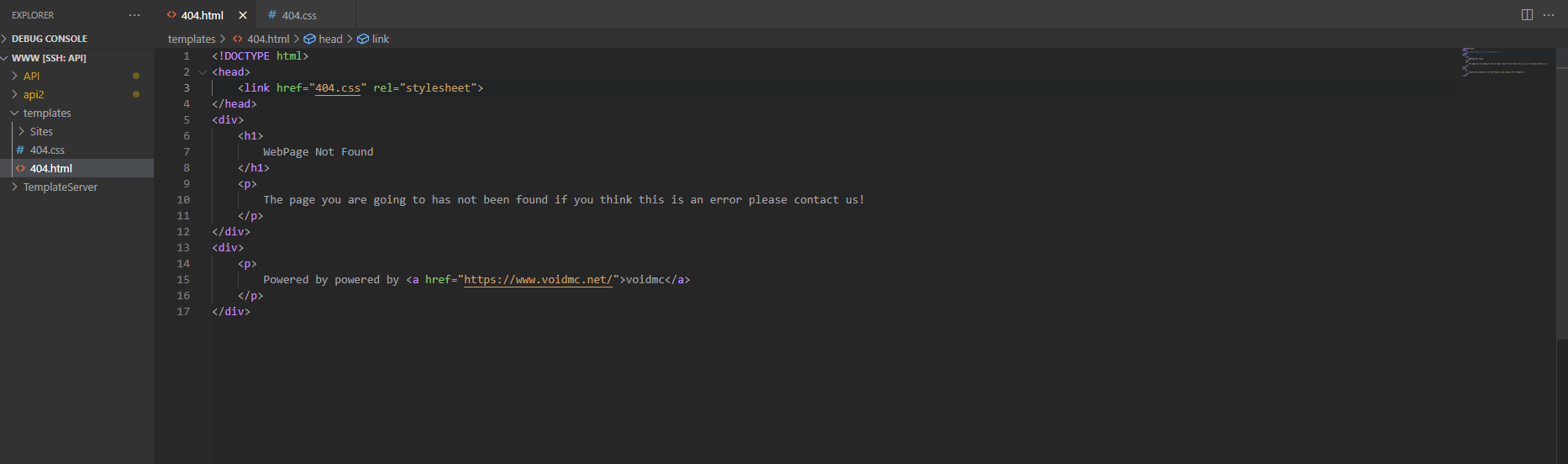
but it doesn't seem to be loading the css file
Typically template engine is not responsible for css loading. Could you please check your generated content to see if it has correct css file path?
You are using the file system absolute path for the css file. Just remove the path /var/www/templates/
from html and see if it works.